
Demo program for the Eyes_uLCD-144-G2 micro LCD
Dependencies: 4DGL-uLCD-SE Eyes_uLCD-144-G2 mbed
main.cpp
00001 /* 00002 Eyes Class produces an LCD Object with Eyes that can look around, blink, 00003 convey expressions, etc. 00004 00005 TODO: Replace "wait()" with Thread::wait() so that this isn't eating 00006 up processor time!. Maybe could use a Ticker or Timer interrupt 00007 but we don't want to call Eye methods until wait is complete... 00008 so that probably isn't a good idea... Thread::wait() is probably 00009 best for an end application. 00010 00011 OR add close_eyes to gesture() method and leave it to user to 00012 create blink gestures and other gestures that require waits... 00013 this can be mentioned in the header and documentation with 00014 solutions the user can use. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "Eyes.h" 00019 00020 00021 Eyes eyes(p28, p27, p30); // serial tx, serial rx, reset pin; 00022 int main() { 00023 while(1){ 00024 int dir = rand()%10; 00025 Eyes::DIRECTION direction; 00026 switch(dir){ 00027 case 0: 00028 direction = Eyes::U; 00029 break; 00030 case 1: 00031 direction = Eyes::D; 00032 break; 00033 case 2: 00034 direction = Eyes::L; 00035 break; 00036 case 3: 00037 direction = Eyes::R; 00038 break; 00039 case 4: 00040 direction = Eyes::C; 00041 break; 00042 case 5: 00043 direction = Eyes::UL; 00044 break; 00045 case 6: 00046 direction = Eyes::UR; 00047 break; 00048 case 7: 00049 direction = Eyes::DL; 00050 break; 00051 case 8: 00052 direction = Eyes::DR; 00053 break; 00054 } 00055 eyes.look(direction); 00056 eyes.draw(); // Call this after calling look() or express() 00057 wait((rand()%10 + 1)*0.40); 00058 eyes.gesture(Eyes::BLINK); 00059 wait((rand()%10 + 1)*0.25); 00060 if(rand()%2) eyes.gesture(Eyes::CLOSE); 00061 wait((rand()%10 + 1)*0.25); 00062 } 00063 }
Generated on Sat Jul 30 2022 07:45:56 by
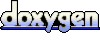