
CooCox 1.1.4 on mbed with simple blinky example
task management implementation code of CooCox CoOS kernel. More...
Go to the source code of this file.
Functions | |
void | CreateTCBList (void) |
Create a TCB list. | |
static BOOL | GetPriSeqNum (U8 pri, OS_TID *SequenceNum) |
Get sequence number for Assign priority. | |
static U8 | GetRdyPriSeqNum (U8 seqNum) |
Get the nearest ready priority sequence number for Assign number. | |
static void | PrioRemap (OS_TID seqNum) |
Remap the ready status of priority queue from Assign sequence number. | |
static BOOL | GetPrioSeqNumStatus (U8 seqNum) |
Get the ready status for assign sequence number. | |
static void | SetPrioSeqNumStatus (U8 seqNum, BOOL isRdy) |
Set the ready status for assign sequence number. | |
void | ActiveTaskPri (U8 pri) |
Active priority in queue. | |
void | DeleteTaskPri (U8 pri) |
Delete priority in queue. | |
void | InsertToTCBRdyList (P_OSTCB tcbInsert) |
Insert a task to the ready list. | |
void | RemoveFromTCBRdyList (P_OSTCB ptcb) |
Remove a task from the READY list. | |
StatusType | CoSetPriority (OS_TID taskID, U8 priority) |
Change task priority. | |
void | Schedule (void) |
Schedule function. | |
static P_OSTCB | AssignTCB (void) |
Assign a TCB to task being created. | |
OS_TID | CreateTask (FUNCPtr task, void *argv, U32 parameter, OS_STK *stk) |
Create a task. | |
StatusType | CoDelTask (OS_TID taskID) |
Delete Task. | |
void | CoExitTask (void) |
Exit Task. | |
StatusType | CoActivateTask (OS_TID taskID, void *argv) |
Activate Task. | |
OS_TID | CoGetCurTaskID (void) |
Get current task id. | |
StatusType | CoSuspendTask (OS_TID taskID) |
Suspend Task. | |
StatusType | CoAwakeTask (OS_TID taskID) |
Awake Task. | |
Variables | |
OSTCB | TCBTbl [CFG_MAX_USER_TASKS+SYS_TASK_NUM] = {{0}} |
P_OSTCB | FreeTCB = 0 |
P_OSTCB | TCBRdy = 0 |
P_OSTCB | TCBNext = 0 |
P_OSTCB | TCBRunning = 0 |
U64 | OSCheckTime = 0 |
Detailed Description
task management implementation code of CooCox CoOS kernel.
- Version:
- V1.1.4
- Date:
- 2011.04.20
INTERNAL FILE,DON'T PUBLIC.
© COPYRIGHT 2009 CooCox
Definition in file task.c.
Function Documentation
void ActiveTaskPri | ( | U8 | pri ) |
Active priority in queue.
- Parameters:
-
[in] pri Task priority [in] None [out] None
- Return values:
-
None
- Description
This function is called in Binary-Scheduling Algorithm to active priority in queue, if this priority had been in activation, increate the task num for this priority.
static P_OSTCB AssignTCB | ( | void | ) | [static] |
StatusType CoActivateTask | ( | OS_TID | taskID, |
void * | argv | ||
) |
StatusType CoAwakeTask | ( | OS_TID | taskID ) |
Awake Task.
- Parameters:
-
[in] taskID ID of task that will been awaked. [out] None
- Return values:
-
E_OK Task awake successful. E_INVALID_ID Invalid task ID. E_TASK_NOT_WAITING Task now not in waiting state. E_TASK_WAIT_OTHER Task now waiting other awake event. E_PROTECTED_TASK Idle task mustn't be awaked.
- Description
This function is called to awake current task.
StatusType CoDelTask | ( | OS_TID | taskID ) |
void CoExitTask | ( | void | ) |
OS_TID CoGetCurTaskID | ( | void | ) |
StatusType CoSetPriority | ( | OS_TID | taskID, |
U8 | priority | ||
) |
Change task priority.
- Parameters:
-
[in] taskID Specify task id. [in] priority New priority. [out] None
- Return values:
-
E_OK Change priority successful. E_INVALID_ID Invalid id,change priority fail. E_PROTECTED_TASK Can't change idle task priority.
- Description
This function is called to change priority for a specify task.
StatusType CoSuspendTask | ( | OS_TID | taskID ) |
Suspend Task.
- Parameters:
-
[in] taskID ID of task that want to suspend. [out] None
- Return values:
-
E_OK Task suspend successful. E_INVALID_ID Invalid event ID. E_PROTECTED_TASK Can't suspend idle task. E_ALREADY_IN_WAITING Task now in waiting state.
- Description
This function is called to exit current task.
OS_TID CreateTask | ( | FUNCPtr | task, |
void * | argv, | ||
U32 | parameter, | ||
OS_STK * | stk | ||
) |
Create a task.
- Parameters:
-
[in] task Task code entry. [in] argv The parameter passed to task. [in] parameter Task priority + stack size + time slice + isWaitting. [in] stk Pointer to stack top of task. [out] None
- Return values:
-
E_CREATE_FAIL Fail to create a task . others Valid task id.
- Description
This function is called by application to create a task,return a id to mark this task.
void CreateTCBList | ( | void | ) |
void DeleteTaskPri | ( | U8 | pri ) |
static BOOL GetPrioSeqNumStatus | ( | U8 | seqNum ) | [static] |
Get the ready status for assign sequence number.
- Parameters:
-
[in] seqNum Assign sequence number [out] None
- Return values:
-
Co_TRUE This priority has ready task Co_FALSE This priority doesn't have ready task
- Description
This function is called in Binary-Scheduling Algorithm to get the ready status for assign sequence number.
static BOOL GetPriSeqNum | ( | U8 | pri, |
OS_TID * | SequenceNum | ||
) | [static] |
Get sequence number for Assign priority.
- Parameters:
-
[in] pri Assign priority [out] SequenceNum priority number
- Return values:
-
Co_TRUE Assign priority in priority queue. Co_FALSE Assign priority not in priority queue.
- Description
This function is called in Binary-Scheduling Algorithm to get sequence number for Assign priority.
static U8 GetRdyPriSeqNum | ( | U8 | seqNum ) | [static] |
Get the nearest ready priority sequence number for Assign number.
- Parameters:
-
[in] seqNum Assign sequence number [out] None
- Return values:
-
INVALID_ID Cannot find higher ready priority. Others Nearest ready priority sequence number
- Description
This function is called in Binary-Scheduling Algorithm to get the nearest ready priority sequence number.
void InsertToTCBRdyList | ( | P_OSTCB | tcbInsert ) |
static void PrioRemap | ( | OS_TID | seqNum ) | [static] |
void RemoveFromTCBRdyList | ( | P_OSTCB | ptcb ) |
void Schedule | ( | void | ) |
static void SetPrioSeqNumStatus | ( | U8 | seqNum, |
BOOL | isRdy | ||
) | [static] |
Set the ready status for assign sequence number.
- Parameters:
-
[in] seqNum Assign sequence number [in] isRdy Ready statues for assign sequence number [out] None
- Return values:
-
None
- Description
This function is called in Binary-Scheduling Algorithm to set the ready status for assign sequence number.
Variable Documentation
U64 OSCheckTime = 0 |
P_OSTCB TCBRunning = 0 |
Generated on Tue Jul 12 2022 18:19:10 by
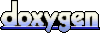