
Pong game for ELEC1620 board.
Embed:
(wiki syntax)
Show/hide line numbers
PongEngine.cpp
00001 #include "PongEngine.h" 00002 00003 PongEngine::PongEngine(){ _lives = 4; } 00004 00005 void PongEngine::init(int paddle_position, int paddle_height, int paddle_width, int ball_size, int speed){ 00006 //printf("Pong Engine: Init\n"); 00007 _ball.init(ball_size,speed); 00008 _paddle.init(paddle_position, paddle_height, paddle_width); 00009 } 00010 00011 int PongEngine::update(UserInput input) { 00012 //printf("Pong Engine: Update\n"); 00013 check_goal(); // checking for a goal is a priority 00014 _ball.update(); 00015 _paddle.update(input); 00016 // important to update paddles and ball before checking collisions so can 00017 // correct for it before updating the display 00018 check_wall_collision(); 00019 check_paddle_collision(); 00020 00021 return _lives; 00022 } 00023 00024 void PongEngine::draw(N5110 &lcd) { 00025 //printf("Pong Engine: Draw\n"); 00026 // draw the elements in the LCD buffer 00027 // pitch 00028 lcd.drawLine(0,0,WIDTH-1,0,1); // top 00029 lcd.drawLine(WIDTH-1,0,WIDTH-1,HEIGHT-1,1); // back wall 00030 lcd.drawLine(0,HEIGHT-1,WIDTH-1,HEIGHT-1,1); // bottom 00031 _ball.draw(lcd); 00032 _paddle.draw(lcd); 00033 } 00034 00035 void PongEngine::check_wall_collision() { 00036 //printf("Pong Engine: Check Wall Collision\n"); 00037 // read current ball attributes 00038 Position2D ball_pos = _ball.get_pos(); 00039 Position2D ball_velocity = _ball.get_velocity(); 00040 int size = _ball.get_size(); 00041 00042 // check if hit top wall 00043 if (ball_pos.y <= 1) { // 1 due to 1 pixel boundary 00044 ball_pos.y = 1; // bounce off ceiling without going off screen 00045 ball_velocity.y = -ball_velocity.y; // flip velocity 00046 } else if (ball_pos.y + size >= (HEIGHT-1) ) { 00047 // hit bottom 00048 ball_pos.y = (HEIGHT-1) - size; // stops ball going off screen 00049 ball_velocity.y = -ball_velocity.y; // flip velcoity 00050 } else if (ball_pos.x + size >= (WIDTH-1) ) { 00051 // hit right wall 00052 ball_pos.x = (WIDTH-1) - size; // stops ball going off screen 00053 ball_velocity.x = -ball_velocity.x; // flip velcoity 00054 } 00055 00056 // update ball parameters 00057 _ball.set_velocity(ball_velocity); 00058 _ball.set_pos(ball_pos); 00059 } 00060 00061 void PongEngine::check_paddle_collision() { 00062 //printf("Pong Engine: Check Paddle Collision\n"); 00063 // read current ball and paddle attributes 00064 Position2D ball_pos = _ball.get_pos(); 00065 Position2D ball_velocity = _ball.get_velocity(); 00066 Position2D paddle_pos = _paddle.get_pos(); // paddle 00067 00068 // see if ball has hit the paddle by checking for overlaps 00069 if ( 00070 (ball_pos.y >= paddle_pos.y) && //top 00071 (ball_pos.y <= paddle_pos.y + _paddle.get_height() ) && //bottom 00072 (ball_pos.x >= paddle_pos.x) && //left 00073 (ball_pos.x <= paddle_pos.x + _paddle.get_width() ) //right 00074 ) { 00075 // if it has, fix position and reflect x velocity 00076 ball_pos.x = paddle_pos.x + _paddle.get_width(); 00077 ball_velocity.x = -ball_velocity.x; 00078 } 00079 00080 // write new attributes 00081 _ball.set_velocity(ball_velocity); 00082 _ball.set_pos(ball_pos); 00083 } 00084 00085 void PongEngine::check_goal() { 00086 //printf("Pong Engine: Check Goal\n"); 00087 Position2D ball_pos = _ball.get_pos(); 00088 int size = _ball.get_size(); 00089 int speed = abs(_ball.get_velocity().x); // speed is magnitude of velocity 00090 // check if ball position has gone off the left 00091 if (ball_pos.x + size < 0) { 00092 // reset the ball 00093 _ball.init(size,speed); 00094 _lives--; // lose a life 00095 } 00096 }
Generated on Thu Jul 14 2022 15:46:36 by
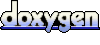