
Example FIR filter using the draft mbed DSP API
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "dsp.h" 00003 00004 #define BLOCK_SIZE (32) 00005 #define NUM_BLOCKS (10) 00006 #define TEST_LENGTH_SAMPLES (BLOCK_SIZE * NUM_BLOCKS) 00007 00008 #define SAMPLE_RATE (48000) 00009 00010 #define SNR_THRESHOLD_F32 (50.0f) 00011 00012 float32_t expected_output[TEST_LENGTH_SAMPLES]; 00013 float32_t output[TEST_LENGTH_SAMPLES]; 00014 00015 /* FIR Coefficients buffer generated using fir1() MATLAB function: fir1(28, 6/24) */ 00016 #define NUM_TAPS 29 00017 const float32_t firCoeffs32[NUM_TAPS] = { 00018 -0.0018225230f, -0.0015879294f, +0.0000000000f, +0.0036977508f, +0.0080754303f, 00019 +0.0085302217f, -0.0000000000f, -0.0173976984f, -0.0341458607f, -0.0333591565f, 00020 +0.0000000000f, +0.0676308395f, +0.1522061835f, +0.2229246956f, +0.2504960933f, 00021 +0.2229246956f, +0.1522061835f, +0.0676308395f, +0.0000000000f, -0.0333591565f, 00022 -0.0341458607f, -0.0173976984f, -0.0000000000f, +0.0085302217f, +0.0080754303f, 00023 +0.0036977508f, +0.0000000000f, -0.0015879294f, -0.0018225230f 00024 }; 00025 #define WARMUP (NUM_TAPS-1) 00026 #define DELAY (WARMUP/2) 00027 00028 int main() { 00029 Sine_f32 sine_1KHz( 1000, SAMPLE_RATE, 1.0); 00030 Sine_f32 sine_15KHz(15000, SAMPLE_RATE, 0.5); 00031 FIR_f32<NUM_TAPS> fir(firCoeffs32); 00032 00033 float32_t buffer_a[BLOCK_SIZE]; 00034 float32_t buffer_b[BLOCK_SIZE]; 00035 for (float32_t *sgn=output; sgn<(output+TEST_LENGTH_SAMPLES); sgn += BLOCK_SIZE) { 00036 sine_1KHz.generate(buffer_a); // Generate a 1KHz sine wave 00037 sine_15KHz.process(buffer_a, buffer_b); // Add a 15KHz sine wave 00038 fir.process(buffer_b, sgn); // FIR low pass filter: 6KHz cutoff 00039 } 00040 00041 sine_1KHz.reset(); 00042 for (float32_t *sgn=expected_output; sgn<(expected_output+TEST_LENGTH_SAMPLES); sgn += BLOCK_SIZE) { 00043 sine_1KHz.generate(sgn); // Generate a 1KHz sine wave 00044 } 00045 00046 float snr = arm_snr_f32(&expected_output[DELAY-1], &output[WARMUP-1], TEST_LENGTH_SAMPLES-WARMUP); 00047 printf("snr: %f\n\r", snr); 00048 if (snr < SNR_THRESHOLD_F32) { 00049 printf("Failed\n\r"); 00050 } else { 00051 printf("Success\n\r"); 00052 } 00053 }
Generated on Tue Jul 12 2022 13:32:58 by
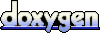