
I2C code testing
Dependencies: FreescaleIAP SimpleDMA mbed-rtos mbed
Fork of COM_MNG_TMTC_SIMPLE_pl123 by
dmaSPIslave.h
00001 #ifdef TARGET_KL46Z 00002 class dmaSPISlave : public SPISlave{ 00003 public: 00004 /* 00005 @brief: constructor : initialise the spi slave pins 00006 @param: mosi, miso, sclk, ssel 00007 @return: none 00008 */ 00009 dmaSPISlave(PinName mosi, PinName miso, PinName sclk, PinName ssel) : SPISlave(mosi, miso, sclk, ssel){ 00010 // trigger appropriate spi for dma 00011 if(_spi.spi == SPI0){ 00012 read_dma.trigger(Trigger_SPI0_RX); 00013 } 00014 else{ 00015 read_dma.trigger(Trigger_SPI1_RX); 00016 } 00017 00018 // set source for spi slave dma : mosi 00019 read_dma.source(&_spi.spi->DL, false); 00020 } 00021 00022 /* 00023 @brief: initialise the dma buffer to store the recevied data 00024 @param: read_data : pointer to the buffer 00025 len : length in bytes to store in the buffer 00026 fun : address of the function to attach to the dma interrupt, interrupt is called when the len num of bytes are written to the buffer 00027 @return: none 00028 */ 00029 void bulkRead_init(uint8_t *read_data, int len, void (*fun)(void) ){ 00030 // acquire(); 00031 _spi.spi->C2 |= SPI_C2_RXDMAE_MASK; 00032 00033 // auto increment is true 00034 read_dma.destination(read_data, true); 00035 00036 // specify length 00037 length = len; 00038 00039 // attach interrupt function 00040 read_dma.attach(fun); 00041 00042 } 00043 00044 /* 00045 @brief: start the dma read process : has to be done everytime the buffer gets filled, can be used repeatedly 00046 @param: none 00047 @return: none 00048 */ 00049 void bulkRead_start(){ 00050 // start the read_dma 00051 read_dma.start(length); 00052 } 00053 00054 /* 00055 @brief: end dma process and return back to normal spi mode 00056 @param: none 00057 @return: none 00058 */ 00059 void bulkRead_end(){ 00060 // turn off dma 00061 _spi.spi->C2 &= ~(SPI_C2_RXDMAE_MASK); 00062 } 00063 00064 private: 00065 int length; 00066 SimpleDMA read_dma; 00067 }; 00068 #endif
Generated on Sat Jul 16 2022 17:06:14 by
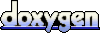