Edited version of the wave player class that uses the MODDMA library to handle transfers to the DAC.
Dependents: WavePlayer_MODDMA wave_player_DMA_mbed
wave_player.h
00001 #include <mbed.h> 00002 #include "MODDMA.h" 00003 00004 #define BUF_SIZE 1000 00005 00006 typedef struct uFMT_STRUCT { 00007 short comp_code; 00008 short num_channels; 00009 unsigned sample_rate; 00010 unsigned avg_Bps; 00011 short block_align; 00012 short sig_bps; 00013 } FMT_STRUCT; 00014 00015 00016 /** wave file player class. 00017 * 00018 * Example: 00019 * @code 00020 * #include <mbed.h> 00021 * #include <wave_player.h> 00022 * 00023 * AnalogOut DACout(p18); 00024 * wave_player waver(&DACout); 00025 * 00026 * int main() { 00027 * FILE *wave_file; 00028 * 00029 * printf("\n\n\nHello, wave world!\n"); 00030 * wave_file=fopen("/sd/44_8_st.wav","r"); 00031 * waver.play(wave_file); 00032 * fclose(wave_file); 00033 * } 00034 * @endcode 00035 */ 00036 class wave_player { 00037 00038 public: 00039 /** Create a wave player using a pointer to the given AnalogOut object. 00040 * 00041 * @param _dac pointer to an AnalogOut object to which the samples are sent. 00042 */ 00043 wave_player(AnalogOut *_dac); 00044 00045 /** the player function. 00046 * 00047 * @param wavefile A pointer to an opened wave file 00048 */ 00049 void play(FILE *wavefile); 00050 00051 /** Set the printf verbosity of the wave player. A nonzero verbosity level 00052 * will put wave_player in a mode where the complete contents of the wave 00053 * file are echoed to the screen, including header values, and including 00054 * all of the sample values placed into the DAC FIFO, and the sample values 00055 * removed from the DAC FIFO by the ISR. The sample output frequency is 00056 * fixed at 2 Hz in this mode, so it's all very slow and the DAC output isn't 00057 * very useful, but it lets you see what's going on and may help for debugging 00058 * wave files that don't play correctly. 00059 * 00060 * @param v the verbosity level 00061 */ 00062 void set_verbosity(int v); 00063 00064 private: 00065 int verbosity; 00066 AnalogOut *wave_DAC; 00067 MODDMA dma; 00068 MODDMA_Config *conf0; 00069 MODDMA_Config *conf1; 00070 short DAC_on; 00071 uint32_t DAC_buf0[BUF_SIZE]; 00072 uint32_t DAC_buf1[BUF_SIZE]; 00073 void TC0_callback(void); 00074 void ERR0_callback(void); 00075 void TC1_callback(void); 00076 void ERR1_callback(void); 00077 bool dma0_fin_flag; 00078 bool dma1_fin_flag; 00079 }; 00080 00081
Generated on Fri Jul 15 2022 01:28:23 by
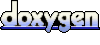