
Test Code for MMA7660FC device
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* Author: Edoardo De Marchi 00002 * Name: Test Code for MMA7660FC 00003 */ 00004 #include "mbed.h" 00005 #include "MMA7660FC.h" 00006 00007 #define ADDR_MMA7660 0x98 // I2C SLAVE ADDR MMA7660FC 00008 00009 MMA7660FC Acc(p28, p27, ADDR_MMA7660); //sda, scl, Addr 00010 Serial pc(USBTX, USBRX); 00011 00012 00013 float G_VALUE[64] = {0, 0.047, 0.094, 0.141, 0.188, 0.234, 0.281, 0.328, 0.375, 0.422, 0.469, 0.516, 0.563, 0.609, 0.656, 0.703, 0.750, 0.797, 0.844, 0.891, 0.938, 0.984, 1.031, 1.078, 1.125, 1.172, 1.219, 1.266, 1.313, 1.359, 1.406, 1.453, -1.500, -1.453, -1.406, -1.359, -1.313, -1.266, -1.219, -1.172, -1.125, -1.078, -1.031, -0.984, -0.938, -0.891, -0.844, -0.797, -0.750, -0.703, -0.656, -0.609, -0.563, -0.516, -0.469, -0.422, -0.375, -0.328, -0.281, -0.234, -0.188, -0.141, -0.094, -0.047}; 00014 00015 00016 00017 int main() 00018 { 00019 00020 Acc.init(); // Initialization 00021 pc.printf("Value reg 0x06: %#x\n", Acc.read_reg(0x06)); // Test the correct value of the register 0x06 00022 pc.printf("Value reg 0x08: %#x\n", Acc.read_reg(0x08)); // Test the correct value of the register 0x08 00023 pc.printf("Value reg 0x07: %#x\n\r", Acc.read_reg(0x07)); // Test the correct value of the register 0x07 00024 00025 while(1) 00026 { 00027 float x=0, y=0, z=0; 00028 00029 Acc.read_Tilt(&x, &y, &z); // Read the acceleration 00030 pc.printf("Tilt x: %2.2f degree \n", x); // Print the tilt orientation of the X axis 00031 pc.printf("Tilt y: %2.2f degree \n", y); // Print the tilt orientation of the Y axis 00032 pc.printf("Tilt z: %2.2f degree \n", z); // Print the tilt orientation of the Z axis 00033 00034 wait_ms(100); 00035 00036 pc.printf("x: %1.3f g \n", G_VALUE[Acc.read_x()]); // Print the X axis acceleration 00037 pc.printf("y: %1.3f g \n", G_VALUE[Acc.read_y()]); // Print the Y axis acceleration 00038 pc.printf("z: %1.3f g \n", G_VALUE[Acc.read_z()]); // Print the Z axis acceleration 00039 pc.printf("\n"); 00040 wait(5); 00041 00042 } 00043 } 00044
Generated on Tue Jul 12 2022 19:22:00 by
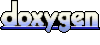