
Pong for Gamepad2
Embed:
(wiki syntax)
Show/hide line numbers
Ball.cpp
00001 #include "Ball.h" 00002 00003 Ball::Ball() 00004 { 00005 00006 } 00007 00008 Ball::~Ball() 00009 { 00010 00011 } 00012 00013 void Ball::init(int size,int speed) 00014 { 00015 _size = size; 00016 00017 _x = WIDTH/2 - _size/2; 00018 _y = HEIGHT/2 - _size/2; 00019 00020 srand(time(NULL)); 00021 int direction = rand() % 4; // randomise initial direction. 00022 00023 // 4 possibilities. Get random modulo and set velocities accordingly 00024 if (direction == 0) { 00025 _velocity.x = speed; 00026 _velocity.y = speed; 00027 } else if (direction == 1) { 00028 _velocity.x = speed; 00029 _velocity.y = -speed; 00030 } else if (direction == 2) { 00031 _velocity.x = speed; 00032 _velocity.y = speed; 00033 } else { 00034 _velocity.x = -speed; 00035 _velocity.y = -speed; 00036 } 00037 } 00038 00039 void Ball::draw(N5110 &lcd) 00040 { 00041 lcd.drawRect(_x,_y,_size,_size,FILL_BLACK); 00042 } 00043 00044 void Ball::update() 00045 { 00046 _x += _velocity.x; 00047 _y += _velocity.y; 00048 } 00049 00050 void Ball::set_velocity(Vector2D v) 00051 { 00052 _velocity.x = v.x; 00053 _velocity.y = v.y; 00054 } 00055 00056 Vector2D Ball::get_velocity() 00057 { 00058 Vector2D v = {_velocity.x,_velocity.y}; 00059 return v; 00060 } 00061 00062 Vector2D Ball::get_pos() 00063 { 00064 Vector2D p = {_x,_y}; 00065 return p; 00066 } 00067 00068 void Ball::set_pos(Vector2D p) 00069 { 00070 _x = p.x; 00071 _y = p.y; 00072 }
Generated on Sat Jul 16 2022 18:01:16 by
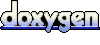