
test sending sensor results over lora radio. Accelerometer and temp/pressure.
Functions | |
static DrvStatusTypeDef | LIS2DH12_FIFO_Get_Overrun_Status (DrvContextTypeDef *handle, uint8_t *status) |
Get status of FIFO_OVR flag. | |
static DrvStatusTypeDef | LIS2DH12_FIFO_Get_Num_Of_Samples (DrvContextTypeDef *handle, uint16_t *nSamples) |
Get number of unread FIFO samples. | |
static DrvStatusTypeDef | LIS2DH12_FIFO_Set_Mode (DrvContextTypeDef *handle, uint8_t mode) |
Set FIFO mode. | |
static DrvStatusTypeDef | LIS2DH12_FIFO_Set_INT1_FIFO_Overrun (DrvContextTypeDef *handle, uint8_t status) |
Set FIFO_OVR interrupt on INT1 pin. | |
static DrvStatusTypeDef | LIS2DH12_Get_AxesSuperRaw (DrvContextTypeDef *handle, int16_t *pData, ACTIVE_AXIS_t axl_axis) |
Get the LIS2DH12 accelerometer sensor super raw axes. | |
static DrvStatusTypeDef | LIS2DH12_Get_OpMode (DrvContextTypeDef *handle, OP_MODE_t *axl_opMode) |
Get the Operating Mode of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Set_OpMode (DrvContextTypeDef *handle, OP_MODE_t axl_opMode) |
Set the Operating Mode of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Get_Active_Axis (DrvContextTypeDef *handle, ACTIVE_AXIS_t *axl_axis) |
Get the active axis of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Set_Active_Axis (DrvContextTypeDef *handle, ACTIVE_AXIS_t axl_axis) |
Set the active axis of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Enable_HP_Filter (DrvContextTypeDef *handle) |
Enable the HP Filter of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Disable_HP_Filter (DrvContextTypeDef *handle) |
Disable the HP Filter of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_ClearDRDY (DrvContextTypeDef *handle, ACTIVE_AXIS_t axl_axis) |
Clear DRDY of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Set_INT1_DRDY (DrvContextTypeDef *handle, INT1_DRDY_CONFIG_t axl_drdyStatus) |
Set DRDY enable/disable of the LIS2DH12 sensor on INT1. |
Function Documentation
static DrvStatusTypeDef LIS2DH12_ClearDRDY | ( | DrvContextTypeDef * | handle, |
ACTIVE_AXIS_t | axl_axis | ||
) | [static] |
Clear DRDY of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1531 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Disable_HP_Filter | ( | DrvContextTypeDef * | handle ) | [static] |
Disable the HP Filter of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1514 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Enable_HP_Filter | ( | DrvContextTypeDef * | handle ) | [static] |
Enable the HP Filter of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle mode the HP Filter mode to be set cutoff the HP Filter cutoff frequency to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1487 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_FIFO_Get_Num_Of_Samples | ( | DrvContextTypeDef * | handle, |
uint16_t * | nSamples | ||
) | [static] |
Get number of unread FIFO samples.
- Parameters:
-
handle the device handle *nSamples Number of unread FIFO samples
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1202 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_FIFO_Get_Overrun_Status | ( | DrvContextTypeDef * | handle, |
uint8_t * | status | ||
) | [static] |
Get status of FIFO_OVR flag.
- Parameters:
-
handle the device handle *status The pointer where the status of FIFO is stored
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1182 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_FIFO_Set_INT1_FIFO_Overrun | ( | DrvContextTypeDef * | handle, |
uint8_t | status | ||
) | [static] |
Set FIFO_OVR interrupt on INT1 pin.
- Parameters:
-
handle the device handle status FIFO_OVR interrupt on INT1 pin enable/disable
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1259 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_FIFO_Set_Mode | ( | DrvContextTypeDef * | handle, |
uint8_t | mode | ||
) | [static] |
Set FIFO mode.
- Parameters:
-
handle the device handle mode FIFO mode
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1226 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_Active_Axis | ( | DrvContextTypeDef * | handle, |
ACTIVE_AXIS_t * | axl_axis | ||
) | [static] |
Get the active axis of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle axis pointer to the value of active axis
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1411 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_AxesSuperRaw | ( | DrvContextTypeDef * | handle, |
int16_t * | pData, | ||
ACTIVE_AXIS_t | axl_axis | ||
) | [static] |
Get the LIS2DH12 accelerometer sensor super raw axes.
- Parameters:
-
handle the device handle pData pointer where the super raw values of the axes are written
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1301 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_OpMode | ( | DrvContextTypeDef * | handle, |
OP_MODE_t * | axl_opMode | ||
) | [static] |
Get the Operating Mode of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle opMode pointer to the value of Operating mode
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1342 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_Active_Axis | ( | DrvContextTypeDef * | handle, |
ACTIVE_AXIS_t | axl_axis | ||
) | [static] |
Set the active axis of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle axis the active axis to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1448 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_INT1_DRDY | ( | DrvContextTypeDef * | handle, |
INT1_DRDY_CONFIG_t | axl_drdyStatus | ||
) | [static] |
Set DRDY enable/disable of the LIS2DH12 sensor on INT1.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1576 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_OpMode | ( | DrvContextTypeDef * | handle, |
OP_MODE_t | axl_opMode | ||
) | [static] |
Set the Operating Mode of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle opMode the operating mode to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 1376 of file LIS2DH12_ACC_driver_HL.c.
Generated on Tue Jul 12 2022 16:29:50 by
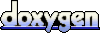