
Example of code that returns information about the status of the Ethernet connection.
Dependencies: EthernetInterface mbed-rtos mbed
main.cpp
00001 /* 00002 * Author: Edoardo De Marchi 00003 * Date: 15/02/14 00004 * Notes: Checks the Ethernet cable connection 00005 */ 00006 00007 00008 #include "mbed.h" 00009 #include "EthernetInterface.h" 00010 #include "eth_status.h" 00011 00012 DigitalOut led1(LED1); 00013 DigitalOut led2(LED2); 00014 DigitalOut led3(LED3); 00015 DigitalOut led4(LED4); 00016 00017 00018 //ETHERNET 00019 #define ECHO_SERVER_PORT 2000 00020 00021 char* ip = "192.168.153.153"; // ip 00022 char* mask = "255.255.255.0"; // mask 00023 char* gateway = "192.168.153.100"; // gateway 00024 EthernetInterface eth; 00025 TCPSocketConnection client; 00026 TCPSocketServer server; 00027 00028 00029 int Init() 00030 { 00031 led1 = 0; 00032 led2 = 0; 00033 led3 = 0; 00034 led4 = 0; 00035 00036 00037 // ETHERNET 00038 eth.init(ip, mask, gateway); 00039 eth.connect(); 00040 server.bind(ECHO_SERVER_PORT); 00041 server.listen(1); 00042 printf("IP Address is %s\r\n", eth.getIPAddress()); 00043 printf("%s - Speed: %d Mbps\n", get_transmission_status(), get_connection_speed()); 00044 00045 return 0; 00046 } 00047 00048 00049 00050 int main() 00051 { 00052 bool eth_status = false; 00053 bool eth_status_temp = true; 00054 00055 Init(); 00056 00057 00058 while(true) 00059 { 00060 printf("\nWait for new connection...\n"); 00061 server.accept(client); 00062 00063 printf("Connection from: %s\n", client.get_address()); 00064 00065 while (true) 00066 { 00067 if(!get_link_status()) 00068 { 00069 eth_status = true; 00070 }else 00071 { 00072 eth_status = false; 00073 eth_status_temp = true; 00074 led1 = 0; 00075 } 00076 00077 if(eth_status == eth_status_temp) 00078 { 00079 eth_status_temp = !eth_status; 00080 printf("Check cable connection\r\n"); 00081 led1 = 1; 00082 eth_status = false; 00083 } 00084 led2 = !led2; 00085 osDelay(500); 00086 } 00087 } 00088 }
Generated on Tue Jul 19 2022 16:06:08 by
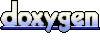