NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
sys.c
00001 /** 00002 * @file 00003 * lwIP Operating System abstraction 00004 * 00005 */ 00006 00007 /* 00008 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. The name of the author may not be used to endorse or promote products 00020 * derived from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00023 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00024 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00025 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00026 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00027 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00028 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00029 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00030 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00031 * OF SUCH DAMAGE. 00032 * 00033 * This file is part of the lwIP TCP/IP stack. 00034 * 00035 * Author: Adam Dunkels <adam@sics.se> 00036 * 00037 */ 00038 00039 #include "lwip/opt.h" 00040 00041 #include "lwip/sys.h" 00042 00043 /* Most of the functions defined in sys.h must be implemented in the 00044 * architecture-dependent file sys_arch.c */ 00045 00046 #if !NO_SYS 00047 00048 /** 00049 * Sleep for some ms. Timeouts are NOT processed while sleeping. 00050 * 00051 * @param ms number of milliseconds to sleep 00052 */ 00053 void 00054 sys_msleep(u32_t ms) 00055 { 00056 if (ms > 0) { 00057 sys_sem_t delaysem; 00058 err_t err = sys_sem_new(&delaysem, 0); 00059 if (err == ERR_OK) { 00060 sys_arch_sem_wait(&delaysem, ms); 00061 sys_sem_free(&delaysem); 00062 } 00063 } 00064 } 00065 00066 #endif /* !NO_SYS */
Generated on Tue Jul 12 2022 11:52:58 by
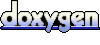