modify the write function for temperature update If you use update temperature, you modify the repeat condition when write function use - read16() -> m_I2C.write(m_Addr, ®, 1); //stop condition - read16() -> m_I2C.write(m_Addr, ®, 1,1); //repeat condition
Fork of LM75B by
LM75B.cpp
00001 /* LM75B Driver Library 00002 * Copyright (c) 2013 Neil Thiessen 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "LM75B.h" 00018 00019 LM75B::LM75B(PinName sda, PinName scl, Address addr, int hz) : m_I2C(sda, scl) 00020 { 00021 //Set the internal device address 00022 m_Addr = (int)addr; 00023 m_I2C.frequency(hz); 00024 } 00025 00026 00027 bool LM75B::open(void) 00028 { 00029 //Probe for the LM75B using a Zero Length Transfer 00030 if (!m_I2C.write(m_Addr, NULL, 0)) { 00031 //Reset the device to default configuration 00032 write8(REG_CONF, 0x00); 00033 write16(REG_THYST, 0x4B00); 00034 write16(REG_TOS, 0x5000); 00035 00036 //Return success 00037 return true; 00038 } else { 00039 //Return failure 00040 return false; 00041 } 00042 } 00043 00044 LM75B::PowerMode LM75B::powerMode(void) 00045 { 00046 //Read the 8-bit register value 00047 char value = read8(REG_CONF); 00048 00049 //Return the status of the SHUTDOWN bit 00050 if (value & (1 << 0)) 00051 return POWER_SHUTDOWN; 00052 else 00053 return POWER_NORMAL; 00054 } 00055 00056 void LM75B::powerMode(PowerMode mode) 00057 { 00058 //Read the current 8-bit register value 00059 char value = read8(REG_CONF); 00060 00061 //Set or clear the SHUTDOWN bit 00062 if (mode == POWER_SHUTDOWN) 00063 value |= (1 << 0); 00064 else 00065 value &= ~(1 << 0); 00066 00067 //Write the value back out 00068 write8(REG_CONF, value); 00069 } 00070 00071 LM75B::OSMode LM75B::osMode(void) 00072 { 00073 //Read the 8-bit register value 00074 char value = read8(REG_CONF); 00075 00076 //Return the status of the OS_COMP_INT bit 00077 if (value & (1 << 1)) 00078 return OS_INTERRUPT; 00079 else 00080 return OS_COMPARATOR; 00081 } 00082 00083 void LM75B::osMode(OSMode mode) 00084 { 00085 //Read the current 8-bit register value 00086 char value = read8(REG_CONF); 00087 00088 //Set or clear the OS_COMP_INT bit 00089 if (mode == OS_INTERRUPT) 00090 value |= (1 << 1); 00091 else 00092 value &= ~(1 << 1); 00093 00094 //Write the value back out 00095 write8(REG_CONF, value); 00096 } 00097 00098 LM75B::OSPolarity LM75B::osPolarity(void) 00099 { 00100 //Read the 8-bit register value 00101 char value = read8(REG_CONF); 00102 00103 //Return the status of the OS_POL bit 00104 if (value & (1 << 2)) 00105 return OS_ACTIVE_HIGH; 00106 else 00107 return OS_ACTIVE_LOW; 00108 } 00109 00110 void LM75B::osPolarity(OSPolarity polarity) 00111 { 00112 //Read the current 8-bit register value 00113 char value = read8(REG_CONF); 00114 00115 //Set or clear the OS_POL bit 00116 if (polarity == OS_ACTIVE_HIGH) 00117 value |= (1 << 2); 00118 else 00119 value &= ~(1 << 2); 00120 00121 //Write the value back out 00122 write8(REG_CONF, value); 00123 } 00124 00125 LM75B::OSFaultQueue LM75B::osFaultQueue(void) 00126 { 00127 //Read the 8-bit register value 00128 char value = read8(REG_CONF); 00129 00130 //Return the status of the OS_F_QUE bits 00131 if ((value & (1 << 3)) && (value & (1 << 4))) 00132 return OS_FAULT_QUEUE_6; 00133 else if (!(value & (1 << 3)) && (value & (1 << 4))) 00134 return OS_FAULT_QUEUE_4; 00135 else if ((value & (1 << 3)) && !(value & (1 << 4))) 00136 return OS_FAULT_QUEUE_2; 00137 else 00138 return OS_FAULT_QUEUE_1; 00139 } 00140 00141 void LM75B::osFaultQueue(OSFaultQueue queue) 00142 { 00143 //Read the current 8-bit register value 00144 char value = read8(REG_CONF); 00145 00146 //Clear the old OS_F_QUE bits 00147 value &= ~(3 << 3); 00148 00149 //Set the new OS_F_QUE bits 00150 if (queue == OS_FAULT_QUEUE_2) 00151 value |= (1 << 3); 00152 else if (queue == OS_FAULT_QUEUE_4) 00153 value |= (2 << 3); 00154 else if (queue == OS_FAULT_QUEUE_6) 00155 value |= (3 << 3); 00156 00157 //Write the value back out 00158 write8(REG_CONF, value); 00159 } 00160 00161 float LM75B::alertTemp(void) 00162 { 00163 //Use the 9-bit helper to read the TOS register 00164 return readAlertTempHelper(REG_TOS); 00165 } 00166 00167 void LM75B::alertTemp(float temp) 00168 { 00169 //Use the 9-bit helper to write to the TOS register 00170 return writeAlertTempHelper(REG_TOS, temp); 00171 } 00172 00173 float LM75B::alertHyst(void) 00174 { 00175 //Use the 9-bit helper to read the THYST register 00176 return readAlertTempHelper(REG_THYST); 00177 } 00178 00179 void LM75B::alertHyst(float temp) 00180 { 00181 //Use the 9-bit helper to write to the THYST register 00182 return writeAlertTempHelper(REG_THYST, temp); 00183 } 00184 00185 float LM75B::temp(void) 00186 { 00187 //Signed return value 00188 short value; 00189 00190 //Read the 11-bit raw temperature value 00191 value = read16(REG_TEMP) >> 5; 00192 //Sign extend negative numbers 00193 if (value & (1 << 10)) 00194 value |= 0xFC00; 00195 00196 //Return the temperature in °C 00197 return value*0.125; 00198 } 00199 00200 char LM75B::read8(char reg) 00201 { 00202 //Select the register 00203 m_I2C.write(m_Addr, ®, 1); 00204 00205 //Read the 8-bit register 00206 m_I2C.read(m_Addr, ®, 1); 00207 00208 //Return the byte 00209 return reg; 00210 } 00211 00212 void LM75B::write8(char reg, char data) 00213 { 00214 //Create a temporary buffer 00215 char buff[2]; 00216 00217 //Load the register address and 8-bit data 00218 buff[0] = reg; 00219 buff[1] = data; 00220 00221 //Write the data 00222 m_I2C.write(m_Addr, buff, 2); 00223 } 00224 00225 unsigned short LM75B::read16(char reg) 00226 { 00227 //Create a temporary buffer 00228 char buff[2]; 00229 00230 //Select the register 00231 m_I2C.write(m_Addr, ®, 1,1); 00232 00233 //Read the 16-bit register 00234 m_I2C.read(m_Addr, buff, 2); 00235 00236 //Return the combined 16-bit value 00237 return (buff[0] << 8) | buff[1]; 00238 } 00239 00240 void LM75B::write16(char reg, unsigned short data) 00241 { 00242 //Create a temporary buffer 00243 char buff[3]; 00244 00245 //Load the register address and 16-bit data 00246 buff[0] = reg; 00247 buff[1] = data >> 8; 00248 buff[2] = data; 00249 00250 //Write the data 00251 m_I2C.write(m_Addr, buff, 3); 00252 } 00253 00254 float LM75B::readAlertTempHelper(char reg) 00255 { 00256 //Signed return value 00257 short value; 00258 00259 //Read the 9-bit raw temperature value 00260 value = read16(reg) >> 7; 00261 00262 //Sign extend negative numbers 00263 if (value & (1 << 8)) 00264 value |= 0xFF00; 00265 00266 //Return the temperature in °C 00267 return value * 0.5; 00268 } 00269 00270 void LM75B::writeAlertTempHelper(char reg, float temp) 00271 { 00272 //Range limit temp 00273 if (temp < -55.0) 00274 temp = -55.0; 00275 else if (temp > 125.0) 00276 temp = 125.0; 00277 00278 //Extract and shift the signed integer 00279 short value = temp * 2; 00280 value <<= 7; 00281 00282 //Send the new value 00283 write16(reg, value); 00284 }
Generated on Tue Jul 12 2022 19:35:25 by
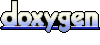