
Pong game for ELEC1620 board.
Embed:
(wiki syntax)
Show/hide line numbers
Ball.h
00001 #ifndef BALL_H 00002 #define BALL_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Utils.h" // for Position 00007 00008 00009 /** Ball Class 00010 @author Dr Craig A. Evans, University of Leeds 00011 @brief Controls the ball in the Pong game 00012 @date Febraury 2017 00013 */ 00014 00015 class Ball 00016 { 00017 00018 public: 00019 Ball(); 00020 void init(int size,int speed); 00021 void draw(N5110 &lcd); 00022 void update(); 00023 /// accessors and mutators 00024 void set_velocity(Position2D v); 00025 Position2D get_velocity(); 00026 Position2D get_pos(); 00027 int get_size(); 00028 void set_pos(Position2D p); 00029 00030 private: 00031 00032 Position2D _velocity; 00033 int _size; 00034 int _x; 00035 int _y; 00036 }; 00037 #endif
Generated on Thu Jul 14 2022 15:46:36 by
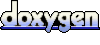