
Regenerating PPM signal based on distances from ultrasonic sensors, ESP8266 for connectin via wifi. Autonomous quadcopter behaviour, autonomou height holding. Flying direction based on front and back ultrasonic sensors.
Dependencies: ConfigFile HCSR04 PID PPM2 mbed-rtos mbed
hardware.h
00001 #ifndef HARDWARE_H 00002 #define HARDWARE_H 00003 00004 #include "mbed.h" 00005 #include "PpmRegen.h" 00006 #include "PID.h" 00007 #include "hcsr04.h" 00008 #include "ConfigFile.h" 00009 00010 00011 void loadConfigFile(void); 00012 void writeSettingsToConfig(void); 00013 void writeErrorLog(char *message); 00014 void ConvertToCharArray(float number); 00015 void ConvertToCharArray(int number); 00016 00017 Serial pc(USBTX, USBRX); // tx, rx 00018 PID* _groundDistance; 00019 //RtosTimer *_groudRegulationUpdateTimer; 00020 HCSR04* _sonic; 00021 uint8_t _frontDistance; 00022 uint8_t _leftDistance; 00023 uint8_t _rightDistance; 00024 uint8_t _backDistance; 00025 PpmRegen* _ppmRegen; 00026 00027 ConfigFile _configFile; 00028 LocalFileSystem local("local"); 00029 00030 Semaphore _semaphore(1); 00031 Thread _serverThread; 00032 Thread _frontSensorThread; 00033 Thread _leftSensorThread; 00034 Thread _rightSensorThread; 00035 Thread _backSensorThread; 00036 Thread *_distanceThread; 00037 00038 00039 InterruptIn* _interruptPin = new InterruptIn(p28); 00040 PwmOut* _roll = new PwmOut(p21); 00041 PwmOut* _pitch = new PwmOut(p22); 00042 PwmOut* _throttle = new PwmOut(p23); 00043 PwmOut* _yaw = new PwmOut(p24); 00044 PwmOut* _aux1 = new PwmOut(p25); 00045 PwmOut* _aux2 = new PwmOut(p26); 00046 00047 00048 char* _str = new char[1024]; 00049 char* _serverMessage = new char[1024]; 00050 bool _configChanges = false; 00051 bool _onlyDistanChanged = false; 00052 // zero is default value 00053 float _P = 0; 00054 float _I = 0; 00055 float _D = 0; 00056 int _groundSetPoint = 0; 00057 int _bias = 0; 00058 int _groundPidMinOutput = 0; 00059 int _groundPidMaxOutput = 0; 00060 bool _groundRegulation = false; 00061 // Temporary values for Server to filter noise 00062 float _newP; 00063 float _newI; 00064 float _newD; 00065 int _newGroundSetPoint; 00066 bool _tempGroundRegulation = false; 00067 bool _goAhead = false; 00068 bool _backWall = true; 00069 bool _frontWall = false; 00070 00071 00072 00073 00074 00075 00076 00077 void loadConfigFile(void){ 00078 //reading configFile 00079 _configFile.read("/local/config.cfg"); 00080 char value[BUFSIZ]; 00081 if (_configFile.getValue("bias", &value[0], sizeof(value))) 00082 _bias = atof(value); 00083 if (_configFile.getValue("groundPidMinOutput", &value[0], sizeof(value))) 00084 _groundPidMinOutput = atof(value); 00085 if (_configFile.getValue("groundPidMaxOutput", &value[0], sizeof(value))) 00086 _groundPidMaxOutput = atof(value); 00087 if (_configFile.getValue("P", &value[0], sizeof(value))) 00088 _P = atof(value); 00089 if (_configFile.getValue("I", &value[0], sizeof(value))) 00090 _I = atof(value); 00091 if (_configFile.getValue("D", &value[0], sizeof(value))) 00092 _D = atof(value); 00093 if (_configFile.getValue("groundSetPoint", &value[0], sizeof(value))) 00094 _groundSetPoint = atof(value); 00095 00096 } 00097 00098 void writeSettingsToConfig(void){ 00099 ConvertToCharArray(_bias); 00100 if(!_configFile.setValue("bias", _str)) 00101 strcat(_serverMessage, "bias value could not be saved to configFile\n\r"); 00102 ConvertToCharArray(_groundPidMinOutput); 00103 if(!_configFile.setValue("groundPidMinOutput", _str)) 00104 strcat(_serverMessage, "groundPidMinOutput value could not be saved to configFile\n\r"); 00105 ConvertToCharArray(_groundPidMaxOutput); 00106 if(!_configFile.setValue("groundPidMaxOutput", _str)) 00107 strcat(_serverMessage, "groundPidMaxOutput value could not be saved to configFile\n\r"); 00108 ConvertToCharArray(_P); 00109 if(!_configFile.setValue("P", _str)) 00110 strcat(_serverMessage, "P value could not be saved to configFile\n\r"); 00111 ConvertToCharArray(_I); 00112 if(!_configFile.setValue("I", _str)) 00113 strcat(_serverMessage, "I value could not be saved to configFile\n\r"); 00114 ConvertToCharArray(_D); 00115 if(!_configFile.setValue("D", _str)) 00116 strcat(_serverMessage, "D value could not be saved to configFile\n\r"); 00117 ConvertToCharArray(_groundSetPoint); 00118 if(!_configFile.setValue("groundSetPoint", _str)){ 00119 strcat(_serverMessage, "groundSetPoint value could not be saved to configFile\n\r"); 00120 pc.printf("groundSetPoint value could not be saved to configFile\n\r"); 00121 } 00122 _configFile.write("/local/config.cfg"); 00123 00124 } 00125 00126 00127 void writeErrorLog(char *message){ 00128 FILE *fp = fopen("/local/errorlog.txt", "w"); 00129 fprintf(fp, message); 00130 fclose(fp); 00131 00132 } 00133 00134 00135 //Converts float to char array 00136 void ConvertToCharArray(float number) 00137 { 00138 sprintf(_str, "%3.2f", number ); 00139 } 00140 00141 //Converts integer to char array 00142 void ConvertToCharArray(int number) 00143 { 00144 sprintf(_str, "%d", number ); 00145 } 00146 00147 00148 #endif
Generated on Sun Jul 17 2022 22:20:41 by
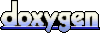