
Example solution
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* ELEC1620 Lab 2 Task 10 00002 00003 Bit-wise Operators 00004 00005 (c) Dr Craig A. Evans, Feb 2017 00006 00007 */ 00008 #include "mbed.h" 00009 00010 int main() 00011 { 00012 char a = 34; // 0010 0010 00013 char b = 107; // 0110 1011 00014 printf("a = %i\n",a); 00015 printf("b = %i\n",b); 00016 00017 printf("We'll shift a by one place to the left and store in c.\n"); 00018 printf("This is the same as multiplying by 2.\n"); 00019 char c = a << 1; // 0100 0100 = 64 + 4 = 68 00020 printf("c = %i\n",c); 00021 00022 printf("We'll shift b by fives place to the right and store in d.\n"); 00023 printf("This is the same as dividing by 2^5 = 32.\n"); 00024 char d = b >> 5; // 0000 0011 = 2 + 1 = 3 00025 printf("d = %i\n",d); 00026 printf("Remember, these are integers so we lose the decimal places.\n"); 00027 00028 printf("Now we'll set bit 6 of c using the bit-wise OR operation.\n"); 00029 char e = c | (1<<6); // 0100 0100 | 0100 0000 00030 printf("It turns out bit 6 is already set and so the value doesn't change.\n"); 00031 printf("e = %i\n",e); 00032 00033 printf("Finally we'll clear bit 2 of c using the bit-wise AND and NOT operation.\n"); 00034 char f = c & ~(1<<2); // 0100 0100 & 1111 1011 = 0100 0000 00035 printf("f = %i\n",f); 00036 }
Generated on Mon Aug 29 2022 02:35:00 by
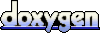