
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
ConfigFile.h
00001 /** 00002 * ConfigFile.cpp 00003 * Simple Config file reader class 00004 * 00005 * Copyright (c) 2011 Peter Brier 00006 * 00007 * This file is part of the LaOS project (see: http://wiki.protospace.nl/index.php/LaOS) 00008 * 00009 * LaOS is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * LaOS is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00021 * 00022 * Reads a setting, based on the key. (case sensitive) 00023 * If the key is not found, the default value is returned. 00024 * 00025 * Simple, not (time) efficient. For every request, the complete file is reset and read again 00026 * 00027 @code 00028 file format 00029 00030 ; comment 00031 key value [newline || comment] 00032 00033 For example: 00034 00035 ; This is a test config file 00036 ip 192.168.1.1 00037 port 1234 ; the key is "port" the value is "1234". The rest is comment 00038 ; EOF 00039 @endcode 00040 */ 00041 #ifndef _CONFIG_FILE_H_ 00042 #define _CONFIG_FILE_H_ 00043 00044 #include "global.h" 00045 #include "laosfilesystem.h" 00046 00047 /** Simple config file object 00048 * Only supports reading config files. Tries to limit memory usage. 00049 * Note: the file handle is kept open during the lifetime of this object. 00050 * To close the file: destroy this ConfigFile object! A simple way is to enclose the creation 00051 * of this object inside a code block 00052 * Example: 00053 * @code 00054 * char ip[16]; 00055 * int port; 00056 * { 00057 * ConfigFile cfg("/local/config.txt"); 00058 * cfg.Value("ip", ip, sizeof(ip), "192.168.1.10"); 00059 * cfg.Value("port", &port, 80); 00060 * } 00061 * @endcode 00062 */ 00063 class ConfigFile { 00064 public: 00065 /** Make new ConfigFile object. Open config file. 00066 * Note: the file handle is kept open during the lifetime of this object. 00067 * To close the file: destroy this ConfigFile object! 00068 * @param file Filename of the configuration file. 00069 */ 00070 ConfigFile(char *name); 00071 00072 ~ConfigFile(); 00073 00074 /** Read value. If file is not open, or key does not exist: copy default value (return false) 00075 * @param key name of the key in the file 00076 * @param value pointer to buffer that receives the value 00077 * @param maxlen the maximum length of the value. If the actual value is longer, it is truncated 00078 * @param def Default value. If the key is not found in the file, this value is copied. 00079 * @return "true" if the key is found "false" is key is not found (default value is returned) 00080 */ 00081 bool Value(char *key, char *value, size_t maxlen, char *def); 00082 00083 /** Read Integer value. If file is not open, or key does not exist: copy default value (return false) 00084 * @param key name of the key in the file 00085 * @param value pointer to integer that receives the value 00086 * @param def Default value. If the key is not found in the file, this value is copied. 00087 * @return "true" if the key is found "false" is key is not found (default value is returned) 00088 */ 00089 bool Value(char *key, int *value, int def); 00090 00091 /** See if file was present 00092 * @return "true" if file is open, "false" file is not found 00093 */ 00094 bool IsOpen(void) { return fp != NULL; } 00095 00096 private: 00097 FILE *fp; 00098 00099 }; 00100 00101 00102 #endif
Generated on Tue Jul 12 2022 21:03:02 by
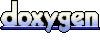