
Example of code that returns information about the status of the Ethernet connection.
Dependencies: EthernetInterface mbed-rtos mbed
eth_status.h
00001 #include "lpc_phy.h" 00002 00003 /** \brief DP83848 PHY status definitions */ 00004 #define DP8_REMOTEFAULT (1 << 6) /**< Remote fault */ 00005 #define DP8_FULLDUPLEX (1 << 2) /**< 1=full duplex */ 00006 #define DP8_SPEED10MBPS (1 << 1) /**< 1=10MBps speed */ 00007 #define DP8_VALID_LINK (1 << 0) /**< 1=Link active */ 00008 00009 00010 // This function returns the current status of connection. 00011 static bool get_link_status() 00012 { 00013 u32_t tmp = lpc_mii_read_data(); 00014 return (tmp & DP8_VALID_LINK) ? true : false; 00015 } 00016 00017 // This function returns the status of transmission. 00018 static char* get_transmission_status() 00019 { 00020 u32_t tmp = lpc_mii_read_data(); 00021 if(tmp & DP8_FULLDUPLEX) 00022 { 00023 return "FULL DUPLEX"; 00024 }else 00025 { 00026 return "HALF DUPLEX"; 00027 } 00028 } 00029 00030 // This function returns the speed of the connection. 00031 static int get_connection_speed() 00032 { 00033 u32_t tmp = lpc_mii_read_data(); 00034 return (tmp & DP8_SPEED10MBPS) ? 10 : 100; 00035 } 00036 00037 // This function returns the current value in the MII data register. 00038 static u32_t mii_read_data() 00039 { 00040 return lpc_mii_read_data(); // 16-bit MRDD - address 0x2008 4030 00041 }
Generated on Tue Jul 19 2022 16:06:08 by
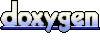