
Pong game for ELEC1620 board.
Embed:
(wiki syntax)
Show/hide line numbers
Ball.cpp
00001 #include "Ball.h" 00002 00003 Ball::Ball() {} 00004 00005 void Ball::init(int size, int speed) { 00006 //printf("Ball: Init\n"); 00007 _size = size; 00008 _x = WIDTH/2 - _size/2; 00009 _y = HEIGHT/2 - _size/2; 00010 00011 _velocity.x = speed; 00012 _velocity.y = speed; 00013 } 00014 00015 void Ball::draw(N5110 &lcd) { 00016 //printf("Ball: Draw\n"); 00017 lcd.drawRect(_x,_y,_size,_size,FILL_BLACK); 00018 } 00019 00020 void Ball::update(){ 00021 //printf("Ball: Update\n"); 00022 _x += _velocity.x; 00023 _y += _velocity.y; 00024 } 00025 00026 void Ball::set_velocity(Position2D v){ 00027 printf("Ball: Velocity\n"); 00028 _velocity.x = v.x; 00029 _velocity.y = v.y; 00030 } 00031 00032 void Ball::set_pos(Position2D p) { 00033 printf("Ball: Set Position\n"); 00034 _x = p.x; 00035 _y = p.y; 00036 } 00037 00038 Position2D Ball::get_velocity(){ return {_velocity.x,_velocity.y}; } 00039 00040 Position2D Ball::get_pos() { return {_x,_y}; } 00041 00042 int Ball::get_size() { return _size; } 00043
Generated on Thu Jul 14 2022 15:46:36 by
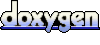