
Pong game for ELEC1620 board.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 ///////////// includes ///////////////////// 00003 #include "mbed.h" 00004 #include "platform/mbed_thread.h" 00005 #include "Joystick.h" 00006 #include "N5110.h" 00007 #include "ShiftReg.h" 00008 #include "PongEngine.h" 00009 #include "Utils.h" 00010 ///////////// defines ///////////////////// 00011 #define PADDLE_WIDTH 2 00012 #define PADDLE_HEIGHT 8 00013 #define BALL_SIZE 2 00014 #define BALL_SPEED 3 00015 ///////////// objects /////////////////// 00016 N5110 lcd(p14,p8,p9,p10,p11,p13,p21); 00017 Joystick joystick(p20,p19); 00018 DigitalIn buttonA(p29); 00019 BusOut leds(LED4,LED3,LED2,LED1); 00020 ShiftReg seven_seg; 00021 PongEngine pong; 00022 AnalogIn pot(p17); 00023 ///////////// prototypes /////////////// 00024 void init(); 00025 void render(); 00026 void welcome(); 00027 void display_lives(int lives); 00028 void game_over(); 00029 //////////////////////////////////////// 00030 00031 int main() { 00032 init(); // initialise devices and objects 00033 welcome(); // waiting for the user to start 00034 render(); // first draw the initial frame 00035 int fps = 10; 00036 thread_sleep_for(1000/fps); // and wait for one frame period - millseconds 00037 00038 int lives = 4; // display lives on LEDs 00039 display_lives(lives); 00040 00041 while (lives > 0) { // keep looping while lives remain 00042 // read the joystick input and store in a struct 00043 UserInput input = {joystick.get_direction(),joystick.get_mag()}; 00044 lives = pong.update(input); // update the game engine based on input 00045 display_lives(lives); // display lives on LEDs 00046 render(); // draw frame on screen 00047 thread_sleep_for(1000/fps); // and wait for one frame period - ms 00048 } 00049 game_over(); 00050 } 00051 00052 void init() { 00053 seven_seg.write(0x00); // turn of 7-seg display 00054 lcd.init(); 00055 lcd.setContrast(0.5); 00056 joystick.init(); 00057 pong.init(0,8,2,2,2); // paddle x position, paddle_height,paddle_width,ball_size,speed 00058 } 00059 00060 void render() { // clear screen, re-draw and refresh 00061 lcd.clear(); 00062 pong.draw(lcd); 00063 lcd.refresh(); 00064 } 00065 00066 void welcome() { // splash screen 00067 lcd.printString(" Pong! ",0,1); 00068 lcd.printString(" Press A ",0,4); 00069 lcd.refresh(); 00070 00071 // wait flashing LEDs until button A is pressed 00072 while (buttonA.read() == 0) { 00073 leds = 0b1111; 00074 thread_sleep_for(100); 00075 leds = 0b0000; 00076 thread_sleep_for(100); 00077 } 00078 } 00079 00080 void display_lives(int lives) { 00081 if (lives == 4) { 00082 leds = 0b1111; 00083 seven_seg.write(0x66); 00084 } else if (lives == 3) { 00085 leds = 0b1110; 00086 seven_seg.write(0x4F); 00087 } else if (lives == 2) { 00088 leds = 0b1100; 00089 seven_seg.write(0x5B); 00090 } else if (lives == 1) { 00091 leds = 0b1000; 00092 seven_seg.write(0x06); 00093 } else { 00094 leds = 0b0000; 00095 seven_seg.write(0x3F); 00096 } 00097 } 00098 00099 void game_over() { // splash screen 00100 while (1) { 00101 lcd.clear(); 00102 lcd.printString(" Game Over ",0,2); 00103 lcd.printString(" Loser! ",0,4); 00104 lcd.refresh(); 00105 leds = 0b1111; 00106 thread_sleep_for(250); 00107 lcd.clear(); 00108 lcd.refresh(); 00109 leds = 0b0000; 00110 thread_sleep_for(250); 00111 } 00112 }
Generated on Thu Jul 14 2022 15:46:36 by
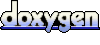