
test sending sensor results over lora radio. Accelerometer and temp/pressure.
Functions | |
static DrvStatusTypeDef | LIS2DH12_Init (DrvContextTypeDef *handle) |
Initialize the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_DeInit (DrvContextTypeDef *handle) |
Deinitialize the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Sensor_Enable (DrvContextTypeDef *handle) |
Enable the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Sensor_Disable (DrvContextTypeDef *handle) |
Disable the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Get_WhoAmI (DrvContextTypeDef *handle, uint8_t *who_am_i) |
Get the WHO_AM_I ID of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Check_WhoAmI (DrvContextTypeDef *handle) |
Check the WHO_AM_I ID of the LIS2DH12 sensor. | |
static DrvStatusTypeDef | LIS2DH12_Get_Axes (DrvContextTypeDef *handle, SensorAxes_t *acceleration) |
Get the LIS2DH12 accelerometer sensor axes. | |
static DrvStatusTypeDef | LIS2DH12_Get_AxesRaw (DrvContextTypeDef *handle, SensorAxesRaw_t *value) |
Get the LIS2DH12 accelerometer sensor raw axes. | |
static DrvStatusTypeDef | LIS2DH12_Get_Sensitivity (DrvContextTypeDef *handle, float *sensitivity) |
Get the LIS2DH12 accelerometer sensor sensitivity. | |
static DrvStatusTypeDef | LIS2DH12_Get_ODR (DrvContextTypeDef *handle, float *odr) |
Get the LIS2DH12 accelerometer sensor output data rate. | |
static DrvStatusTypeDef | LIS2DH12_Set_ODR (DrvContextTypeDef *handle, SensorOdr_t odr) |
Set the LIS2DH12 accelerometer sensor output data rate. | |
static DrvStatusTypeDef | LIS2DH12_Set_ODR_Value (DrvContextTypeDef *handle, float odr) |
Set the LIS2DH12 accelerometer sensor output data rate. | |
static DrvStatusTypeDef | LIS2DH12_Get_FS (DrvContextTypeDef *handle, float *fullScale) |
Get the LIS2DH12 accelerometer sensor full scale. | |
static DrvStatusTypeDef | LIS2DH12_Set_FS (DrvContextTypeDef *handle, SensorFs_t fullScale) |
Set the LIS2DH12 accelerometer sensor full scale. | |
static DrvStatusTypeDef | LIS2DH12_Set_FS_Value (DrvContextTypeDef *handle, float fullScale) |
Set the LIS2DH12 accelerometer sensor full scale. | |
static DrvStatusTypeDef | LIS2DH12_Get_Axes_Status (DrvContextTypeDef *handle, uint8_t *xyz_enabled) |
Get the LIS2DH12 accelerometer sensor axes enabled/disabled status. | |
static DrvStatusTypeDef | LIS2DH12_Set_Axes_Status (DrvContextTypeDef *handle, uint8_t *enable_xyz) |
Set the LIS2DH12 accelerometer sensor axes enabled/disabled. | |
static DrvStatusTypeDef | LIS2DH12_Read_Reg (DrvContextTypeDef *handle, uint8_t reg, uint8_t *data) |
Read the data from register. | |
static DrvStatusTypeDef | LIS2DH12_Write_Reg (DrvContextTypeDef *handle, uint8_t reg, uint8_t data) |
Write the data to register. | |
static DrvStatusTypeDef | LIS2DH12_Get_DRDY_Status (DrvContextTypeDef *handle, uint8_t *status) |
Get data ready status. |
Function Documentation
static DrvStatusTypeDef LIS2DH12_Check_WhoAmI | ( | DrvContextTypeDef * | handle ) | [static] |
Check the WHO_AM_I ID of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 356 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_DeInit | ( | DrvContextTypeDef * | handle ) | [static] |
Deinitialize the LIS2DH12 sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 246 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_Axes | ( | DrvContextTypeDef * | handle, |
SensorAxes_t * | acceleration | ||
) | [static] |
Get the LIS2DH12 accelerometer sensor axes.
- Parameters:
-
handle the device handle acceleration pointer to where acceleration data write to
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 380 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_Axes_Status | ( | DrvContextTypeDef * | handle, |
uint8_t * | xyz_enabled | ||
) | [static] |
Get the LIS2DH12 accelerometer sensor axes enabled/disabled status.
- Parameters:
-
handle the device handle xyz_enabled pointer to where the vector of the axes enabled/disabled status write to
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 811 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_AxesRaw | ( | DrvContextTypeDef * | handle, |
SensorAxesRaw_t * | value | ||
) | [static] |
Get the LIS2DH12 accelerometer sensor raw axes.
- Parameters:
-
handle the device handle acceleration_raw pointer to where acceleration raw data write to
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 412 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_DRDY_Status | ( | DrvContextTypeDef * | handle, |
uint8_t * | status | ||
) | [static] |
Get data ready status.
- Parameters:
-
handle the device handle status the data ready status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 895 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_FS | ( | DrvContextTypeDef * | handle, |
float * | fullScale | ||
) | [static] |
Get the LIS2DH12 accelerometer sensor full scale.
- Parameters:
-
handle the device handle fullScale pointer to where full scale write to
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 706 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_ODR | ( | DrvContextTypeDef * | handle, |
float * | odr | ||
) | [static] |
Get the LIS2DH12 accelerometer sensor output data rate.
- Parameters:
-
handle the device handle odr pointer to where output data rate write to
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 554 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_Sensitivity | ( | DrvContextTypeDef * | handle, |
float * | sensitivity | ||
) | [static] |
Get the LIS2DH12 accelerometer sensor sensitivity.
- Parameters:
-
handle the device handle sensitivity pointer to where sensitivity write to
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 437 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Get_WhoAmI | ( | DrvContextTypeDef * | handle, |
uint8_t * | who_am_i | ||
) | [static] |
Get the WHO_AM_I ID of the LIS2DH12 sensor.
- Parameters:
-
handle the device handle who_am_i pointer to the value of WHO_AM_I register
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 339 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Init | ( | DrvContextTypeDef * | handle ) | [static] |
Initialize the LIS2DH12 sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 184 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Read_Reg | ( | DrvContextTypeDef * | handle, |
uint8_t | reg, | ||
uint8_t * | data | ||
) | [static] |
Read the data from register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 858 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Sensor_Disable | ( | DrvContextTypeDef * | handle ) | [static] |
Disable the LIS2DH12 sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 304 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Sensor_Enable | ( | DrvContextTypeDef * | handle ) | [static] |
Enable the LIS2DH12 sensor.
- Parameters:
-
handle the device handle
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 276 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_Axes_Status | ( | DrvContextTypeDef * | handle, |
uint8_t * | enable_xyz | ||
) | [static] |
Set the LIS2DH12 accelerometer sensor axes enabled/disabled.
- Parameters:
-
handle the device handle enable_xyz vector of the axes enabled/disabled status
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 834 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_FS | ( | DrvContextTypeDef * | handle, |
SensorFs_t | fullScale | ||
) | [static] |
Set the LIS2DH12 accelerometer sensor full scale.
- Parameters:
-
handle the device handle fullScale the functional full scale to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 744 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_FS_Value | ( | DrvContextTypeDef * | handle, |
float | fullScale | ||
) | [static] |
Set the LIS2DH12 accelerometer sensor full scale.
- Parameters:
-
handle the device handle fullScale the full scale value to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 787 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_ODR | ( | DrvContextTypeDef * | handle, |
SensorOdr_t | odr | ||
) | [static] |
Set the LIS2DH12 accelerometer sensor output data rate.
- Parameters:
-
handle the device handle odr the functional output data rate to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 652 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Set_ODR_Value | ( | DrvContextTypeDef * | handle, |
float | odr | ||
) | [static] |
Set the LIS2DH12 accelerometer sensor output data rate.
- Parameters:
-
handle the device handle odr the output data rate value to be set
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 679 of file LIS2DH12_ACC_driver_HL.c.
static DrvStatusTypeDef LIS2DH12_Write_Reg | ( | DrvContextTypeDef * | handle, |
uint8_t | reg, | ||
uint8_t | data | ||
) | [static] |
Write the data to register.
- Parameters:
-
handle the device handle reg register address data register data
- Return values:
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 877 of file LIS2DH12_ACC_driver_HL.c.
Generated on Tue Jul 12 2022 16:29:50 by
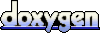