NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
sys.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_SYS_H__ 00033 #define __LWIP_SYS_H__ 00034 00035 #include "lwip/opt.h" 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 #if NO_SYS 00042 00043 /* For a totally minimal and standalone system, we provide null 00044 definitions of the sys_ functions. */ 00045 typedef u8_t sys_sem_t; 00046 typedef u8_t sys_mutex_t; 00047 typedef u8_t sys_mbox_t; 00048 00049 #define sys_sem_new(s, c) ERR_OK 00050 #define sys_sem_signal(s) 00051 #define sys_sem_wait(s) 00052 #define sys_arch_sem_wait(s,t) 00053 #define sys_sem_free(s) 00054 #define sys_mutex_new(mu) ERR_OK 00055 #define sys_mutex_lock(mu) 00056 #define sys_mutex_unlock(mu) 00057 #define sys_mutex_free(mu) 00058 #define sys_mbox_new(m, s) ERR_OK 00059 #define sys_mbox_fetch(m,d) 00060 #define sys_mbox_tryfetch(m,d) 00061 #define sys_mbox_post(m,d) 00062 #define sys_mbox_trypost(m,d) 00063 #define sys_mbox_free(m) 00064 00065 #define sys_thread_new(n,t,a,s,p) 00066 00067 #define sys_msleep(t) 00068 00069 #else /* NO_SYS */ 00070 00071 /** Return code for timeouts from sys_arch_mbox_fetch and sys_arch_sem_wait */ 00072 #define SYS_ARCH_TIMEOUT 0xffffffffUL 00073 00074 /** sys_mbox_tryfetch() returns SYS_MBOX_EMPTY if appropriate. 00075 * For now we use the same magic value, but we allow this to change in future. 00076 */ 00077 #define SYS_MBOX_EMPTY SYS_ARCH_TIMEOUT 00078 00079 #include "lwip/err.h" 00080 #include "arch/sys_arch.h" 00081 00082 /** Function prototype for thread functions */ 00083 typedef void (*lwip_thread_fn)(void *arg); 00084 00085 /* Function prototypes for functions to be implemented by platform ports 00086 (in sys_arch.c) */ 00087 00088 /* Mutex functions: */ 00089 00090 /** Define LWIP_COMPAT_MUTEX if the port has no mutexes and binary semaphores 00091 should be used instead */ 00092 #if LWIP_COMPAT_MUTEX 00093 /* for old ports that don't have mutexes: define them to binary semaphores */ 00094 #define sys_mutex_t sys_sem_t 00095 #define sys_mutex_new(mutex) sys_sem_new(mutex, 1) 00096 #define sys_mutex_lock(mutex) sys_sem_wait(mutex) 00097 #define sys_mutex_unlock(mutex) sys_sem_signal(mutex) 00098 #define sys_mutex_free(mutex) sys_sem_free(mutex) 00099 #define sys_mutex_valid(mutex) sys_sem_valid(mutex) 00100 #define sys_mutex_set_invalid(mutex) sys_sem_set_invalid(mutex) 00101 00102 #else /* LWIP_COMPAT_MUTEX */ 00103 00104 /** Create a new mutex 00105 * @param mutex pointer to the mutex to create 00106 * @return a new mutex */ 00107 err_t sys_mutex_new(sys_mutex_t *mutex); 00108 /** Lock a mutex 00109 * @param mutex the mutex to lock */ 00110 void sys_mutex_lock(sys_mutex_t *mutex); 00111 /** Unlock a mutex 00112 * @param mutex the mutex to unlock */ 00113 void sys_mutex_unlock(sys_mutex_t *mutex); 00114 /** Delete a semaphore 00115 * @param mutex the mutex to delete */ 00116 void sys_mutex_free(sys_mutex_t *mutex); 00117 #ifndef sys_mutex_valid 00118 /** Check if a mutex is valid/allocated: return 1 for valid, 0 for invalid */ 00119 int sys_mutex_valid(sys_mutex_t *mutex); 00120 #endif 00121 #ifndef sys_mutex_set_invalid 00122 /** Set a mutex invalid so that sys_mutex_valid returns 0 */ 00123 void sys_mutex_set_invalid(sys_mutex_t *mutex); 00124 #endif 00125 #endif /* LWIP_COMPAT_MUTEX */ 00126 00127 /* Semaphore functions: */ 00128 00129 /** Create a new semaphore 00130 * @param sem pointer to the semaphore to create 00131 * @param count initial count of the semaphore 00132 * @return ERR_OK if successful, another err_t otherwise */ 00133 err_t sys_sem_new(sys_sem_t *sem, u8_t count); 00134 /** Signals a semaphore 00135 * @param sem the semaphore to signal */ 00136 void sys_sem_signal(sys_sem_t *sem); 00137 /** Wait for a semaphore for the specified timeout 00138 * @param sem the semaphore to wait for 00139 * @param timeout timeout in milliseconds to wait (0 = wait forever) 00140 * @return time (in milliseconds) waited for the semaphore 00141 * or SYS_ARCH_TIMEOUT on timeout */ 00142 u32_t sys_arch_sem_wait(sys_sem_t *sem, u32_t timeout); 00143 /** Delete a semaphore 00144 * @param sem semaphore to delete */ 00145 void sys_sem_free(sys_sem_t *sem); 00146 /** Wait for a semaphore - forever/no timeout */ 00147 #define sys_sem_wait(sem) sys_arch_sem_wait(sem, 0) 00148 #ifndef sys_sem_valid 00149 /** Check if a sempahore is valid/allocated: return 1 for valid, 0 for invalid */ 00150 int sys_sem_valid(sys_sem_t *sem); 00151 #endif 00152 #ifndef sys_sem_set_invalid 00153 /** Set a semaphore invalid so that sys_sem_valid returns 0 */ 00154 void sys_sem_set_invalid(sys_sem_t *sem); 00155 #endif 00156 00157 /* Time functions. */ 00158 #ifndef sys_msleep 00159 void sys_msleep(u32_t ms); /* only has a (close to) 1 jiffy resolution. */ 00160 #endif 00161 00162 /* Mailbox functions. */ 00163 00164 /** Create a new mbox of specified size 00165 * @param mbox pointer to the mbox to create 00166 * @param size (miminum) number of messages in this mbox 00167 * @return ERR_OK if successful, another err_t otherwise */ 00168 err_t sys_mbox_new(sys_mbox_t *mbox, int size); 00169 /** Post a message to an mbox - may not fail 00170 * -> blocks if full, only used from tasks not from ISR 00171 * @param mbox mbox to posts the message 00172 * @param msg message to post (ATTENTION: can be NULL) */ 00173 void sys_mbox_post(sys_mbox_t *mbox, void *msg); 00174 /** Try to post a message to an mbox - may fail if full or ISR 00175 * @param mbox mbox to posts the message 00176 * @param msg message to post (ATTENTION: can be NULL) */ 00177 err_t sys_mbox_trypost(sys_mbox_t *mbox, void *msg); 00178 /** Wait for a new message to arrive in the mbox 00179 * @param mbox mbox to get a message from 00180 * @param msg pointer where the message is stored 00181 * @param timeout maximum time (in milliseconds) to wait for a message 00182 * @return time (in milliseconds) waited for a message, may be 0 if not waited 00183 or SYS_ARCH_TIMEOUT on timeout 00184 * The returned time has to be accurate to prevent timer jitter! */ 00185 u32_t sys_arch_mbox_fetch(sys_mbox_t *mbox, void **msg, u32_t timeout); 00186 /* Allow port to override with a macro, e.g. special timout for sys_arch_mbox_fetch() */ 00187 #ifndef sys_arch_mbox_tryfetch 00188 /** Wait for a new message to arrive in the mbox 00189 * @param mbox mbox to get a message from 00190 * @param msg pointer where the message is stored 00191 * @param timeout maximum time (in milliseconds) to wait for a message 00192 * @return 0 (milliseconds) if a message has been received 00193 * or SYS_MBOX_EMPTY if the mailbox is empty */ 00194 u32_t sys_arch_mbox_tryfetch(sys_mbox_t *mbox, void **msg); 00195 #endif 00196 /** For now, we map straight to sys_arch implementation. */ 00197 #define sys_mbox_tryfetch(mbox, msg) sys_arch_mbox_tryfetch(mbox, msg) 00198 /** Delete an mbox 00199 * @param mbox mbox to delete */ 00200 void sys_mbox_free(sys_mbox_t *mbox); 00201 #define sys_mbox_fetch(mbox, msg) sys_arch_mbox_fetch(mbox, msg, 0) 00202 #ifndef sys_mbox_valid 00203 /** Check if an mbox is valid/allocated: return 1 for valid, 0 for invalid */ 00204 int sys_mbox_valid(sys_mbox_t *mbox); 00205 #endif 00206 #ifndef sys_mbox_set_invalid 00207 /** Set an mbox invalid so that sys_mbox_valid returns 0 */ 00208 void sys_mbox_set_invalid(sys_mbox_t *mbox); 00209 #endif 00210 00211 /** The only thread function: 00212 * Creates a new thread 00213 * @param name human-readable name for the thread (used for debugging purposes) 00214 * @param thread thread-function 00215 * @param arg parameter passed to 'thread' 00216 * @param stacksize stack size in bytes for the new thread (may be ignored by ports) 00217 * @param prio priority of the new thread (may be ignored by ports) */ 00218 sys_thread_t sys_thread_new(const char *name, lwip_thread_fn thread, void *arg, int stacksize, int prio); 00219 00220 #endif /* NO_SYS */ 00221 00222 /* sys_init() must be called before anthing else. */ 00223 void sys_init(void); 00224 00225 #ifndef sys_jiffies 00226 /** Ticks/jiffies since power up. */ 00227 u32_t sys_jiffies(void); 00228 #endif 00229 00230 /** Returns the current time in milliseconds, 00231 * may be the same as sys_jiffies or at least based on it. */ 00232 u32_t sys_now(void); 00233 00234 /* Critical Region Protection */ 00235 /* These functions must be implemented in the sys_arch.c file. 00236 In some implementations they can provide a more light-weight protection 00237 mechanism than using semaphores. Otherwise semaphores can be used for 00238 implementation */ 00239 #ifndef SYS_ARCH_PROTECT 00240 /** SYS_LIGHTWEIGHT_PROT 00241 * define SYS_LIGHTWEIGHT_PROT in lwipopts.h if you want inter-task protection 00242 * for certain critical regions during buffer allocation, deallocation and memory 00243 * allocation and deallocation. 00244 */ 00245 #if SYS_LIGHTWEIGHT_PROT 00246 00247 /** SYS_ARCH_DECL_PROTECT 00248 * declare a protection variable. This macro will default to defining a variable of 00249 * type sys_prot_t. If a particular port needs a different implementation, then 00250 * this macro may be defined in sys_arch.h. 00251 */ 00252 #define SYS_ARCH_DECL_PROTECT(lev) sys_prot_t lev 00253 /** SYS_ARCH_PROTECT 00254 * Perform a "fast" protect. This could be implemented by 00255 * disabling interrupts for an embedded system or by using a semaphore or 00256 * mutex. The implementation should allow calling SYS_ARCH_PROTECT when 00257 * already protected. The old protection level is returned in the variable 00258 * "lev". This macro will default to calling the sys_arch_protect() function 00259 * which should be implemented in sys_arch.c. If a particular port needs a 00260 * different implementation, then this macro may be defined in sys_arch.h 00261 */ 00262 #define SYS_ARCH_PROTECT(lev) lev = sys_arch_protect() 00263 /** SYS_ARCH_UNPROTECT 00264 * Perform a "fast" set of the protection level to "lev". This could be 00265 * implemented by setting the interrupt level to "lev" within the MACRO or by 00266 * using a semaphore or mutex. This macro will default to calling the 00267 * sys_arch_unprotect() function which should be implemented in 00268 * sys_arch.c. If a particular port needs a different implementation, then 00269 * this macro may be defined in sys_arch.h 00270 */ 00271 #define SYS_ARCH_UNPROTECT(lev) sys_arch_unprotect(lev) 00272 sys_prot_t sys_arch_protect(void); 00273 void sys_arch_unprotect(sys_prot_t pval); 00274 00275 #else 00276 00277 #define SYS_ARCH_DECL_PROTECT(lev) 00278 #define SYS_ARCH_PROTECT(lev) 00279 #define SYS_ARCH_UNPROTECT(lev) 00280 00281 #endif /* SYS_LIGHTWEIGHT_PROT */ 00282 00283 #endif /* SYS_ARCH_PROTECT */ 00284 00285 /* 00286 * Macros to set/get and increase/decrease variables in a thread-safe way. 00287 * Use these for accessing variable that are used from more than one thread. 00288 */ 00289 00290 #ifndef SYS_ARCH_INC 00291 #define SYS_ARCH_INC(var, val) do { \ 00292 SYS_ARCH_DECL_PROTECT(old_level); \ 00293 SYS_ARCH_PROTECT(old_level); \ 00294 var += val; \ 00295 SYS_ARCH_UNPROTECT(old_level); \ 00296 } while(0) 00297 #endif /* SYS_ARCH_INC */ 00298 00299 #ifndef SYS_ARCH_DEC 00300 #define SYS_ARCH_DEC(var, val) do { \ 00301 SYS_ARCH_DECL_PROTECT(old_level); \ 00302 SYS_ARCH_PROTECT(old_level); \ 00303 var -= val; \ 00304 SYS_ARCH_UNPROTECT(old_level); \ 00305 } while(0) 00306 #endif /* SYS_ARCH_DEC */ 00307 00308 #ifndef SYS_ARCH_GET 00309 #define SYS_ARCH_GET(var, ret) do { \ 00310 SYS_ARCH_DECL_PROTECT(old_level); \ 00311 SYS_ARCH_PROTECT(old_level); \ 00312 ret = var; \ 00313 SYS_ARCH_UNPROTECT(old_level); \ 00314 } while(0) 00315 #endif /* SYS_ARCH_GET */ 00316 00317 #ifndef SYS_ARCH_SET 00318 #define SYS_ARCH_SET(var, val) do { \ 00319 SYS_ARCH_DECL_PROTECT(old_level); \ 00320 SYS_ARCH_PROTECT(old_level); \ 00321 var = val; \ 00322 SYS_ARCH_UNPROTECT(old_level); \ 00323 } while(0) 00324 #endif /* SYS_ARCH_SET */ 00325 00326 00327 #ifdef __cplusplus 00328 } 00329 #endif 00330 00331 #endif /* __LWIP_SYS_H__ */
Generated on Tue Jul 12 2022 11:52:58 by
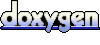