Tricolor LED driver for any 3 pin tricolor LED.
Tricolor Class Reference
#include <tricolor.h>
Public Member Functions | |
Tricolor (PinName r, PinName g, PinName b) | |
Basic Constructor. | |
Tricolor (PinName r, uint8_t r_color, PinName g, uint8_t g_color, PinName b, uint8_t b_color) | |
Color Constructor Adds color values to constructor. | |
void | Blue (void) |
Sets LED to blue (LED will be on afterwards) | |
void | Green (void) |
Sets LED to green (LED will be on afterwards) | |
void | LEDOff (void) |
Turns the LED off (saves color) | |
void | LEDOn (void) |
Restores LED to set color (color MUST be set beforehand) | |
void | Red (void) |
Sets LED to red (LED will be on afterwards) | |
void | SetLEDColor (uint8_t, uint8_t, uint8_t) |
Sets custom color for LED (LED will be on afterwards) | |
void | Toggle (void) |
Toggles the LED on and off. |
Detailed Description
Tricolor LED driver.
This is a reentrant LED controller
#include "mbed.h" #include "tricolor.h" Tricolor led(p23, p24, p25); // red, green, blue int main() { led.SetLEDColor(0, 100, 0); // Green @ about 40% while(1) { wait(0.5); led.Toggle(); } }
Definition at line 26 of file tricolor.h.
Constructor & Destructor Documentation
Tricolor | ( | PinName | r, |
PinName | g, | ||
PinName | b | ||
) |
Basic Constructor.
Definition at line 31 of file tricolor.h.
Tricolor | ( | PinName | r, |
uint8_t | r_color, | ||
PinName | g, | ||
uint8_t | g_color, | ||
PinName | b, | ||
uint8_t | b_color | ||
) |
Color Constructor Adds color values to constructor.
Definition at line 37 of file tricolor.h.
Member Function Documentation
void Blue | ( | void | ) |
Sets LED to blue (LED will be on afterwards)
Definition at line 9 of file tricolor.cpp.
void Green | ( | void | ) |
Sets LED to green (LED will be on afterwards)
Definition at line 13 of file tricolor.cpp.
void LEDOff | ( | void | ) |
Turns the LED off (saves color)
Definition at line 17 of file tricolor.cpp.
void LEDOn | ( | void | ) |
Restores LED to set color (color MUST be set beforehand)
Definition at line 25 of file tricolor.cpp.
void Red | ( | void | ) |
Sets LED to red (LED will be on afterwards)
Definition at line 32 of file tricolor.cpp.
void SetLEDColor | ( | uint8_t | red, |
uint8_t | green, | ||
uint8_t | blue | ||
) |
Sets custom color for LED (LED will be on afterwards)
- Parameters:
-
red Amount of red color (0-255) green Amount of green color (0-255) blue Amount of blue color (0-255)
Definition at line 36 of file tricolor.cpp.
void Toggle | ( | void | ) |
Toggles the LED on and off.
Definition at line 44 of file tricolor.cpp.
Generated on Tue Jul 12 2022 16:54:56 by
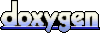