
test sending sensor results over lora radio. Accelerometer and temp/pressure.
Embed:
(wiki syntax)
Show/hide line numbers
LIS2DH12_ACC_driver_HL.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file LIS2DH12_ACC_driver_HL.c 00004 * @author MEMS Application Team 00005 * @brief This file provides a set of high-level functions needed to manage 00006 the LIS2DH12 sensor 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2018 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 00039 #include "LIS2DH12_ACC_driver_HL.h" 00040 00041 /** @addtogroup BSP BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup COMPONENTS COMPONENTS 00046 * @{ 00047 */ 00048 00049 /** @addtogroup LIS2DH12 LIS2DH12 00050 * @{ 00051 */ 00052 00053 /** @addtogroup LIS2DH12_Private_Function_Prototypes Private function prototypes 00054 * @{ 00055 */ 00056 00057 static DrvStatusTypeDef LIS2DH12_Get_Axes_Raw_Data(DrvContextTypeDef *handle, int16_t *pData); 00058 static DrvStatusTypeDef LIS2DH12_Set_ODR_When_Enabled(DrvContextTypeDef *handle, SensorOdr_t odr); 00059 static DrvStatusTypeDef LIS2DH12_Set_ODR_When_Disabled(DrvContextTypeDef *handle, SensorOdr_t odr); 00060 static DrvStatusTypeDef LIS2DH12_Set_ODR_Value_When_Enabled(DrvContextTypeDef *handle, float odr); 00061 static DrvStatusTypeDef LIS2DH12_Set_ODR_Value_When_Disabled(DrvContextTypeDef *handle, float odr); 00062 00063 /** 00064 * @} 00065 */ 00066 00067 /** @addtogroup LIS2DH12_Callable_Private_Function_Prototypes Callable private function prototypes 00068 * @{ 00069 */ 00070 00071 static DrvStatusTypeDef LIS2DH12_Init(DrvContextTypeDef *handle); 00072 static DrvStatusTypeDef LIS2DH12_DeInit(DrvContextTypeDef *handle); 00073 static DrvStatusTypeDef LIS2DH12_Sensor_Enable(DrvContextTypeDef *handle); 00074 static DrvStatusTypeDef LIS2DH12_Sensor_Disable(DrvContextTypeDef *handle); 00075 static DrvStatusTypeDef LIS2DH12_Get_WhoAmI(DrvContextTypeDef *handle, uint8_t *who_am_i); 00076 static DrvStatusTypeDef LIS2DH12_Check_WhoAmI(DrvContextTypeDef *handle); 00077 static DrvStatusTypeDef LIS2DH12_Get_Axes(DrvContextTypeDef *handle, SensorAxes_t *acceleration); 00078 static DrvStatusTypeDef LIS2DH12_Get_AxesRaw(DrvContextTypeDef *handle, SensorAxesRaw_t *value); 00079 static DrvStatusTypeDef LIS2DH12_Get_Sensitivity(DrvContextTypeDef *handle, float *sensitivity); 00080 static DrvStatusTypeDef LIS2DH12_Get_ODR(DrvContextTypeDef *handle, float *odr); 00081 static DrvStatusTypeDef LIS2DH12_Set_ODR(DrvContextTypeDef *handle, SensorOdr_t odr); 00082 static DrvStatusTypeDef LIS2DH12_Set_ODR_Value(DrvContextTypeDef *handle, float odr); 00083 static DrvStatusTypeDef LIS2DH12_Get_FS(DrvContextTypeDef *handle, float *fullScale); 00084 static DrvStatusTypeDef LIS2DH12_Set_FS(DrvContextTypeDef *handle, SensorFs_t fs); 00085 static DrvStatusTypeDef LIS2DH12_Set_FS_Value(DrvContextTypeDef *handle, float fullScale); 00086 static DrvStatusTypeDef LIS2DH12_Get_Axes_Status(DrvContextTypeDef *handle, uint8_t *xyz_enabled); 00087 static DrvStatusTypeDef LIS2DH12_Set_Axes_Status(DrvContextTypeDef *handle, uint8_t *enable_xyz); 00088 static DrvStatusTypeDef LIS2DH12_Read_Reg(DrvContextTypeDef *handle, uint8_t reg, uint8_t *data); 00089 static DrvStatusTypeDef LIS2DH12_Write_Reg(DrvContextTypeDef *handle, uint8_t reg, uint8_t data); 00090 static DrvStatusTypeDef LIS2DH12_Get_DRDY_Status(DrvContextTypeDef *handle, uint8_t *status); 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /** @addtogroup LIS2DH12_Callable_Private_Function_Ext_Prototypes Callable private function for extended features prototypes 00097 * @{ 00098 */ 00099 00100 static DrvStatusTypeDef LIS2DH12_FIFO_Get_Overrun_Status(DrvContextTypeDef *handle, uint8_t *status); 00101 static DrvStatusTypeDef LIS2DH12_FIFO_Get_Num_Of_Samples(DrvContextTypeDef *handle, uint16_t *nSamples); 00102 static DrvStatusTypeDef LIS2DH12_FIFO_Set_Mode(DrvContextTypeDef *handle, uint8_t mode); 00103 static DrvStatusTypeDef LIS2DH12_FIFO_Set_INT1_FIFO_Overrun(DrvContextTypeDef *handle, uint8_t status); 00104 static DrvStatusTypeDef LIS2DH12_Get_AxesSuperRaw(DrvContextTypeDef *handle, int16_t *pData, ACTIVE_AXIS_t axl_axis); 00105 static DrvStatusTypeDef LIS2DH12_Get_OpMode(DrvContextTypeDef *handle, OP_MODE_t *axl_opMode); 00106 static DrvStatusTypeDef LIS2DH12_Set_OpMode(DrvContextTypeDef *handle, OP_MODE_t axl_opMode); 00107 static DrvStatusTypeDef LIS2DH12_Get_Active_Axis(DrvContextTypeDef *handle, ACTIVE_AXIS_t *axl_axis); 00108 static DrvStatusTypeDef LIS2DH12_Set_Active_Axis(DrvContextTypeDef *handle, ACTIVE_AXIS_t axl_axis); 00109 static DrvStatusTypeDef LIS2DH12_Enable_HP_Filter(DrvContextTypeDef *handle); 00110 static DrvStatusTypeDef LIS2DH12_Disable_HP_Filter(DrvContextTypeDef *handle); 00111 static DrvStatusTypeDef LIS2DH12_ClearDRDY(DrvContextTypeDef *handle, ACTIVE_AXIS_t axl_axis); 00112 static DrvStatusTypeDef LIS2DH12_Set_INT1_DRDY(DrvContextTypeDef *handle, INT1_DRDY_CONFIG_t axl_drdyStatus); 00113 00114 00115 /** 00116 * @} 00117 */ 00118 00119 /** @addtogroup LIS2DH12_Public_Variables Public variables 00120 * @{ 00121 */ 00122 00123 /** 00124 * @brief LIS2DH12 accelero extended features driver internal structure 00125 */ 00126 LIS2DH12_ExtDrv_t LIS2DH12_ExtDrv = 00127 { 00128 LIS2DH12_FIFO_Get_Overrun_Status, 00129 LIS2DH12_FIFO_Get_Num_Of_Samples, 00130 LIS2DH12_FIFO_Set_Mode, 00131 LIS2DH12_FIFO_Set_INT1_FIFO_Overrun, 00132 LIS2DH12_Get_AxesSuperRaw, 00133 LIS2DH12_Get_OpMode, 00134 LIS2DH12_Set_OpMode, 00135 LIS2DH12_Get_Active_Axis, 00136 LIS2DH12_Set_Active_Axis, 00137 LIS2DH12_Enable_HP_Filter, 00138 LIS2DH12_Disable_HP_Filter, 00139 LIS2DH12_ClearDRDY, 00140 LIS2DH12_Set_INT1_DRDY 00141 }; 00142 00143 /** 00144 * @brief LIS2DH12 accelero driver structure 00145 */ 00146 ACCELERO_Drv_t LIS2DH12_Drv = 00147 { 00148 LIS2DH12_Init, 00149 LIS2DH12_DeInit, 00150 LIS2DH12_Sensor_Enable, 00151 LIS2DH12_Sensor_Disable, 00152 LIS2DH12_Get_WhoAmI, 00153 LIS2DH12_Check_WhoAmI, 00154 LIS2DH12_Get_Axes, 00155 LIS2DH12_Get_AxesRaw, 00156 LIS2DH12_Get_Sensitivity, 00157 LIS2DH12_Get_ODR, 00158 LIS2DH12_Set_ODR, 00159 LIS2DH12_Set_ODR_Value, 00160 LIS2DH12_Get_FS, 00161 LIS2DH12_Set_FS, 00162 LIS2DH12_Set_FS_Value, 00163 LIS2DH12_Get_Axes_Status, 00164 LIS2DH12_Set_Axes_Status, 00165 LIS2DH12_Read_Reg, 00166 LIS2DH12_Write_Reg, 00167 LIS2DH12_Get_DRDY_Status 00168 }; 00169 00170 /** 00171 * @} 00172 */ 00173 00174 /** @addtogroup LIS2DH12_Callable_Private_Functions Callable private functions 00175 * @{ 00176 */ 00177 00178 /** 00179 * @brief Initialize the LIS2DH12 sensor 00180 * @param handle the device handle 00181 * @retval COMPONENT_OK in case of success 00182 * @retval COMPONENT_ERROR in case of failure 00183 */ 00184 static DrvStatusTypeDef LIS2DH12_Init(DrvContextTypeDef *handle) 00185 { 00186 uint8_t axes_status[] = { 1, 1, 1 }; 00187 00188 if (LIS2DH12_Check_WhoAmI(handle) == COMPONENT_ERROR) 00189 { 00190 return COMPONENT_ERROR; 00191 } 00192 00193 /* Enable BDU. */ 00194 if (LIS2DH12_SetBDU((void *)handle, LIS2DH12_ENABLE) == MEMS_ERROR) 00195 { 00196 return COMPONENT_ERROR; 00197 } 00198 00199 /* FIFO mode selection - FIFO disable. */ 00200 if (LIS2DH12_FIFOModeEnable((void *)handle, LIS2DH12_FIFO_DISABLE) == MEMS_ERROR) 00201 { 00202 return COMPONENT_ERROR; 00203 } 00204 00205 /* Output data rate selection - power down. */ 00206 if (LIS2DH12_SetODR((void *)handle, LIS2DH12_ODR_POWER_DOWN) == MEMS_ERROR) 00207 { 00208 return COMPONENT_ERROR; 00209 } 00210 00211 /* Set default power mode - High resolution. */ 00212 if (LIS2DH12_SetMode((void *)handle, LIS2DH12_HIGH_RES) == MEMS_ERROR) 00213 { 00214 return COMPONENT_ERROR; 00215 } 00216 00217 /* Select default output data rate. */ 00218 if (LIS2DH12_Set_ODR_When_Disabled(handle, ODR_MID_HIGH) == COMPONENT_ERROR) 00219 { 00220 return COMPONENT_ERROR; 00221 } 00222 00223 /* Full scale selection. */ 00224 if (LIS2DH12_Set_FS(handle, FS_LOW) == COMPONENT_ERROR) 00225 { 00226 return COMPONENT_ERROR; 00227 } 00228 00229 /* Enable axes. */ 00230 if (LIS2DH12_Set_Axes_Status(handle, axes_status) == COMPONENT_ERROR) 00231 { 00232 return COMPONENT_ERROR; 00233 } 00234 00235 handle->isInitialized = 1; 00236 00237 return COMPONENT_OK; 00238 } 00239 00240 /** 00241 * @brief Deinitialize the LIS2DH12 sensor 00242 * @param handle the device handle 00243 * @retval COMPONENT_OK in case of success 00244 * @retval COMPONENT_ERROR in case of failure 00245 */ 00246 static DrvStatusTypeDef LIS2DH12_DeInit(DrvContextTypeDef *handle) 00247 { 00248 ACCELERO_Data_t *pData = (ACCELERO_Data_t *)handle->pData; 00249 LIS2DH12_Data_t *pComponentData = (LIS2DH12_Data_t *)pData->pComponentData; 00250 00251 if (LIS2DH12_Check_WhoAmI(handle) == COMPONENT_ERROR) 00252 { 00253 return COMPONENT_ERROR; 00254 } 00255 00256 /* Disable the component */ 00257 if (LIS2DH12_Sensor_Disable(handle) == COMPONENT_ERROR) 00258 { 00259 return COMPONENT_ERROR; 00260 } 00261 00262 /* Reset previous output data rate. */ 00263 pComponentData->Previous_ODR = 0.0f; 00264 00265 handle->isInitialized = 0; 00266 00267 return COMPONENT_OK; 00268 } 00269 00270 /** 00271 * @brief Enable the LIS2DH12 sensor 00272 * @param handle the device handle 00273 * @retval COMPONENT_OK in case of success 00274 * @retval COMPONENT_ERROR in case of failure 00275 */ 00276 static DrvStatusTypeDef LIS2DH12_Sensor_Enable(DrvContextTypeDef *handle) 00277 { 00278 ACCELERO_Data_t *pData = (ACCELERO_Data_t *)handle->pData; 00279 LIS2DH12_Data_t *pComponentData = (LIS2DH12_Data_t *)pData->pComponentData; 00280 00281 /* Check if the component is already enabled */ 00282 if (handle->isEnabled == 1) 00283 { 00284 return COMPONENT_OK; 00285 } 00286 00287 /* Output data rate selection. */ 00288 if (LIS2DH12_Set_ODR_Value_When_Enabled(handle, pComponentData->Previous_ODR) == COMPONENT_ERROR) 00289 { 00290 return COMPONENT_ERROR; 00291 } 00292 00293 handle->isEnabled = 1; 00294 00295 return COMPONENT_OK; 00296 } 00297 00298 /** 00299 * @brief Disable the LIS2DH12 sensor 00300 * @param handle the device handle 00301 * @retval COMPONENT_OK in case of success 00302 * @retval COMPONENT_ERROR in case of failure 00303 */ 00304 static DrvStatusTypeDef LIS2DH12_Sensor_Disable(DrvContextTypeDef *handle) 00305 { 00306 ACCELERO_Data_t *pData = (ACCELERO_Data_t *)handle->pData; 00307 LIS2DH12_Data_t *pComponentData = (LIS2DH12_Data_t *)pData->pComponentData; 00308 00309 /* Check if the component is already disabled */ 00310 if (handle->isEnabled == 0) 00311 { 00312 return COMPONENT_OK; 00313 } 00314 00315 /* Store actual output data rate. */ 00316 if (LIS2DH12_Get_ODR(handle, &(pComponentData->Previous_ODR)) == COMPONENT_ERROR) 00317 { 00318 return COMPONENT_ERROR; 00319 } 00320 00321 /* Output data rate selection - power down. */ 00322 if (LIS2DH12_SetODR((void *)handle, LIS2DH12_ODR_POWER_DOWN) == MEMS_ERROR) 00323 { 00324 return COMPONENT_ERROR; 00325 } 00326 00327 handle->isEnabled = 0; 00328 00329 return COMPONENT_OK; 00330 } 00331 00332 /** 00333 * @brief Get the WHO_AM_I ID of the LIS2DH12 sensor 00334 * @param handle the device handle 00335 * @param who_am_i pointer to the value of WHO_AM_I register 00336 * @retval COMPONENT_OK in case of success 00337 * @retval COMPONENT_ERROR in case of failure 00338 */ 00339 static DrvStatusTypeDef LIS2DH12_Get_WhoAmI(DrvContextTypeDef *handle, uint8_t *who_am_i) 00340 { 00341 /* Read WHO AM I register */ 00342 if (LIS2DH12_GetWHO_AM_I((void *)handle, (uint8_t *)who_am_i) == MEMS_ERROR) 00343 { 00344 return COMPONENT_ERROR; 00345 } 00346 00347 return COMPONENT_OK; 00348 } 00349 00350 /** 00351 * @brief Check the WHO_AM_I ID of the LIS2DH12 sensor 00352 * @param handle the device handle 00353 * @retval COMPONENT_OK in case of success 00354 * @retval COMPONENT_ERROR in case of failure 00355 */ 00356 static DrvStatusTypeDef LIS2DH12_Check_WhoAmI(DrvContextTypeDef *handle) 00357 { 00358 uint8_t who_am_i = 0x00; 00359 00360 if (LIS2DH12_Get_WhoAmI(handle, &who_am_i) == COMPONENT_ERROR) 00361 { 00362 return COMPONENT_ERROR; 00363 } 00364 00365 if (who_am_i != handle->who_am_i) 00366 { 00367 return COMPONENT_ERROR; 00368 } 00369 00370 return COMPONENT_OK; 00371 } 00372 00373 /** 00374 * @brief Get the LIS2DH12 accelerometer sensor axes 00375 * @param handle the device handle 00376 * @param acceleration pointer to where acceleration data write to 00377 * @retval COMPONENT_OK in case of success 00378 * @retval COMPONENT_ERROR in case of failure 00379 */ 00380 static DrvStatusTypeDef LIS2DH12_Get_Axes(DrvContextTypeDef *handle, SensorAxes_t *acceleration) 00381 { 00382 int16_t dataRaw[3]; 00383 float sensitivity = 0; 00384 00385 /* Read raw data from LIS2DH12 output register. */ 00386 if (LIS2DH12_Get_Axes_Raw_Data(handle, dataRaw) == COMPONENT_ERROR) 00387 { 00388 return COMPONENT_ERROR; 00389 } 00390 00391 /* Get LIS2DH12 actual sensitivity. */ 00392 if (LIS2DH12_Get_Sensitivity(handle, &sensitivity) == COMPONENT_ERROR) 00393 { 00394 return COMPONENT_ERROR; 00395 } 00396 00397 /* Calculate the data. */ 00398 acceleration->AXIS_X = (int32_t)(dataRaw[0] * sensitivity); 00399 acceleration->AXIS_Y = (int32_t)(dataRaw[1] * sensitivity); 00400 acceleration->AXIS_Z = (int32_t)(dataRaw[2] * sensitivity); 00401 00402 return COMPONENT_OK; 00403 } 00404 00405 /** 00406 * @brief Get the LIS2DH12 accelerometer sensor raw axes 00407 * @param handle the device handle 00408 * @param acceleration_raw pointer to where acceleration raw data write to 00409 * @retval COMPONENT_OK in case of success 00410 * @retval COMPONENT_ERROR in case of failure 00411 */ 00412 static DrvStatusTypeDef LIS2DH12_Get_AxesRaw(DrvContextTypeDef *handle, SensorAxesRaw_t *value) 00413 { 00414 int16_t dataRaw[3]; 00415 00416 /* Read raw data from LIS2DH12 output register. */ 00417 if (LIS2DH12_Get_Axes_Raw_Data(handle, dataRaw) == COMPONENT_ERROR) 00418 { 00419 return COMPONENT_ERROR; 00420 } 00421 00422 /* Set the raw data. */ 00423 value->AXIS_X = dataRaw[0]; 00424 value->AXIS_Y = dataRaw[1]; 00425 value->AXIS_Z = dataRaw[2]; 00426 00427 return COMPONENT_OK; 00428 } 00429 00430 /** 00431 * @brief Get the LIS2DH12 accelerometer sensor sensitivity 00432 * @param handle the device handle 00433 * @param sensitivity pointer to where sensitivity write to 00434 * @retval COMPONENT_OK in case of success 00435 * @retval COMPONENT_ERROR in case of failure 00436 */ 00437 static DrvStatusTypeDef LIS2DH12_Get_Sensitivity(DrvContextTypeDef *handle, float *sensitivity) 00438 { 00439 LIS2DH12_Mode_t mode; 00440 LIS2DH12_Fullscale_t fullScale; 00441 00442 /* Read actual power mode selection from sensor. */ 00443 if (LIS2DH12_GetMode((void *)handle, &mode) == MEMS_ERROR) 00444 { 00445 return COMPONENT_ERROR; 00446 } 00447 00448 /* Read actual full scale selection from sensor. */ 00449 if (LIS2DH12_GetFullScale((void *)handle, &fullScale) == MEMS_ERROR) 00450 { 00451 return COMPONENT_ERROR; 00452 } 00453 00454 /* Store the sensitivity based on actual operating mode and full scale. */ 00455 switch (mode) 00456 { 00457 00458 case LIS2DH12_HIGH_RES: 00459 switch (fullScale) 00460 { 00461 00462 case LIS2DH12_FULLSCALE_2: 00463 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_2G_HI_RES; 00464 break; 00465 00466 case LIS2DH12_FULLSCALE_4: 00467 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_4G_HI_RES; 00468 break; 00469 00470 case LIS2DH12_FULLSCALE_8: 00471 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_8G_HI_RES; 00472 break; 00473 00474 case LIS2DH12_FULLSCALE_16: 00475 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_16G_HI_RES; 00476 break; 00477 00478 default: 00479 *sensitivity = -1.0f; 00480 return COMPONENT_ERROR; 00481 } 00482 00483 break; 00484 00485 case LIS2DH12_NORMAL: 00486 switch (fullScale) 00487 { 00488 00489 case LIS2DH12_FULLSCALE_2: 00490 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_2G_NORMAL; 00491 break; 00492 00493 case LIS2DH12_FULLSCALE_4: 00494 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_4G_NORMAL; 00495 break; 00496 00497 case LIS2DH12_FULLSCALE_8: 00498 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_8G_NORMAL; 00499 break; 00500 00501 case LIS2DH12_FULLSCALE_16: 00502 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_16G_NORMAL; 00503 break; 00504 00505 default: 00506 *sensitivity = -1.0f; 00507 return COMPONENT_ERROR; 00508 } 00509 00510 break; 00511 00512 case LIS2DH12_LOW_POWER: 00513 switch (fullScale) 00514 { 00515 00516 case LIS2DH12_FULLSCALE_2: 00517 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_2G_LO_POW; 00518 break; 00519 00520 case LIS2DH12_FULLSCALE_4: 00521 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_4G_LO_POW; 00522 break; 00523 00524 case LIS2DH12_FULLSCALE_8: 00525 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_8G_LO_POW; 00526 break; 00527 00528 case LIS2DH12_FULLSCALE_16: 00529 *sensitivity = (float)LIS2DH12_ACC_SENSITIVITY_FOR_FS_16G_LO_POW; 00530 break; 00531 00532 default: 00533 *sensitivity = -1.0f; 00534 return COMPONENT_ERROR; 00535 } 00536 00537 break; 00538 00539 default: 00540 *sensitivity = -1.0f; 00541 return COMPONENT_ERROR; 00542 } 00543 00544 return COMPONENT_OK; 00545 } 00546 00547 /** 00548 * @brief Get the LIS2DH12 accelerometer sensor output data rate 00549 * @param handle the device handle 00550 * @param odr pointer to where output data rate write to 00551 * @retval COMPONENT_OK in case of success 00552 * @retval COMPONENT_ERROR in case of failure 00553 */ 00554 static DrvStatusTypeDef LIS2DH12_Get_ODR(DrvContextTypeDef *handle, float *odr) 00555 { 00556 LIS2DH12_Mode_t mode; 00557 LIS2DH12_ODR_t odr_low_level; 00558 00559 /* Read actual power mode selection from sensor. */ 00560 if (LIS2DH12_GetMode((void *)handle, &mode) == MEMS_ERROR) 00561 { 00562 return COMPONENT_ERROR; 00563 } 00564 00565 /* Read actual output data rate selection from sensor. */ 00566 if (LIS2DH12_GetODR((void *)handle, &odr_low_level) == MEMS_ERROR) 00567 { 00568 return COMPONENT_ERROR; 00569 } 00570 00571 switch (odr_low_level) 00572 { 00573 00574 case LIS2DH12_ODR_POWER_DOWN: 00575 *odr = 0.0f; 00576 break; 00577 00578 case LIS2DH12_ODR_1Hz: 00579 *odr = 1.0f; 00580 break; 00581 00582 case LIS2DH12_ODR_10Hz: 00583 *odr = 10.0f; 00584 break; 00585 00586 case LIS2DH12_ODR_25Hz: 00587 *odr = 25.0f; 00588 break; 00589 00590 case LIS2DH12_ODR_50Hz: 00591 *odr = 50.0f; 00592 break; 00593 00594 case LIS2DH12_ODR_100Hz: 00595 *odr = 100.0f; 00596 break; 00597 00598 case LIS2DH12_ODR_200Hz: 00599 *odr = 200.0f; 00600 break; 00601 00602 case LIS2DH12_ODR_400Hz: 00603 *odr = 400.0f; 00604 break; 00605 00606 case LIS2DH12_ODR_1620Hz_LP: 00607 switch (mode) 00608 { 00609 00610 case LIS2DH12_LOW_POWER: 00611 *odr = 1620.0f; 00612 break; 00613 00614 default: 00615 *odr = -1.0f; 00616 return COMPONENT_ERROR; 00617 } 00618 00619 case LIS2DH12_ODR_1344Hz_NP_5367HZ_LP: 00620 switch (mode) 00621 { 00622 00623 case LIS2DH12_NORMAL: 00624 case LIS2DH12_HIGH_RES: 00625 *odr = 1344.0f; 00626 break; 00627 00628 case LIS2DH12_LOW_POWER: 00629 *odr = 5376.0f; 00630 break; 00631 00632 default: 00633 *odr = -1.0f; 00634 return COMPONENT_ERROR; 00635 } 00636 00637 default: 00638 *odr = -1.0f; 00639 return COMPONENT_ERROR; 00640 } 00641 00642 return COMPONENT_OK; 00643 } 00644 00645 /** 00646 * @brief Set the LIS2DH12 accelerometer sensor output data rate 00647 * @param handle the device handle 00648 * @param odr the functional output data rate to be set 00649 * @retval COMPONENT_OK in case of success 00650 * @retval COMPONENT_ERROR in case of failure 00651 */ 00652 static DrvStatusTypeDef LIS2DH12_Set_ODR(DrvContextTypeDef *handle, SensorOdr_t odr) 00653 { 00654 if (handle->isEnabled == 1) 00655 { 00656 if (LIS2DH12_Set_ODR_When_Enabled(handle, odr) == COMPONENT_ERROR) 00657 { 00658 return COMPONENT_ERROR; 00659 } 00660 } 00661 else 00662 { 00663 if (LIS2DH12_Set_ODR_When_Disabled(handle, odr) == COMPONENT_ERROR) 00664 { 00665 return COMPONENT_ERROR; 00666 } 00667 } 00668 00669 return COMPONENT_OK; 00670 } 00671 00672 /** 00673 * @brief Set the LIS2DH12 accelerometer sensor output data rate 00674 * @param handle the device handle 00675 * @param odr the output data rate value to be set 00676 * @retval COMPONENT_OK in case of success 00677 * @retval COMPONENT_ERROR in case of failure 00678 */ 00679 static DrvStatusTypeDef LIS2DH12_Set_ODR_Value(DrvContextTypeDef *handle, float odr) 00680 { 00681 if (handle->isEnabled == 1) 00682 { 00683 if (LIS2DH12_Set_ODR_Value_When_Enabled(handle, odr) == COMPONENT_ERROR) 00684 { 00685 return COMPONENT_ERROR; 00686 } 00687 } 00688 else 00689 { 00690 if (LIS2DH12_Set_ODR_Value_When_Disabled(handle, odr) == COMPONENT_ERROR) 00691 { 00692 return COMPONENT_ERROR; 00693 } 00694 } 00695 00696 return COMPONENT_OK; 00697 } 00698 00699 /** 00700 * @brief Get the LIS2DH12 accelerometer sensor full scale 00701 * @param handle the device handle 00702 * @param fullScale pointer to where full scale write to 00703 * @retval COMPONENT_OK in case of success 00704 * @retval COMPONENT_ERROR in case of failure 00705 */ 00706 static DrvStatusTypeDef LIS2DH12_Get_FS(DrvContextTypeDef *handle, float *fullScale) 00707 { 00708 LIS2DH12_Fullscale_t fs_low_level; 00709 00710 if (LIS2DH12_GetFullScale((void *)handle, &fs_low_level) == MEMS_ERROR) 00711 { 00712 return COMPONENT_ERROR; 00713 } 00714 00715 switch (fs_low_level) 00716 { 00717 case LIS2DH12_FULLSCALE_2: 00718 *fullScale = 2.0f; 00719 break; 00720 case LIS2DH12_FULLSCALE_4: 00721 *fullScale = 4.0f; 00722 break; 00723 case LIS2DH12_FULLSCALE_8: 00724 *fullScale = 8.0f; 00725 break; 00726 case LIS2DH12_FULLSCALE_16: 00727 *fullScale = 16.0f; 00728 break; 00729 default: 00730 *fullScale = -1.0f; 00731 return COMPONENT_ERROR; 00732 } 00733 00734 return COMPONENT_OK; 00735 } 00736 00737 /** 00738 * @brief Set the LIS2DH12 accelerometer sensor full scale 00739 * @param handle the device handle 00740 * @param fullScale the functional full scale to be set 00741 * @retval COMPONENT_OK in case of success 00742 * @retval COMPONENT_ERROR in case of failure 00743 */ 00744 static DrvStatusTypeDef LIS2DH12_Set_FS(DrvContextTypeDef *handle, SensorFs_t fullScale) 00745 { 00746 LIS2DH12_Fullscale_t new_fs; 00747 00748 switch (fullScale) 00749 { 00750 00751 case FS_LOW: 00752 new_fs = LIS2DH12_FULLSCALE_2; 00753 break; 00754 00755 case FS_MID_LOW: 00756 case FS_MID: 00757 new_fs = LIS2DH12_FULLSCALE_4; 00758 break; 00759 00760 case FS_MID_HIGH: 00761 new_fs = LIS2DH12_FULLSCALE_8; 00762 break; 00763 00764 case FS_HIGH: 00765 new_fs = LIS2DH12_FULLSCALE_16; 00766 break; 00767 00768 default: 00769 return COMPONENT_ERROR; 00770 } 00771 00772 if (LIS2DH12_SetFullScale((void *)handle, new_fs) == MEMS_ERROR) 00773 { 00774 return COMPONENT_ERROR; 00775 } 00776 00777 return COMPONENT_OK; 00778 } 00779 00780 /** 00781 * @brief Set the LIS2DH12 accelerometer sensor full scale 00782 * @param handle the device handle 00783 * @param fullScale the full scale value to be set 00784 * @retval COMPONENT_OK in case of success 00785 * @retval COMPONENT_ERROR in case of failure 00786 */ 00787 static DrvStatusTypeDef LIS2DH12_Set_FS_Value(DrvContextTypeDef *handle, float fullScale) 00788 { 00789 LIS2DH12_Fullscale_t new_fs; 00790 00791 new_fs = (fullScale <= 2.0f) ? LIS2DH12_FULLSCALE_2 00792 : (fullScale <= 4.0f) ? LIS2DH12_FULLSCALE_4 00793 : (fullScale <= 8.0f) ? LIS2DH12_FULLSCALE_8 00794 : LIS2DH12_FULLSCALE_16; 00795 00796 if (LIS2DH12_SetFullScale((void *)handle, new_fs) == MEMS_ERROR) 00797 { 00798 return COMPONENT_ERROR; 00799 } 00800 00801 return COMPONENT_OK; 00802 } 00803 00804 /** 00805 * @brief Get the LIS2DH12 accelerometer sensor axes enabled/disabled status 00806 * @param handle the device handle 00807 * @param xyz_enabled pointer to where the vector of the axes enabled/disabled status write to 00808 * @retval COMPONENT_OK in case of success 00809 * @retval COMPONENT_ERROR in case of failure 00810 */ 00811 static DrvStatusTypeDef LIS2DH12_Get_Axes_Status(DrvContextTypeDef *handle, uint8_t *xyz_enabled) 00812 { 00813 LIS2DH12_AxesEnabled_t axesEnabled; 00814 00815 if (LIS2DH12_GetAxesEnabled((void *)handle, &axesEnabled) == MEMS_ERROR) 00816 { 00817 return COMPONENT_ERROR; 00818 } 00819 00820 xyz_enabled[0] = (axesEnabled & LIS2DH12_X_ENABLE) ? 1 : 0; 00821 xyz_enabled[1] = (axesEnabled & LIS2DH12_Y_ENABLE) ? 1 : 0; 00822 xyz_enabled[2] = (axesEnabled & LIS2DH12_Z_ENABLE) ? 1 : 0; 00823 00824 return COMPONENT_OK; 00825 } 00826 00827 /** 00828 * @brief Set the LIS2DH12 accelerometer sensor axes enabled/disabled 00829 * @param handle the device handle 00830 * @param enable_xyz vector of the axes enabled/disabled status 00831 * @retval COMPONENT_OK in case of success 00832 * @retval COMPONENT_ERROR in case of failure 00833 */ 00834 static DrvStatusTypeDef LIS2DH12_Set_Axes_Status(DrvContextTypeDef *handle, uint8_t *enable_xyz) 00835 { 00836 LIS2DH12_AxesEnabled_t axesEnable = 0x00; 00837 00838 axesEnable |= (enable_xyz[0] == 1) ? LIS2DH12_X_ENABLE : LIS2DH12_X_DISABLE; 00839 axesEnable |= (enable_xyz[1] == 1) ? LIS2DH12_Y_ENABLE : LIS2DH12_Y_DISABLE; 00840 axesEnable |= (enable_xyz[2] == 1) ? LIS2DH12_Z_ENABLE : LIS2DH12_Z_DISABLE; 00841 00842 if (LIS2DH12_SetAxesEnabled((void *)handle, axesEnable) == MEMS_ERROR) 00843 { 00844 return COMPONENT_ERROR; 00845 } 00846 00847 return COMPONENT_OK; 00848 } 00849 00850 /** 00851 * @brief Read the data from register 00852 * @param handle the device handle 00853 * @param reg register address 00854 * @param data register data 00855 * @retval COMPONENT_OK in case of success 00856 * @retval COMPONENT_ERROR in case of failure 00857 */ 00858 static DrvStatusTypeDef LIS2DH12_Read_Reg(DrvContextTypeDef *handle, uint8_t reg, uint8_t *data) 00859 { 00860 00861 if (LIS2DH12_ACC_ReadReg((void *)handle, reg, data, 1) == MEMS_ERROR) 00862 { 00863 return COMPONENT_ERROR; 00864 } 00865 00866 return COMPONENT_OK; 00867 } 00868 00869 /** 00870 * @brief Write the data to register 00871 * @param handle the device handle 00872 * @param reg register address 00873 * @param data register data 00874 * @retval COMPONENT_OK in case of success 00875 * @retval COMPONENT_ERROR in case of failure 00876 */ 00877 static DrvStatusTypeDef LIS2DH12_Write_Reg(DrvContextTypeDef *handle, uint8_t reg, uint8_t data) 00878 { 00879 00880 if (LIS2DH12_ACC_WriteReg((void *)handle, reg, &data, 1) == MEMS_ERROR) 00881 { 00882 return COMPONENT_ERROR; 00883 } 00884 00885 return COMPONENT_OK; 00886 } 00887 00888 /** 00889 * @brief Get data ready status 00890 * @param handle the device handle 00891 * @param status the data ready status 00892 * @retval COMPONENT_OK in case of success 00893 * @retval COMPONENT_ERROR in case of failure 00894 */ 00895 static DrvStatusTypeDef LIS2DH12_Get_DRDY_Status(DrvContextTypeDef *handle, uint8_t *status) 00896 { 00897 00898 if (LIS2DH12_GetStatusBit((void *)handle, LIS2DH12_STATUS_REG_ZYXDA, status) == MEMS_ERROR) 00899 { 00900 return COMPONENT_ERROR; 00901 } 00902 00903 return COMPONENT_OK; 00904 } 00905 00906 /** 00907 * @} 00908 */ 00909 00910 /** @addtogroup LIS2DH12_Private_Functions Private functions 00911 * @{ 00912 */ 00913 00914 /** 00915 * @brief Get the LIS2DH12 accelerometer sensor raw axes data 00916 * @param handle the device handle 00917 * @param pData pointer to where the raw values of the axes write to 00918 * @retval COMPONENT_OK in case of success 00919 * @retval COMPONENT_ERROR in case of failure 00920 */ 00921 static DrvStatusTypeDef LIS2DH12_Get_Axes_Raw_Data(DrvContextTypeDef *handle, int16_t *pData) 00922 { 00923 uint8_t regValue[6] = { 0, 0, 0, 0, 0, 0 }; 00924 LIS2DH12_Mode_t mode; 00925 00926 /* Read output registers from LIS2DH12_OUT_X_L to LIS2DH12_OUT_Z_H. */ 00927 if (LIS2DH12_GetAccAxesRaw((void *)handle, (u8_t *)regValue) == MEMS_ERROR) 00928 { 00929 return COMPONENT_ERROR; 00930 } 00931 00932 pData[0] = ((((int16_t)regValue[1]) << 8) + (int16_t)regValue[0]); 00933 pData[1] = ((((int16_t)regValue[3]) << 8) + (int16_t)regValue[2]); 00934 pData[2] = ((((int16_t)regValue[5]) << 8) + (int16_t)regValue[4]); 00935 00936 /* Read actual power mode selection from sensor. */ 00937 if (LIS2DH12_GetMode((void *)handle, &mode) == MEMS_ERROR) 00938 { 00939 return COMPONENT_ERROR; 00940 } 00941 00942 /* Select raw data format according to power mode selected. */ 00943 switch (mode) 00944 { 00945 00946 case LIS2DH12_HIGH_RES: /* 12 bits left justified */ 00947 pData[0] >>= 4; 00948 pData[1] >>= 4; 00949 pData[2] >>= 4; 00950 break; 00951 00952 case LIS2DH12_NORMAL: /* 10 bits left justified */ 00953 pData[0] >>= 6; 00954 pData[1] >>= 6; 00955 pData[2] >>= 6; 00956 break; 00957 00958 case LIS2DH12_LOW_POWER: /* 8 bits left justified */ 00959 pData[0] >>= 8; 00960 pData[1] >>= 8; 00961 pData[2] >>= 8; 00962 break; 00963 00964 default: 00965 return COMPONENT_ERROR; 00966 } 00967 00968 return COMPONENT_OK; 00969 } 00970 00971 /** 00972 * @brief Set the LIS2DH12 accelerometer sensor output data rate when enabled 00973 * @param handle the device handle 00974 * @param odr the functional output data rate to be set 00975 * @retval COMPONENT_OK in case of success 00976 * @retval COMPONENT_ERROR in case of failure 00977 */ 00978 static DrvStatusTypeDef LIS2DH12_Set_ODR_When_Enabled(DrvContextTypeDef *handle, SensorOdr_t odr) 00979 { 00980 LIS2DH12_ODR_t new_odr; 00981 00982 switch (odr) 00983 { 00984 00985 case ODR_LOW: 00986 new_odr = LIS2DH12_ODR_10Hz; 00987 break; 00988 00989 case ODR_MID_LOW: 00990 new_odr = LIS2DH12_ODR_25Hz; 00991 break; 00992 00993 case ODR_MID: 00994 new_odr = LIS2DH12_ODR_50Hz; 00995 break; 00996 00997 case ODR_MID_HIGH: 00998 new_odr = LIS2DH12_ODR_100Hz; 00999 break; 01000 01001 case ODR_HIGH: 01002 new_odr = LIS2DH12_ODR_200Hz; 01003 break; 01004 01005 default: 01006 return COMPONENT_ERROR; 01007 } 01008 01009 if (LIS2DH12_SetODR((void *)handle, new_odr) == MEMS_ERROR) 01010 { 01011 return COMPONENT_ERROR; 01012 } 01013 01014 return COMPONENT_OK; 01015 } 01016 01017 /** 01018 * @brief Set the LIS2DH12 accelerometer sensor output data rate when disabled 01019 * @param handle the device handle 01020 * @param odr the functional output data rate to be set 01021 * @retval COMPONENT_OK in case of success 01022 * @retval COMPONENT_ERROR in case of failure 01023 */ 01024 static DrvStatusTypeDef LIS2DH12_Set_ODR_When_Disabled(DrvContextTypeDef *handle, SensorOdr_t odr) 01025 { 01026 ACCELERO_Data_t *pData = (ACCELERO_Data_t *)handle->pData; 01027 LIS2DH12_Data_t *pComponentData = (LIS2DH12_Data_t *)pData->pComponentData; 01028 01029 switch (odr) 01030 { 01031 01032 case ODR_LOW: 01033 pComponentData->Previous_ODR = 10.0f; 01034 break; 01035 01036 case ODR_MID_LOW: 01037 pComponentData->Previous_ODR = 25.0f; 01038 break; 01039 01040 case ODR_MID: 01041 pComponentData->Previous_ODR = 50.0f; 01042 break; 01043 01044 case ODR_MID_HIGH: 01045 pComponentData->Previous_ODR = 100.0f; 01046 break; 01047 01048 case ODR_HIGH: 01049 pComponentData->Previous_ODR = 200.0f; 01050 break; 01051 01052 default: 01053 return COMPONENT_ERROR; 01054 } 01055 01056 return COMPONENT_OK; 01057 } 01058 01059 /** 01060 * @brief Set the LIS2DH12 accelerometer sensor output data rate when enabled 01061 * @param handle the device handle 01062 * @param odr the output data rate value to be set 01063 * @retval COMPONENT_OK in case of success 01064 * @retval COMPONENT_ERROR in case of failure 01065 */ 01066 static DrvStatusTypeDef LIS2DH12_Set_ODR_Value_When_Enabled(DrvContextTypeDef *handle, float odr) 01067 { 01068 LIS2DH12_Mode_t mode; 01069 LIS2DH12_ODR_t new_odr; 01070 01071 /* Read actual power mode selection from sensor. */ 01072 if (LIS2DH12_GetMode((void *)handle, &mode) == MEMS_ERROR) 01073 { 01074 return COMPONENT_ERROR; 01075 } 01076 01077 if (odr <= 400.0f) 01078 { 01079 new_odr = (odr <= 1.0f) ? LIS2DH12_ODR_1Hz 01080 : (odr <= 10.0f) ? LIS2DH12_ODR_10Hz 01081 : (odr <= 25.0f) ? LIS2DH12_ODR_25Hz 01082 : (odr <= 50.0f) ? LIS2DH12_ODR_50Hz 01083 : (odr <= 100.0f) ? LIS2DH12_ODR_100Hz 01084 : (odr <= 200.0f) ? LIS2DH12_ODR_200Hz 01085 : LIS2DH12_ODR_400Hz; 01086 } 01087 01088 else 01089 { 01090 switch (mode) 01091 { 01092 01093 case LIS2DH12_LOW_POWER: 01094 new_odr = (odr <= 1620.0f) ? LIS2DH12_ODR_1620Hz_LP : LIS2DH12_ODR_1344Hz_NP_5367HZ_LP; 01095 break; 01096 01097 case LIS2DH12_NORMAL: 01098 case LIS2DH12_HIGH_RES: 01099 new_odr = LIS2DH12_ODR_1344Hz_NP_5367HZ_LP; 01100 break; 01101 01102 default: 01103 return COMPONENT_ERROR; 01104 } 01105 } 01106 01107 if (LIS2DH12_SetODR((void *)handle, new_odr) == MEMS_ERROR) 01108 { 01109 return COMPONENT_ERROR; 01110 } 01111 01112 return COMPONENT_OK; 01113 } 01114 01115 /** 01116 * @brief Set the LIS2DH12 accelerometer sensor output data rate when disabled 01117 * @param handle the device handle 01118 * @param odr the output data rate value to be set 01119 * @retval COMPONENT_OK in case of success 01120 * @retval COMPONENT_ERROR in case of failure 01121 */ 01122 static DrvStatusTypeDef LIS2DH12_Set_ODR_Value_When_Disabled(DrvContextTypeDef *handle, float odr) 01123 { 01124 ACCELERO_Data_t *pData = (ACCELERO_Data_t *)handle->pData; 01125 LIS2DH12_Data_t *pComponentData = (LIS2DH12_Data_t *)pData->pComponentData; 01126 LIS2DH12_Mode_t mode; 01127 01128 /* Read actual power mode selection from sensor. */ 01129 if (LIS2DH12_GetMode((void *)handle, &mode) == MEMS_ERROR) 01130 { 01131 return COMPONENT_ERROR; 01132 } 01133 01134 if (odr <= 400.0f) 01135 { 01136 pComponentData->Previous_ODR = (odr <= 1.0f) ? 1.0f 01137 : (odr <= 10.0f) ? 10.0f 01138 : (odr <= 25.0f) ? 25.0f 01139 : (odr <= 50.0f) ? 50.0f 01140 : (odr <= 100.0f) ? 100.0f 01141 : (odr <= 200.0f) ? 200.0f 01142 : 400.0f; 01143 } 01144 01145 else 01146 { 01147 switch (mode) 01148 { 01149 01150 case LIS2DH12_LOW_POWER: 01151 pComponentData->Previous_ODR = (odr <= 1620.0f) ? 1620.0f : 5376.0f; 01152 break; 01153 01154 case LIS2DH12_NORMAL: 01155 case LIS2DH12_HIGH_RES: 01156 pComponentData->Previous_ODR = 1344.0f; 01157 break; 01158 01159 default: 01160 return COMPONENT_ERROR; 01161 } 01162 } 01163 01164 return COMPONENT_OK; 01165 } 01166 01167 /** 01168 * @} 01169 */ 01170 01171 /** @addtogroup LIS2DH12_Callable_Private_Functions_Ext Callable private functions for extended features 01172 * @{ 01173 */ 01174 01175 /** 01176 * @brief Get status of FIFO_OVR flag 01177 * @param handle the device handle 01178 * @param *status The pointer where the status of FIFO is stored 01179 * @retval COMPONENT_OK in case of success 01180 * @retval COMPONENT_ERROR in case of failure 01181 */ 01182 static DrvStatusTypeDef LIS2DH12_FIFO_Get_Overrun_Status(DrvContextTypeDef *handle, uint8_t *status) 01183 { 01184 01185 if (LIS2DH12_GetFifoSourceBit(handle, LIS2DH12_FIFO_SRC_OVRUN, status) == MEMS_ERROR) 01186 { 01187 return COMPONENT_ERROR; 01188 } 01189 01190 return COMPONENT_OK; 01191 } 01192 01193 01194 01195 /** 01196 * @brief Get number of unread FIFO samples 01197 * @param handle the device handle 01198 * @param *nSamples Number of unread FIFO samples 01199 * @retval COMPONENT_OK in case of success 01200 * @retval COMPONENT_ERROR in case of failure 01201 */ 01202 static DrvStatusTypeDef LIS2DH12_FIFO_Get_Num_Of_Samples(DrvContextTypeDef *handle, uint16_t *nSamples) 01203 { 01204 01205 uint8_t nSamplesRaw = 0; 01206 01207 if (LIS2DH12_GetFifoSourceFSS(handle, &nSamplesRaw) == MEMS_ERROR) 01208 { 01209 return COMPONENT_ERROR; 01210 } 01211 01212 *nSamples = (uint16_t)nSamplesRaw; 01213 01214 return COMPONENT_OK; 01215 } 01216 01217 01218 01219 /** 01220 * @brief Set FIFO mode 01221 * @param handle the device handle 01222 * @param mode FIFO mode 01223 * @retval COMPONENT_OK in case of success 01224 * @retval COMPONENT_ERROR in case of failure 01225 */ 01226 static DrvStatusTypeDef LIS2DH12_FIFO_Set_Mode(DrvContextTypeDef *handle, uint8_t mode) 01227 { 01228 01229 /* Verify that the passed parameter contains one of the valid values */ 01230 switch ((LIS2DH12_FifoMode_t)mode) 01231 { 01232 case LIS2DH12_FIFO_DISABLE: /* Disable FIFO */ 01233 case LIS2DH12_FIFO_BYPASS_MODE: /* Bypass mode */ 01234 case LIS2DH12_FIFO_MODE: /* FIFO mode */ 01235 case LIS2DH12_FIFO_STREAM_MODE: /* Stream mode */ 01236 case LIS2DH12_FIFO_TRIGGER_MODE: /* Stream to FIFO mode */ 01237 break; 01238 default: 01239 return COMPONENT_ERROR; 01240 } 01241 01242 if (LIS2DH12_FIFOModeEnable(handle, (LIS2DH12_FifoMode_t)mode) == MEMS_ERROR) 01243 { 01244 return COMPONENT_ERROR; 01245 } 01246 01247 return COMPONENT_OK; 01248 } 01249 01250 01251 01252 /** 01253 * @brief Set FIFO_OVR interrupt on INT1 pin 01254 * @param handle the device handle 01255 * @param status FIFO_OVR interrupt on INT1 pin enable/disable 01256 * @retval COMPONENT_OK in case of success 01257 * @retval COMPONENT_ERROR in case of failure 01258 */ 01259 static DrvStatusTypeDef LIS2DH12_FIFO_Set_INT1_FIFO_Overrun(DrvContextTypeDef *handle, uint8_t status) 01260 { 01261 01262 LIS2DH12_IntPinConf_t value = 0; 01263 01264 /* Read current status of register */ 01265 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_CTRL_REG3, &value, 1) == MEMS_ERROR) 01266 { 01267 return COMPONENT_ERROR; 01268 } 01269 01270 /* Set / reset the desired bit */ 01271 if ((LIS2DH12_ACC_State_t)status == LIS2DH12_ENABLE) 01272 { 01273 value |= LIS2DH12_INT1_OVERRUN_ENABLE; 01274 } 01275 else if ((LIS2DH12_ACC_State_t)status == LIS2DH12_DISABLE) 01276 { 01277 value &= ~LIS2DH12_INT1_OVERRUN_ENABLE; 01278 } 01279 else 01280 { 01281 return COMPONENT_ERROR; 01282 } 01283 01284 /* Set new register value */ 01285 if (LIS2DH12_SetInt1Pin(handle, value) == MEMS_ERROR) 01286 { 01287 return COMPONENT_ERROR; 01288 } 01289 01290 return COMPONENT_OK; 01291 } 01292 01293 01294 /** 01295 * @brief Get the LIS2DH12 accelerometer sensor super raw axes 01296 * @param handle the device handle 01297 * @param pData pointer where the super raw values of the axes are written 01298 * @retval COMPONENT_OK in case of success 01299 * @retval COMPONENT_ERROR in case of failure 01300 */ 01301 static DrvStatusTypeDef LIS2DH12_Get_AxesSuperRaw(DrvContextTypeDef *handle, int16_t *pData, ACTIVE_AXIS_t axl_axis) 01302 { 01303 uint8_t regValue[2] = {0, 0}; 01304 01305 switch (axl_axis) 01306 { 01307 case X_AXIS: 01308 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_OUT_X_L, regValue, 2) == MEMS_ERROR) 01309 { 01310 return COMPONENT_ERROR; 01311 } 01312 break; 01313 case Y_AXIS: 01314 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_OUT_Y_L, regValue, 2) == MEMS_ERROR) 01315 { 01316 return COMPONENT_ERROR; 01317 } 01318 break; 01319 case Z_AXIS: 01320 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_OUT_Z_L, regValue, 2) == MEMS_ERROR) 01321 { 01322 return COMPONENT_ERROR; 01323 } 01324 break; 01325 default: 01326 return COMPONENT_ERROR; 01327 } 01328 01329 *pData = ((((int16_t)regValue[1]) << 8) + ((int16_t)regValue[0])); 01330 01331 return COMPONENT_OK; 01332 01333 } 01334 01335 /** 01336 * @brief Get the Operating Mode of the LIS2DH12 sensor 01337 * @param handle the device handle 01338 * @param opMode pointer to the value of Operating mode 01339 * @retval COMPONENT_OK in case of success 01340 * @retval COMPONENT_ERROR in case of failure 01341 */ 01342 static DrvStatusTypeDef LIS2DH12_Get_OpMode(DrvContextTypeDef *handle, OP_MODE_t *axl_opMode) 01343 { 01344 LIS2DH12_Mode_t opMode; 01345 01346 if (LIS2DH12_GetMode((void *)handle, &opMode) == MEMS_ERROR) 01347 { 01348 return COMPONENT_ERROR; 01349 } 01350 01351 switch (opMode) 01352 { 01353 case LIS2DH12_NORMAL: 01354 *axl_opMode = NORMAL_MODE; 01355 break; 01356 case LIS2DH12_HIGH_RES: 01357 *axl_opMode = HIGH_RES_MODE; 01358 break; 01359 case LIS2DH12_LOW_POWER: 01360 *axl_opMode = LOW_PWR_MODE; 01361 break; 01362 default: 01363 return COMPONENT_ERROR; 01364 } 01365 01366 return COMPONENT_OK; 01367 } 01368 01369 /** 01370 * @brief Set the Operating Mode of the LIS2DH12 sensor 01371 * @param handle the device handle 01372 * @param opMode the operating mode to be set 01373 * @retval COMPONENT_OK in case of success 01374 * @retval COMPONENT_ERROR in case of failure 01375 */ 01376 static DrvStatusTypeDef LIS2DH12_Set_OpMode(DrvContextTypeDef *handle, OP_MODE_t axl_opMode) 01377 { 01378 LIS2DH12_Mode_t opMode; 01379 01380 switch (axl_opMode) 01381 { 01382 case NORMAL_MODE: 01383 opMode = LIS2DH12_NORMAL; 01384 break; 01385 case HIGH_RES_MODE: 01386 opMode = LIS2DH12_HIGH_RES; 01387 break; 01388 case LOW_PWR_MODE: 01389 opMode = LIS2DH12_LOW_POWER; 01390 break; 01391 default: 01392 return COMPONENT_ERROR; 01393 } 01394 01395 if (LIS2DH12_SetMode((void *)handle, opMode) == MEMS_ERROR) 01396 { 01397 return COMPONENT_ERROR; 01398 } 01399 01400 return COMPONENT_OK; 01401 } 01402 01403 01404 /** 01405 * @brief Get the active axis of the LIS2DH12 sensor 01406 * @param handle the device handle 01407 * @param axis pointer to the value of active axis 01408 * @retval COMPONENT_OK in case of success 01409 * @retval COMPONENT_ERROR in case of failure 01410 */ 01411 static DrvStatusTypeDef LIS2DH12_Get_Active_Axis(DrvContextTypeDef *handle, ACTIVE_AXIS_t *axl_axis) 01412 { 01413 LIS2DH12_AxesEnabled_t axis; 01414 01415 if (LIS2DH12_GetAxesEnabled((void *)handle, &axis) == MEMS_ERROR) 01416 { 01417 return COMPONENT_ERROR; 01418 } 01419 01420 switch (axis) 01421 { 01422 case LIS2DH12_X_ENABLE: 01423 *axl_axis = X_AXIS; 01424 break; 01425 case LIS2DH12_Y_ENABLE: 01426 *axl_axis = Y_AXIS; 01427 break; 01428 case LIS2DH12_Z_ENABLE: 01429 *axl_axis = Z_AXIS; 01430 break; 01431 case (LIS2DH12_X_ENABLE|LIS2DH12_Y_ENABLE|LIS2DH12_Z_ENABLE): 01432 *axl_axis = ALL_ACTIVE; 01433 break; 01434 default: 01435 return COMPONENT_ERROR; 01436 } 01437 01438 return COMPONENT_OK; 01439 } 01440 01441 /** 01442 * @brief Set the active axis of the LIS2DH12 sensor 01443 * @param handle the device handle 01444 * @param axis the active axis to be set 01445 * @retval COMPONENT_OK in case of success 01446 * @retval COMPONENT_ERROR in case of failure 01447 */ 01448 static DrvStatusTypeDef LIS2DH12_Set_Active_Axis(DrvContextTypeDef *handle, ACTIVE_AXIS_t axl_axis) 01449 { 01450 LIS2DH12_AxesEnabled_t axis; 01451 01452 switch (axl_axis) 01453 { 01454 case X_AXIS: 01455 axis = LIS2DH12_X_ENABLE; 01456 break; 01457 case Y_AXIS: 01458 axis = LIS2DH12_Y_ENABLE; 01459 break; 01460 case Z_AXIS: 01461 axis = LIS2DH12_Z_ENABLE; 01462 break; 01463 case ALL_ACTIVE: 01464 axis = (LIS2DH12_X_ENABLE | LIS2DH12_Y_ENABLE | LIS2DH12_Z_ENABLE); 01465 break; 01466 default: 01467 return COMPONENT_ERROR; 01468 } 01469 01470 if (LIS2DH12_SetAxesEnabled((void *)handle, axis) == MEMS_ERROR) 01471 { 01472 return COMPONENT_ERROR; 01473 } 01474 01475 return COMPONENT_OK; 01476 } 01477 01478 01479 /** 01480 * @brief Enable the HP Filter of the LIS2DH12 sensor 01481 * @param handle the device handle 01482 * @param mode the HP Filter mode to be set 01483 * @param cutoff the HP Filter cutoff frequency to be set 01484 * @retval COMPONENT_OK in case of success 01485 * @retval COMPONENT_ERROR in case of failure 01486 */ 01487 static DrvStatusTypeDef LIS2DH12_Enable_HP_Filter(DrvContextTypeDef *handle) 01488 { 01489 if (LIS2DH12_SetHPFMode((void *)handle, LIS2DH12_HPM_NORMAL_MODE) == MEMS_ERROR) 01490 { 01491 return COMPONENT_ERROR; 01492 } 01493 01494 if (LIS2DH12_SetHPFCutOFF((void *)handle, LIS2DH12_HPFCF_1) == MEMS_ERROR) 01495 { 01496 return COMPONENT_ERROR; 01497 } 01498 01499 if (LIS2DH12_SetFilterDataSel((void *)handle, LIS2DH12_ENABLE) == MEMS_ERROR) 01500 { 01501 return COMPONENT_ERROR; 01502 } 01503 01504 return COMPONENT_OK; 01505 } 01506 01507 01508 /** 01509 * @brief Disable the HP Filter of the LIS2DH12 sensor 01510 * @param handle the device handle 01511 * @retval COMPONENT_OK in case of success 01512 * @retval COMPONENT_ERROR in case of failure 01513 */ 01514 static DrvStatusTypeDef LIS2DH12_Disable_HP_Filter(DrvContextTypeDef *handle) 01515 { 01516 if (LIS2DH12_SetFilterDataSel((void *)handle, LIS2DH12_DISABLE) == MEMS_ERROR) 01517 { 01518 return COMPONENT_ERROR; 01519 } 01520 01521 return COMPONENT_OK; 01522 } 01523 01524 01525 /** 01526 * @brief Clear DRDY of the LIS2DH12 sensor 01527 * @param handle the device handle 01528 * @retval COMPONENT_OK in case of success 01529 * @retval COMPONENT_ERROR in case of failure 01530 */ 01531 static DrvStatusTypeDef LIS2DH12_ClearDRDY(DrvContextTypeDef *handle, ACTIVE_AXIS_t axl_axis) 01532 { 01533 uint8_t regValue[6]; 01534 01535 switch (axl_axis) 01536 { 01537 case X_AXIS: 01538 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_OUT_X_L, regValue, 2) == MEMS_ERROR) 01539 { 01540 return COMPONENT_ERROR; 01541 } 01542 break; 01543 case Y_AXIS: 01544 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_OUT_Y_L, regValue, 2) == MEMS_ERROR) 01545 { 01546 return COMPONENT_ERROR; 01547 } 01548 break; 01549 case Z_AXIS: 01550 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_OUT_Z_L, regValue, 2) == MEMS_ERROR) 01551 { 01552 return COMPONENT_ERROR; 01553 } 01554 break; 01555 case ALL_ACTIVE: 01556 if (LIS2DH12_ACC_ReadReg(handle, LIS2DH12_OUT_X_L, regValue, 6) == MEMS_ERROR) 01557 { 01558 return COMPONENT_ERROR; 01559 } 01560 break; 01561 default: 01562 return COMPONENT_ERROR; 01563 } 01564 01565 return COMPONENT_OK; 01566 } 01567 01568 01569 01570 /** 01571 * @brief Set DRDY enable/disable of the LIS2DH12 sensor on INT1 01572 * @param handle the device handle 01573 * @retval COMPONENT_OK in case of success 01574 * @retval COMPONENT_ERROR in case of failure 01575 */ 01576 static DrvStatusTypeDef LIS2DH12_Set_INT1_DRDY(DrvContextTypeDef *handle, INT1_DRDY_CONFIG_t axl_drdyStatus) 01577 { 01578 LIS2DH12_IntPinConf_t pinConf; 01579 01580 switch (axl_drdyStatus) 01581 { 01582 case INT1_DRDY_DISABLED: 01583 pinConf = LIS2DH12_I1_DRDY1_ON_INT1_DISABLE; 01584 break; 01585 case INT1_DRDY_ENABLED: 01586 pinConf = LIS2DH12_I1_DRDY1_ON_INT1_ENABLE; 01587 break; 01588 default: 01589 return COMPONENT_ERROR; 01590 } 01591 01592 if (LIS2DH12_SetInt1Pin((void *)handle, pinConf) == MEMS_ERROR) 01593 { 01594 return COMPONENT_ERROR; 01595 } 01596 01597 return COMPONENT_OK; 01598 } 01599 01600 /** 01601 * @} 01602 */ 01603 01604 /** 01605 * @} 01606 */ 01607 01608 /** 01609 * @} 01610 */ 01611 01612 /** 01613 * @} 01614 */ 01615 01616 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 16:29:49 by
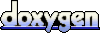