
add drawing circles at every touch points.
Dependencies: BSP_DISCO_F746NG LCD_DISCO_F746NG TS_DISCO_F746NG mbed
Fork of DISCO-F746NG_LCDTS_demo by
main.cpp
00001 #include "mbed.h" 00002 #include "TS_DISCO_F746NG.h" 00003 #include "LCD_DISCO_F746NG.h" 00004 00005 LCD_DISCO_F746NG lcd; 00006 TS_DISCO_F746NG ts; 00007 00008 int main() 00009 { 00010 TS_StateTypeDef TS_State; 00011 uint16_t x, y; 00012 uint8_t text[30]; 00013 uint8_t status; 00014 uint8_t idx; 00015 uint8_t cleared = 0; 00016 uint8_t prev_nb_touches = 0; 00017 00018 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN DEMO", CENTER_MODE); 00019 wait(1); 00020 00021 status = ts.Init(lcd.GetXSize(), lcd.GetYSize()); 00022 if (status != TS_OK) { 00023 lcd.Clear(LCD_COLOR_RED); 00024 lcd.SetBackColor(LCD_COLOR_RED); 00025 lcd.SetTextColor(LCD_COLOR_WHITE); 00026 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT FAIL", CENTER_MODE); 00027 } else { 00028 lcd.Clear(LCD_COLOR_GREEN); 00029 lcd.SetBackColor(LCD_COLOR_GREEN); 00030 lcd.SetTextColor(LCD_COLOR_WHITE); 00031 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT OK", CENTER_MODE); 00032 } 00033 00034 wait(1); 00035 lcd.SetFont(&Font12); 00036 lcd.SetBackColor(LCD_COLOR_BLUE); 00037 lcd.SetTextColor(LCD_COLOR_WHITE); 00038 00039 while(1) { 00040 00041 ts.GetState(&TS_State); 00042 if (TS_State.touchDetected) { 00043 // Clear lines corresponding to old touches coordinates 00044 if (TS_State.touchDetected < prev_nb_touches) { 00045 for (idx = (TS_State.touchDetected + 1); idx <= 5; idx++) { 00046 lcd.ClearStringLine(idx); 00047 } 00048 } 00049 prev_nb_touches = TS_State.touchDetected; 00050 00051 cleared = 0; 00052 00053 sprintf((char*)text, "Touches: %d", TS_State.touchDetected); 00054 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); 00055 00056 for (idx = 0; idx < TS_State.touchDetected; idx++) { 00057 x = TS_State.touchX[idx]; 00058 y = TS_State.touchY[idx]; 00059 sprintf((char*)text, "Touch %d: x=%d y=%d ", idx+1, x, y); 00060 lcd.SetTextColor(LCD_COLOR_WHITE); 00061 lcd.DisplayStringAt(0, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00062 lcd.SetTextColor(LCD_COLOR_YELLOW); 00063 lcd.DrawCircle(x, y, 20); 00064 } 00065 00066 } else { 00067 if (!cleared) { 00068 lcd.Clear(LCD_COLOR_BLUE); 00069 sprintf((char*)text, "Touches: 0"); 00070 lcd.SetTextColor(LCD_COLOR_WHITE); 00071 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); 00072 cleared = 1; 00073 } 00074 } 00075 } 00076 }
Generated on Sun Jul 24 2022 23:08:00 by
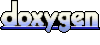