digital light sensor using ardunio i2c pins
Dependents: LoRaWAN_singlechannel_endnode
Fork of TSL2561 by
TSL2561.cpp
00001 00002 #include "TSL2561.h" 00003 #include "mbed.h" 00004 00005 /*Serial DEBUG(USBTX, USBRX); 00006 00007 #define DEBUG_PRINTX(z,x) if(z==1) DEBUG.printf(x); 00008 #define DEBUG_PRINTLNX(z,x) if(z==1) {DEBUG.printf(x); DEBUG.printf("\r\n");} 00009 #define DEBUG_PRINTXY(z,x, y) if(z==1) DEBUG.printf(x, y); 00010 #define DEBUG_PRINTLNXY(z,x, y) if(z==1) {DEBUG.printf(x, y); DEBUG.printf("\r\n");} 00011 */ 00012 00013 TSL2561::TSL2561():i2c(I2C_SDA, I2C_SCL){ 00014 i2c.frequency (300); 00015 _addr = TSL2561_ADDR_FLOAT; 00016 _initialized = false; 00017 _integration = TSL2561_INTEGRATIONTIME_13MS; 00018 _gain = TSL2561_GAIN_16X; 00019 } 00020 00021 TSL2561::TSL2561(uint8_t addr):i2c(I2C_SDA, I2C_SCL) { 00022 00023 _addr = addr; 00024 _initialized = false; 00025 _integration = TSL2561_INTEGRATIONTIME_13MS; 00026 _gain = TSL2561_GAIN_16X; 00027 // we cant do wire initialization till later, because we havent loaded Wire yet 00028 } 00029 00030 TSL2561::TSL2561(PinName sda, PinName scl):i2c(sda, scl) { 00031 00032 _addr = TSL2561_ADDR_FLOAT; 00033 _initialized = false; 00034 _integration = TSL2561_INTEGRATIONTIME_13MS; 00035 _gain = TSL2561_GAIN_16X; 00036 // we cant do wire initialization till later, because we havent loaded Wire yet 00037 } 00038 00039 TSL2561::TSL2561(PinName sda, PinName scl, uint8_t addr):i2c(sda, scl) { 00040 00041 _addr = addr; 00042 _initialized = false; 00043 _integration = TSL2561_INTEGRATIONTIME_13MS; 00044 _gain = TSL2561_GAIN_16X; 00045 // we cant do wire initialization till later, because we havent loaded Wire yet 00046 } 00047 00048 uint16_t TSL2561::getLuminosity (uint8_t channel) { 00049 00050 uint32_t x = getFullLuminosity(); 00051 00052 if (channel == 0) { 00053 // Reads two byte value from channel 0 (visible + infrared) 00054 00055 return (x & 0xFFFF); 00056 } else if (channel == 1) { 00057 // Reads two byte value from channel 1 (infrared) 00058 00059 return (x >> 16); 00060 } else if (channel == 2) { 00061 // Reads all and subtracts out just the visible! 00062 00063 return ( (x & 0xFFFF) - (x >> 16) ); 00064 } 00065 00066 // unknown channel! 00067 return 0; 00068 } 00069 00070 uint32_t TSL2561::getFullLuminosity (void) 00071 { 00072 if (!_initialized) begin(); 00073 00074 // Enable the device by setting the control bit to 0x03 00075 enable(); 00076 00077 // Wait x ms for ADC to complete 00078 switch (_integration) 00079 { 00080 case TSL2561_INTEGRATIONTIME_13MS: 00081 ThisThread::sleep_for(14);//wait_ms(14); 00082 break; 00083 case TSL2561_INTEGRATIONTIME_101MS: 00084 ThisThread::sleep_for(102);//wait_ms(102); 00085 break; 00086 default: 00087 ThisThread::sleep_for(403);//wait_ms(403); 00088 break; 00089 } 00090 00091 //DEBUG_PRINTLNXY(0," Integration:= %d",_integration); 00092 00093 uint32_t x; 00094 x = read16(TSL2561_COMMAND_BIT | TSL2561_WORD_BIT | TSL2561_REGISTER_CHAN1_LOW); 00095 00096 // DEBUG_PRINTLNXY(0," x:= %d",x); 00097 00098 x <<= 16; 00099 x |= read16(TSL2561_COMMAND_BIT | TSL2561_WORD_BIT | TSL2561_REGISTER_CHAN0_LOW); 00100 00101 //DEBUG_PRINTLNXY(0," x:= %d",x); 00102 00103 //wait(3); 00104 disable(); 00105 00106 return x; 00107 } 00108 00109 00110 bool TSL2561::begin(void) { 00111 00112 char reg[1]; 00113 reg[0] = TSL2561_REGISTER_ID; 00114 char receivedata[1]; 00115 char read; 00116 i2c.write(_addr<<1, reg, 1); 00117 i2c.read(_addr<<1, receivedata, 1); 00118 read=receivedata[0]; 00119 00120 if (read & 0x0A ) { 00121 printf("Read 0x%x => Found TSL2561\r\n",read); 00122 } else { 00123 printf("read:%x\r\n", read); 00124 return false; 00125 } 00126 _initialized = true; 00127 00128 // Set default integration time and gain 00129 setTiming(_integration); 00130 setGain(_gain); 00131 00132 // Note: by default, the device is in power down mode on bootup 00133 disable(); 00134 00135 return true; 00136 } 00137 00138 uint16_t TSL2561::read16(uint8_t reg) 00139 { 00140 uint16_t x; 00141 uint16_t t; 00142 char _x; 00143 char _t; 00144 char r[1]; 00145 r[0] = reg; 00146 char receivedata[2]; 00147 00148 i2c.write(_addr<<1, r, 1); 00149 i2c.read(_addr<<1, receivedata, 2); 00150 00151 _t=receivedata[0]; 00152 _x=receivedata[1]; 00153 00154 /*printf("_t:=0x%x",_t); 00155 printf("_x:=0x%x",_x); */ 00156 00157 t=(uint16_t)_t; 00158 x=(uint16_t)_x; 00159 x <<= 8; 00160 x |= t; 00161 00162 //printf("x:= %d\r\n",x); 00163 00164 return x; 00165 } 00166 00167 void TSL2561::write8 (uint8_t reg, uint8_t value) 00168 { 00169 i2c.start(); 00170 i2c.write(_addr<<1); 00171 i2c.write(reg); 00172 i2c.write(value); 00173 i2c.stop(); 00174 } 00175 00176 void TSL2561::setTiming(tsl2561IntegrationTime_t integration){ 00177 00178 if (!_initialized) 00179 begin(); 00180 /*else 00181 printf("--------------Set Timing---------\r\n");*/ 00182 00183 enable(); 00184 00185 _integration = integration; 00186 00187 /*printf("Integration: 0x%x ",_integration); 00188 printf("Gain: 0x%x ",_gain); 00189 printf("Integration | Gain: 0x%x\r\n",_integration | _gain);*/ 00190 00191 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _integration | _gain); 00192 00193 disable(); 00194 00195 //printf("--------------Complete Set Timing-------------\r\n"); 00196 00197 //wait(1); 00198 00199 } 00200 00201 void TSL2561::setGain(tsl2561Gain_t gain) { 00202 00203 if (!_initialized) 00204 begin(); 00205 /*else 00206 printf("-------------Set Gain--------------\r\n");*/ 00207 00208 00209 enable(); 00210 00211 /*printf("Intergration: 0x%x ",_integration); 00212 printf("Gain: 0x%x ",_gain); 00213 printf("Intergration | Gain: 0x%x\r\n",_integration | _gain);*/ 00214 00215 _gain = gain; 00216 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _integration | _gain); 00217 //write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _gain); 00218 disable(); 00219 00220 //printf("---------------Complete Set Gain----------------\r\n"); 00221 //wait(1); 00222 00223 } 00224 00225 void TSL2561::enable(void) 00226 { 00227 if (!_initialized) begin(); 00228 00229 // Enable the device by setting the control bit to 0x03 00230 //printf("Power On\r\n"); 00231 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_CONTROL, TSL2561_CONTROL_POWERON); 00232 } 00233 00234 void TSL2561::disable(void) 00235 { 00236 if (!_initialized) begin(); 00237 00238 // Disable the device by setting the control bit to 0x03 00239 //printf(" Power Off\r\n"); 00240 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_CONTROL, TSL2561_CONTROL_POWEROFF); 00241 }
Generated on Sat Jul 16 2022 13:15:48 by
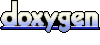