1 sensor using RTOS Timer, another 2 over RTOS Semaphore
Dependents: Autonomous_quadcopter
Fork of HCSR04 by
hcsr04.h
00001 /* File: HCSR04.h 00002 * Author: Antonio Buonanno 00003 *Board: STM NUCLEO F401RE, 00004 *Hardware: Ultrasonic Range HC-SR04, 00005 * 00006 *This work derived from Arduino library, 00007 * 00008 * Desc: driver for HCSR04 Ultrasonic Range Finder. The returned range 00009 * is in units of meters. 00010 * 00011 * 00012 * 00013 */ 00014 00015 /* EXAMPLE 00016 #include "mbed.h" 00017 #include "hcsr04.h" 00018 00019 //D12 TRIGGER D11 ECHO 00020 HCSR04 sensor(D12, D11); 00021 int main() { 00022 while(1) { 00023 00024 long distance = sensor.distance(); 00025 printf("distanza %d \n",distance); 00026 wait(1.0); // 1 sec 00027 00028 } 00029 } 00030 */ 00031 #ifndef hcsr04_H 00032 #define hcsr04_H 00033 #include "mbed.h" 00034 #include "rtos.h" 00035 00036 00037 00038 class HCSR04 { 00039 public: 00040 HCSR04(PinName t1, PinName e1); 00041 00042 float getDistan1(); 00043 uint16_t getDistan2(); 00044 00045 private: 00046 static void threadWorker1(void const *p); 00047 static void threadWorker2(void const *p); 00048 00049 DigitalOut trig1; 00050 DigitalIn echo1; 00051 00052 // sonic thread can't run longer than thread frequency 00053 uint32_t limit; 00054 00055 Timer timer1; 00056 Timer timerLimit; 00057 Timer timer2; 00058 00059 osThreadId id; 00060 00061 RtosTimer *threadUpdateTimer1; 00062 RtosTimer *threadUpdateTimer2; 00063 00064 float distan1; 00065 uint32_t distan2; 00066 bool tooLong; 00067 }; 00068 00069 #endif
Generated on Sun Jul 31 2022 21:51:24 by
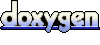