
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
ConfigFile Class Reference
Simple config file object Only supports reading config files. More...
#include <ConfigFile.h>
Public Member Functions | |
ConfigFile (char *name) | |
Make new ConfigFile object. | |
bool | Value (char *key, char *value, size_t maxlen, char *def) |
Read value. | |
bool | Value (char *key, int *value, int def) |
Read Integer value. | |
bool | IsOpen (void) |
See if file was present. |
Detailed Description
Simple config file object Only supports reading config files.
Tries to limit memory usage. Note: the file handle is kept open during the lifetime of this object. To close the file: destroy this ConfigFile object! A simple way is to enclose the creation of this object inside a code block Example:
char ip[16]; int port; { ConfigFile cfg("/local/config.txt"); cfg.Value("ip", ip, sizeof(ip), "192.168.1.10"); cfg.Value("port", &port, 80); }
Definition at line 63 of file ConfigFile.h.
Constructor & Destructor Documentation
ConfigFile | ( | char * | name ) |
Make new ConfigFile object.
Open config file. Note: the file handle is kept open during the lifetime of this object. To close the file: destroy this ConfigFile object!
- Parameters:
-
file Filename of the configuration file.
Definition at line 26 of file ConfigFile.cpp.
Member Function Documentation
bool IsOpen | ( | void | ) |
See if file was present.
- Returns:
- "true" if file is open, "false" file is not found
Definition at line 94 of file ConfigFile.h.
bool Value | ( | char * | key, |
char * | value, | ||
size_t | maxlen, | ||
char * | def | ||
) |
Read value.
If file is not open, or key does not exist: copy default value (return false)
- Parameters:
-
key name of the key in the file value pointer to buffer that receives the value maxlen the maximum length of the value. If the actual value is longer, it is truncated def Default value. If the key is not found in the file, this value is copied.
- Returns:
- "true" if the key is found "false" is key is not found (default value is returned)
Definition at line 50 of file ConfigFile.cpp.
bool Value | ( | char * | key, |
int * | value, | ||
int | def | ||
) |
Read Integer value.
If file is not open, or key does not exist: copy default value (return false)
- Parameters:
-
key name of the key in the file value pointer to integer that receives the value def Default value. If the key is not found in the file, this value is copied.
- Returns:
- "true" if the key is found "false" is key is not found (default value is returned)
Definition at line 132 of file ConfigFile.cpp.
Generated on Tue Jul 12 2022 21:03:04 by
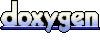