
I2C code testing
Dependencies: FreescaleIAP SimpleDMA mbed-rtos mbed
Fork of COM_MNG_TMTC_SIMPLE_pl123 by
cdms_rtc.h
00001 void FCTN_CDMS_INIT_RTC() 00002 { 00003 SPI_mutex.lock(); 00004 gCS_RTC=1; 00005 spi.format(8,0); 00006 spi.frequency(1000000); 00007 //clearing the halt bit 00008 gCS_RTC=1; 00009 gCS_RTC=0; 00010 spi.write(0x8C); 00011 spi.write(0x00); 00012 00013 //clearing the OF bit 00014 gCS_RTC=1; 00015 gCS_RTC=0; 00016 spi.write(0x8F); 00017 spi.write(0x00); 00018 00019 //century bits 00020 gCS_RTC=1; 00021 gCS_RTC=0; 00022 spi.write(0x80|0x03); 00023 spi.write(0x00); 00024 00025 //Kick starting the oscillator 00026 gCS_RTC=1; 00027 gCS_RTC=0; 00028 spi.write(0x81); //register address with write flag 00029 spi.write(0x80);//enabling stop bit in the seconds register 00030 00031 gCS_RTC=1; 00032 gCS_RTC=0; 00033 spi.write(0x81); 00034 spi.write(0x00);//disabling the stop bit to restart the oscillator 00035 00036 00037 gCS_RTC=1; 00038 gCS_RTC=0; 00039 spi.write(0x80); 00040 spi.write(0x01); // set milliseconds value to 00 00041 gCS_RTC=1; 00042 00043 gCS_RTC=0; 00044 spi.write(0x81); 00045 spi.write(0x01); //set seconds value to 00 00046 gCS_RTC=1; 00047 00048 gCS_RTC=0; 00049 spi.write(0x82); 00050 spi.write(0x01);//set minutes value to 00 00051 gCS_RTC=1; 00052 00053 gCS_RTC=0; 00054 spi.write(0x83); 00055 spi.write(0x23); //set the hours to 01 00056 gCS_RTC=1; 00057 00058 gCS_RTC=0; 00059 spi.write(0x84); 00060 spi.write(0x01); //set day of the week to 01 00061 gCS_RTC=1; 00062 00063 gCS_RTC=0; 00064 spi.write(0x85); 00065 spi.write(0x31); //set date of the month to 01 00066 gCS_RTC=1; 00067 00068 gCS_RTC=0; 00069 spi.write(0x86); 00070 spi.write(0x12); //set month to 01 00071 gCS_RTC=1; 00072 00073 gCS_RTC=0; 00074 spi.write(0x87); 00075 spi.write(0x01); //set year to 00(2000) 00076 gCS_RTC=1; 00077 // printf("\n\r rtc initalised \n"); 00078 SPI_mutex.unlock(); 00079 } 00080 00081 uint64_t FCTN_CDMS_RD_RTC() 00082 { 00083 SPI_mutex.lock(); 00084 uint8_t response; 00085 //printf("\n\r Entered rtc\n"); 00086 gCS_RTC=1; 00087 gCS_RTC=0; 00088 spi.write(0x00); //reading milliseconds register 00089 response = spi.write(0x00); // read the value by sending dummy byte 00090 uint8_t centiseconds = (uint8_t(response&0xF0)>>4)*10+uint8_t(response&0x0F)*1; 00091 00092 gCS_RTC=1; 00093 gCS_RTC=0; 00094 spi.write(0x01); //reading seconds register 00095 response =spi.write(0x01); 00096 uint8_t seconds = ((response&0x70)>>4)*10+(response&0x0F)*1; 00097 00098 gCS_RTC=1; 00099 gCS_RTC=0; 00100 spi.write(0x02); //reading minutes register 00101 response =spi.write(0x01); 00102 uint8_t minutes = ((response&0xF0)>>4)*10+(response&0x0F)*1; 00103 00104 gCS_RTC=1; 00105 gCS_RTC=0; 00106 spi.write(0x03); //reading hours register 00107 response=spi.write(0x01); 00108 uint8_t hours = ((response&0x30)>>4)*10+(response&0x0F)*1; 00109 00110 gCS_RTC=1; 00111 gCS_RTC=0; 00112 spi.write(0x04); //reading day's register 00113 uint8_t day =spi.write(0x01); 00114 00115 gCS_RTC=1; 00116 gCS_RTC=0; 00117 spi.write(0x05); //reading date register 00118 response =spi.write(0x01); 00119 uint8_t date = ((response&0x30)>>4)*10+(response&0x0F)*1; 00120 00121 gCS_RTC=1; 00122 gCS_RTC=0; 00123 spi.write(0x06); //reading month registe 00124 response =spi.write(0x01); 00125 uint8_t month = ((response&0x10)>>4)*10+(response&0x0F)*1; 00126 00127 gCS_RTC=1; 00128 gCS_RTC=0; 00129 spi.write(0x07); //reading year's registe 00130 response =spi.write(0x01); 00131 uint8_t year = ((response&0xF0)>>4)*10+(response&0x0F)*1; 00132 gCS_RTC=1; 00133 //sprintf(Time_stamp,"%02d%02d%02d%02d%02d%02d%02d%02d",year, month, date, day, hours, minutes, seconds, milliseconds ); 00134 uint8_t Time_stamp[8] = {year, month, date, day, hours, minutes, seconds, centiseconds}; 00135 for(int i= 0; i<8;i++); 00136 //printf("%d\t",Time_stamp[i]); 00137 //printf("read\r\n"); 00138 uint64_t time; 00139 time = 0; 00140 time = time|(((uint64_t)(centiseconds&0x7F))); 00141 time = time|(((uint64_t)(seconds&0x3F))<<7); 00142 time = time|(((uint64_t)(minutes&0x3F))<<13); 00143 time = time|(((uint64_t)(hours&0x1F))<<19); 00144 time = time|(((uint64_t)(day&0x1F))<<24); 00145 time = time|(((uint64_t)(month&0x07))<<29); 00146 time = time|(((uint64_t)(year&0x03))<<33); 00147 time = (time&0x00000007FFFFFFFF); 00148 //printf("\n\r%x%x", (int)(time >> 32), (int)(time)); 00149 //printf("\n\r0x%016llx\n\r", time); 00150 return time; 00151 SPI_mutex.unlock(); 00152 }
Generated on Sat Jul 16 2022 17:06:13 by
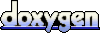