NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
stats.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_STATS_H__ 00033 #define __LWIP_STATS_H__ 00034 00035 #include "lwip/opt.h" 00036 00037 #include "lwip/mem.h" 00038 #include "lwip/memp.h" 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 #if LWIP_STATS 00045 00046 #ifndef LWIP_STATS_LARGE 00047 #define LWIP_STATS_LARGE 0 00048 #endif 00049 00050 #if LWIP_STATS_LARGE 00051 #define STAT_COUNTER u32_t 00052 #define STAT_COUNTER_F U32_F 00053 #else 00054 #define STAT_COUNTER u16_t 00055 #define STAT_COUNTER_F U16_F 00056 #endif 00057 00058 struct stats_proto { 00059 STAT_COUNTER xmit; /* Transmitted packets. */ 00060 STAT_COUNTER recv; /* Received packets. */ 00061 STAT_COUNTER fw; /* Forwarded packets. */ 00062 STAT_COUNTER drop; /* Dropped packets. */ 00063 STAT_COUNTER chkerr; /* Checksum error. */ 00064 STAT_COUNTER lenerr; /* Invalid length error. */ 00065 STAT_COUNTER memerr; /* Out of memory error. */ 00066 STAT_COUNTER rterr; /* Routing error. */ 00067 STAT_COUNTER proterr; /* Protocol error. */ 00068 STAT_COUNTER opterr; /* Error in options. */ 00069 STAT_COUNTER err; /* Misc error. */ 00070 STAT_COUNTER cachehit; 00071 }; 00072 00073 struct stats_igmp { 00074 STAT_COUNTER xmit; /* Transmitted packets. */ 00075 STAT_COUNTER recv; /* Received packets. */ 00076 STAT_COUNTER drop; /* Dropped packets. */ 00077 STAT_COUNTER chkerr; /* Checksum error. */ 00078 STAT_COUNTER lenerr; /* Invalid length error. */ 00079 STAT_COUNTER memerr; /* Out of memory error. */ 00080 STAT_COUNTER proterr; /* Protocol error. */ 00081 STAT_COUNTER rx_v1; /* Received v1 frames. */ 00082 STAT_COUNTER rx_group; /* Received group-specific queries. */ 00083 STAT_COUNTER rx_general; /* Received general queries. */ 00084 STAT_COUNTER rx_report; /* Received reports. */ 00085 STAT_COUNTER tx_join; /* Sent joins. */ 00086 STAT_COUNTER tx_leave; /* Sent leaves. */ 00087 STAT_COUNTER tx_report; /* Sent reports. */ 00088 }; 00089 00090 struct stats_mem { 00091 #ifdef LWIP_DEBUG 00092 const char *name; 00093 #endif /* LWIP_DEBUG */ 00094 mem_size_t avail; 00095 mem_size_t used; 00096 mem_size_t max; 00097 STAT_COUNTER err; 00098 STAT_COUNTER illegal; 00099 }; 00100 00101 struct stats_syselem { 00102 STAT_COUNTER used; 00103 STAT_COUNTER max; 00104 STAT_COUNTER err; 00105 }; 00106 00107 struct stats_sys { 00108 struct stats_syselem sem; 00109 struct stats_syselem mutex; 00110 struct stats_syselem mbox; 00111 }; 00112 00113 struct stats_ { 00114 #if LINK_STATS 00115 struct stats_proto link; 00116 #endif 00117 #if ETHARP_STATS 00118 struct stats_proto etharp; 00119 #endif 00120 #if IPFRAG_STATS 00121 struct stats_proto ip_frag; 00122 #endif 00123 #if IP_STATS 00124 struct stats_proto ip; 00125 #endif 00126 #if ICMP_STATS 00127 struct stats_proto icmp; 00128 #endif 00129 #if IGMP_STATS 00130 struct stats_igmp igmp; 00131 #endif 00132 #if UDP_STATS 00133 struct stats_proto udp; 00134 #endif 00135 #if TCP_STATS 00136 struct stats_proto tcp; 00137 #endif 00138 #if MEM_STATS 00139 struct stats_mem mem; 00140 #endif 00141 #if MEMP_STATS 00142 struct stats_mem memp[MEMP_MAX]; 00143 #endif 00144 #if SYS_STATS 00145 struct stats_sys sys; 00146 #endif 00147 }; 00148 00149 extern struct stats_ lwip_stats; 00150 00151 void stats_init(void); 00152 00153 #define STATS_INC(x) ++lwip_stats.x 00154 #define STATS_DEC(x) --lwip_stats.x 00155 #define STATS_INC_USED(x, y) do { lwip_stats.x.used += y; \ 00156 if (lwip_stats.x.max < lwip_stats.x.used) { \ 00157 lwip_stats.x.max = lwip_stats.x.used; \ 00158 } \ 00159 } while(0) 00160 #else /* LWIP_STATS */ 00161 #define stats_init() 00162 #define STATS_INC(x) 00163 #define STATS_DEC(x) 00164 #define STATS_INC_USED(x) 00165 #endif /* LWIP_STATS */ 00166 00167 #if TCP_STATS 00168 #define TCP_STATS_INC(x) STATS_INC(x) 00169 #define TCP_STATS_DISPLAY() stats_display_proto(&lwip_stats.tcp, "TCP") 00170 #else 00171 #define TCP_STATS_INC(x) 00172 #define TCP_STATS_DISPLAY() 00173 #endif 00174 00175 #if UDP_STATS 00176 #define UDP_STATS_INC(x) STATS_INC(x) 00177 #define UDP_STATS_DISPLAY() stats_display_proto(&lwip_stats.udp, "UDP") 00178 #else 00179 #define UDP_STATS_INC(x) 00180 #define UDP_STATS_DISPLAY() 00181 #endif 00182 00183 #if ICMP_STATS 00184 #define ICMP_STATS_INC(x) STATS_INC(x) 00185 #define ICMP_STATS_DISPLAY() stats_display_proto(&lwip_stats.icmp, "ICMP") 00186 #else 00187 #define ICMP_STATS_INC(x) 00188 #define ICMP_STATS_DISPLAY() 00189 #endif 00190 00191 #if IGMP_STATS 00192 #define IGMP_STATS_INC(x) STATS_INC(x) 00193 #define IGMP_STATS_DISPLAY() stats_display_igmp(&lwip_stats.igmp) 00194 #else 00195 #define IGMP_STATS_INC(x) 00196 #define IGMP_STATS_DISPLAY() 00197 #endif 00198 00199 #if IP_STATS 00200 #define IP_STATS_INC(x) STATS_INC(x) 00201 #define IP_STATS_DISPLAY() stats_display_proto(&lwip_stats.ip, "IP") 00202 #else 00203 #define IP_STATS_INC(x) 00204 #define IP_STATS_DISPLAY() 00205 #endif 00206 00207 #if IPFRAG_STATS 00208 #define IPFRAG_STATS_INC(x) STATS_INC(x) 00209 #define IPFRAG_STATS_DISPLAY() stats_display_proto(&lwip_stats.ip_frag, "IP_FRAG") 00210 #else 00211 #define IPFRAG_STATS_INC(x) 00212 #define IPFRAG_STATS_DISPLAY() 00213 #endif 00214 00215 #if ETHARP_STATS 00216 #define ETHARP_STATS_INC(x) STATS_INC(x) 00217 #define ETHARP_STATS_DISPLAY() stats_display_proto(&lwip_stats.etharp, "ETHARP") 00218 #else 00219 #define ETHARP_STATS_INC(x) 00220 #define ETHARP_STATS_DISPLAY() 00221 #endif 00222 00223 #if LINK_STATS 00224 #define LINK_STATS_INC(x) STATS_INC(x) 00225 #define LINK_STATS_DISPLAY() stats_display_proto(&lwip_stats.link, "LINK") 00226 #else 00227 #define LINK_STATS_INC(x) 00228 #define LINK_STATS_DISPLAY() 00229 #endif 00230 00231 #if MEM_STATS 00232 #define MEM_STATS_AVAIL(x, y) lwip_stats.mem.x = y 00233 #define MEM_STATS_INC(x) STATS_INC(mem.x) 00234 #define MEM_STATS_INC_USED(x, y) STATS_INC_USED(mem, y) 00235 #define MEM_STATS_DEC_USED(x, y) lwip_stats.mem.x -= y 00236 #define MEM_STATS_DISPLAY() stats_display_mem(&lwip_stats.mem, "HEAP") 00237 #else 00238 #define MEM_STATS_AVAIL(x, y) 00239 #define MEM_STATS_INC(x) 00240 #define MEM_STATS_INC_USED(x, y) 00241 #define MEM_STATS_DEC_USED(x, y) 00242 #define MEM_STATS_DISPLAY() 00243 #endif 00244 00245 #if MEMP_STATS 00246 #define MEMP_STATS_AVAIL(x, i, y) lwip_stats.memp[i].x = y 00247 #define MEMP_STATS_INC(x, i) STATS_INC(memp[i].x) 00248 #define MEMP_STATS_DEC(x, i) STATS_DEC(memp[i].x) 00249 #define MEMP_STATS_INC_USED(x, i) STATS_INC_USED(memp[i], 1) 00250 #define MEMP_STATS_DISPLAY(i) stats_display_memp(&lwip_stats.memp[i], i) 00251 #else 00252 #define MEMP_STATS_AVAIL(x, i, y) 00253 #define MEMP_STATS_INC(x, i) 00254 #define MEMP_STATS_DEC(x, i) 00255 #define MEMP_STATS_INC_USED(x, i) 00256 #define MEMP_STATS_DISPLAY(i) 00257 #endif 00258 00259 #if SYS_STATS 00260 #define SYS_STATS_INC(x) STATS_INC(sys.x) 00261 #define SYS_STATS_DEC(x) STATS_DEC(sys.x) 00262 #define SYS_STATS_INC_USED(x) STATS_INC_USED(sys.x, 1) 00263 #define SYS_STATS_DISPLAY() stats_display_sys(&lwip_stats.sys) 00264 #else 00265 #define SYS_STATS_INC(x) 00266 #define SYS_STATS_DEC(x) 00267 #define SYS_STATS_INC_USED(x) 00268 #define SYS_STATS_DISPLAY() 00269 #endif 00270 00271 /* Display of statistics */ 00272 #if LWIP_STATS_DISPLAY 00273 void stats_display(void); 00274 void stats_display_proto(struct stats_proto *proto, char *name); 00275 void stats_display_igmp(struct stats_igmp *igmp); 00276 void stats_display_mem(struct stats_mem *mem, char *name); 00277 void stats_display_memp(struct stats_mem *mem, int index); 00278 void stats_display_sys(struct stats_sys *sys); 00279 #else /* LWIP_STATS_DISPLAY */ 00280 #define stats_display() 00281 #define stats_display_proto(proto, name) 00282 #define stats_display_igmp(igmp) 00283 #define stats_display_mem(mem, name) 00284 #define stats_display_memp(mem, index) 00285 #define stats_display_sys(sys) 00286 #endif /* LWIP_STATS_DISPLAY */ 00287 00288 #ifdef __cplusplus 00289 } 00290 #endif 00291 00292 #endif /* __LWIP_STATS_H__ */
Generated on Tue Jul 12 2022 11:52:58 by
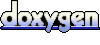