
Pong game for ELEC1620 board.
Embed:
(wiki syntax)
Show/hide line numbers
Joystick.h
00001 #ifndef JOYSTICK_H 00002 #define JOYSTICK_H 00003 00004 #include "mbed.h" 00005 #include "Utils.h" 00006 00007 // this value can be tuned to alter tolerance of joystick movement 00008 #define TOL 0.1f 00009 #define RAD2DEG 57.2957795131f 00010 00011 00012 00013 /** Joystick Class 00014 @author Dr Craig A. Evans, University of Leeds 00015 @brief Library for interfacing with analogue joystick 00016 00017 Example: 00018 00019 @code 00020 00021 #include "mbed.h" 00022 #include "Joystick.h" 00023 00024 // y x button 00025 Joystick joystick(PTB11,PTB10); 00026 00027 int main() { 00028 00029 joystick.init(); 00030 00031 while(1) { 00032 00033 Vector2D coord = joystick.get_coord(); 00034 printf("Coord = %f,%f\n",coord.x,coord.y); 00035 00036 Vector2D mapped_coord = joystick.get_mapped_coord(); 00037 printf("Mapped coord = %f,%f\n",mapped_coord.x,mapped_coord.y); 00038 00039 float mag = joystick.get_mag(); 00040 float angle = joystick.get_angle(); 00041 printf("Mag = %f Angle = %f\n",mag,angle); 00042 00043 Direction d = joystick.get_direction(); 00044 printf("Direction = %i\n",d); 00045 00046 00047 wait(0.5); 00048 } 00049 00050 00051 } 00052 00053 * @endcode 00054 */ 00055 class Joystick 00056 { 00057 public: 00058 00059 // y-pot x-pot 00060 Joystick(PinName vertPin,PinName horizPin); 00061 00062 void init(); // needs to be called at start with joystick centred 00063 float get_mag(); // polar 00064 float get_angle(); // polar 00065 Vector2D get_coord(); // cartesian co-ordinates x,y 00066 Vector2D get_mapped_coord(); // x,y mapped to circle 00067 Direction get_direction(); // N,NE,E,SE etc. 00068 Polar get_polar(); // mag and angle in struct form 00069 00070 private: 00071 00072 AnalogIn *vert; 00073 AnalogIn *horiz; 00074 00075 // centred x,y values 00076 float _x0; 00077 float _y0; 00078 00079 }; 00080 00081 #endif
Generated on Thu Jul 14 2022 15:46:36 by
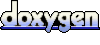