NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
opt.h
00001 /** 00002 * @file 00003 * 00004 * lwIP Options Configuration 00005 */ 00006 00007 /* 00008 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. The name of the author may not be used to endorse or promote products 00020 * derived from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00023 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00024 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00025 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00026 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00027 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00028 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00029 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00030 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00031 * OF SUCH DAMAGE. 00032 * 00033 * This file is part of the lwIP TCP/IP stack. 00034 * 00035 * Author: Adam Dunkels <adam@sics.se> 00036 * 00037 */ 00038 #ifndef __LWIP_OPT_H__ 00039 #define __LWIP_OPT_H__ 00040 00041 /* 00042 * Include user defined options first. Anything not defined in these files 00043 * will be set to standard values. Override anything you dont like! 00044 */ 00045 #include "lwipopts.h" 00046 #include "lwip/debug.h" 00047 00048 /* 00049 ----------------------------------------------- 00050 ---------- Platform specific locking ---------- 00051 ----------------------------------------------- 00052 */ 00053 00054 /** 00055 * SYS_LIGHTWEIGHT_PROT==1: if you want inter-task protection for certain 00056 * critical regions during buffer allocation, deallocation and memory 00057 * allocation and deallocation. 00058 */ 00059 #ifndef SYS_LIGHTWEIGHT_PROT 00060 #define SYS_LIGHTWEIGHT_PROT 0 00061 #endif 00062 00063 /** 00064 * NO_SYS==1: Provides VERY minimal functionality. Otherwise, 00065 * use lwIP facilities. 00066 */ 00067 #ifndef NO_SYS 00068 #define NO_SYS 0 00069 #endif 00070 00071 /** 00072 * NO_SYS_NO_TIMERS==1: Drop support for sys_timeout when NO_SYS==1 00073 * Mainly for compatibility to old versions. 00074 */ 00075 #ifndef NO_SYS_NO_TIMERS 00076 #define NO_SYS_NO_TIMERS 0 00077 #endif 00078 00079 /** 00080 * MEMCPY: override this if you have a faster implementation at hand than the 00081 * one included in your C library 00082 */ 00083 #ifndef MEMCPY 00084 #define MEMCPY(dst,src,len) memcpy(dst,src,len) 00085 #endif 00086 00087 /** 00088 * SMEMCPY: override this with care! Some compilers (e.g. gcc) can inline a 00089 * call to memcpy() if the length is known at compile time and is small. 00090 */ 00091 #ifndef SMEMCPY 00092 #define SMEMCPY(dst,src,len) memcpy(dst,src,len) 00093 #endif 00094 00095 /* 00096 ------------------------------------ 00097 ---------- Memory options ---------- 00098 ------------------------------------ 00099 */ 00100 /** 00101 * MEM_LIBC_MALLOC==1: Use malloc/free/realloc provided by your C-library 00102 * instead of the lwip internal allocator. Can save code size if you 00103 * already use it. 00104 */ 00105 #ifndef MEM_LIBC_MALLOC 00106 #define MEM_LIBC_MALLOC 0 00107 #endif 00108 00109 /** 00110 * MEMP_MEM_MALLOC==1: Use mem_malloc/mem_free instead of the lwip pool allocator. 00111 * Especially useful with MEM_LIBC_MALLOC but handle with care regarding execution 00112 * speed and usage from interrupts! 00113 */ 00114 #ifndef MEMP_MEM_MALLOC 00115 #define MEMP_MEM_MALLOC 0 00116 #endif 00117 00118 /** 00119 * MEM_ALIGNMENT: should be set to the alignment of the CPU 00120 * 4 byte alignment -> #define MEM_ALIGNMENT 4 00121 * 2 byte alignment -> #define MEM_ALIGNMENT 2 00122 */ 00123 #ifndef MEM_ALIGNMENT 00124 #define MEM_ALIGNMENT 1 00125 #endif 00126 00127 /** 00128 * MEM_SIZE: the size of the heap memory. If the application will send 00129 * a lot of data that needs to be copied, this should be set high. 00130 */ 00131 #ifndef MEM_SIZE 00132 #define MEM_SIZE 1600 00133 #endif 00134 00135 /** 00136 * MEMP_SEPARATE_POOLS: if defined to 1, each pool is placed in its own array. 00137 * This can be used to individually change the location of each pool. 00138 * Default is one big array for all pools 00139 */ 00140 #ifndef MEMP_SEPARATE_POOLS 00141 #define MEMP_SEPARATE_POOLS 0 00142 #endif 00143 00144 /** 00145 * MEMP_OVERFLOW_CHECK: memp overflow protection reserves a configurable 00146 * amount of bytes before and after each memp element in every pool and fills 00147 * it with a prominent default value. 00148 * MEMP_OVERFLOW_CHECK == 0 no checking 00149 * MEMP_OVERFLOW_CHECK == 1 checks each element when it is freed 00150 * MEMP_OVERFLOW_CHECK >= 2 checks each element in every pool every time 00151 * memp_malloc() or memp_free() is called (useful but slow!) 00152 */ 00153 #ifndef MEMP_OVERFLOW_CHECK 00154 #define MEMP_OVERFLOW_CHECK 0 00155 #endif 00156 00157 /** 00158 * MEMP_SANITY_CHECK==1: run a sanity check after each memp_free() to make 00159 * sure that there are no cycles in the linked lists. 00160 */ 00161 #ifndef MEMP_SANITY_CHECK 00162 #define MEMP_SANITY_CHECK 0 00163 #endif 00164 00165 /** 00166 * MEM_USE_POOLS==1: Use an alternative to malloc() by allocating from a set 00167 * of memory pools of various sizes. When mem_malloc is called, an element of 00168 * the smallest pool that can provide the length needed is returned. 00169 * To use this, MEMP_USE_CUSTOM_POOLS also has to be enabled. 00170 */ 00171 #ifndef MEM_USE_POOLS 00172 #define MEM_USE_POOLS 0 00173 #endif 00174 00175 /** 00176 * MEM_USE_POOLS_TRY_BIGGER_POOL==1: if one malloc-pool is empty, try the next 00177 * bigger pool - WARNING: THIS MIGHT WASTE MEMORY but it can make a system more 00178 * reliable. */ 00179 #ifndef MEM_USE_POOLS_TRY_BIGGER_POOL 00180 #define MEM_USE_POOLS_TRY_BIGGER_POOL 0 00181 #endif 00182 00183 /** 00184 * MEMP_USE_CUSTOM_POOLS==1: whether to include a user file lwippools.h 00185 * that defines additional pools beyond the "standard" ones required 00186 * by lwIP. If you set this to 1, you must have lwippools.h in your 00187 * inlude path somewhere. 00188 */ 00189 #ifndef MEMP_USE_CUSTOM_POOLS 00190 #define MEMP_USE_CUSTOM_POOLS 0 00191 #endif 00192 00193 /** 00194 * Set this to 1 if you want to free PBUF_RAM pbufs (or call mem_free()) from 00195 * interrupt context (or another context that doesn't allow waiting for a 00196 * semaphore). 00197 * If set to 1, mem_malloc will be protected by a semaphore and SYS_ARCH_PROTECT, 00198 * while mem_free will only use SYS_ARCH_PROTECT. mem_malloc SYS_ARCH_UNPROTECTs 00199 * with each loop so that mem_free can run. 00200 * 00201 * ATTENTION: As you can see from the above description, this leads to dis-/ 00202 * enabling interrupts often, which can be slow! Also, on low memory, mem_malloc 00203 * can need longer. 00204 * 00205 * If you don't want that, at least for NO_SYS=0, you can still use the following 00206 * functions to enqueue a deallocation call which then runs in the tcpip_thread 00207 * context: 00208 * - pbuf_free_callback(p); 00209 * - mem_free_callback(m); 00210 */ 00211 #ifndef LWIP_ALLOW_MEM_FREE_FROM_OTHER_CONTEXT 00212 #define LWIP_ALLOW_MEM_FREE_FROM_OTHER_CONTEXT 0 00213 #endif 00214 00215 /* 00216 ------------------------------------------------ 00217 ---------- Internal Memory Pool Sizes ---------- 00218 ------------------------------------------------ 00219 */ 00220 /** 00221 * MEMP_NUM_PBUF: the number of memp struct pbufs (used for PBUF_ROM and PBUF_REF). 00222 * If the application sends a lot of data out of ROM (or other static memory), 00223 * this should be set high. 00224 */ 00225 #ifndef MEMP_NUM_PBUF 00226 #define MEMP_NUM_PBUF 16 00227 #endif 00228 00229 /** 00230 * MEMP_NUM_RAW_PCB: Number of raw connection PCBs 00231 * (requires the LWIP_RAW option) 00232 */ 00233 #ifndef MEMP_NUM_RAW_PCB 00234 #define MEMP_NUM_RAW_PCB 4 00235 #endif 00236 00237 /** 00238 * MEMP_NUM_UDP_PCB: the number of UDP protocol control blocks. One 00239 * per active UDP "connection". 00240 * (requires the LWIP_UDP option) 00241 */ 00242 #ifndef MEMP_NUM_UDP_PCB 00243 #define MEMP_NUM_UDP_PCB 4 00244 #endif 00245 00246 /** 00247 * MEMP_NUM_TCP_PCB: the number of simulatenously active TCP connections. 00248 * (requires the LWIP_TCP option) 00249 */ 00250 #ifndef MEMP_NUM_TCP_PCB 00251 #define MEMP_NUM_TCP_PCB 5 00252 #endif 00253 00254 /** 00255 * MEMP_NUM_TCP_PCB_LISTEN: the number of listening TCP connections. 00256 * (requires the LWIP_TCP option) 00257 */ 00258 #ifndef MEMP_NUM_TCP_PCB_LISTEN 00259 #define MEMP_NUM_TCP_PCB_LISTEN 8 00260 #endif 00261 00262 /** 00263 * MEMP_NUM_TCP_SEG: the number of simultaneously queued TCP segments. 00264 * (requires the LWIP_TCP option) 00265 */ 00266 #ifndef MEMP_NUM_TCP_SEG 00267 #define MEMP_NUM_TCP_SEG 16 00268 #endif 00269 00270 /** 00271 * MEMP_NUM_REASSDATA: the number of IP packets simultaneously queued for 00272 * reassembly (whole packets, not fragments!) 00273 */ 00274 #ifndef MEMP_NUM_REASSDATA 00275 #define MEMP_NUM_REASSDATA 5 00276 #endif 00277 00278 /** 00279 * MEMP_NUM_FRAG_PBUF: the number of IP fragments simultaneously sent 00280 * (fragments, not whole packets!). 00281 * This is only used with IP_FRAG_USES_STATIC_BUF==0 and 00282 * LWIP_NETIF_TX_SINGLE_PBUF==0 and only has to be > 1 with DMA-enabled MACs 00283 * where the packet is not yet sent when netif->output returns. 00284 */ 00285 #ifndef MEMP_NUM_FRAG_PBUF 00286 #define MEMP_NUM_FRAG_PBUF 15 00287 #endif 00288 00289 /** 00290 * MEMP_NUM_ARP_QUEUE: the number of simulateously queued outgoing 00291 * packets (pbufs) that are waiting for an ARP request (to resolve 00292 * their destination address) to finish. 00293 * (requires the ARP_QUEUEING option) 00294 */ 00295 #ifndef MEMP_NUM_ARP_QUEUE 00296 #define MEMP_NUM_ARP_QUEUE 30 00297 #endif 00298 00299 /** 00300 * MEMP_NUM_IGMP_GROUP: The number of multicast groups whose network interfaces 00301 * can be members et the same time (one per netif - allsystems group -, plus one 00302 * per netif membership). 00303 * (requires the LWIP_IGMP option) 00304 */ 00305 #ifndef MEMP_NUM_IGMP_GROUP 00306 #define MEMP_NUM_IGMP_GROUP 8 00307 #endif 00308 00309 /** 00310 * MEMP_NUM_SYS_TIMEOUT: the number of simulateously active timeouts. 00311 * (requires NO_SYS==0) 00312 */ 00313 #ifndef MEMP_NUM_SYS_TIMEOUT 00314 #define MEMP_NUM_SYS_TIMEOUT 3 00315 #endif 00316 00317 /** 00318 * MEMP_NUM_NETBUF: the number of struct netbufs. 00319 * (only needed if you use the sequential API, like api_lib.c) 00320 */ 00321 #ifndef MEMP_NUM_NETBUF 00322 #define MEMP_NUM_NETBUF 2 00323 #endif 00324 00325 /** 00326 * MEMP_NUM_NETCONN: the number of struct netconns. 00327 * (only needed if you use the sequential API, like api_lib.c) 00328 */ 00329 #ifndef MEMP_NUM_NETCONN 00330 #define MEMP_NUM_NETCONN 4 00331 #endif 00332 00333 /** 00334 * MEMP_NUM_TCPIP_MSG_API: the number of struct tcpip_msg, which are used 00335 * for callback/timeout API communication. 00336 * (only needed if you use tcpip.c) 00337 */ 00338 #ifndef MEMP_NUM_TCPIP_MSG_API 00339 #define MEMP_NUM_TCPIP_MSG_API 8 00340 #endif 00341 00342 /** 00343 * MEMP_NUM_TCPIP_MSG_INPKT: the number of struct tcpip_msg, which are used 00344 * for incoming packets. 00345 * (only needed if you use tcpip.c) 00346 */ 00347 #ifndef MEMP_NUM_TCPIP_MSG_INPKT 00348 #define MEMP_NUM_TCPIP_MSG_INPKT 8 00349 #endif 00350 00351 /** 00352 * MEMP_NUM_SNMP_NODE: the number of leafs in the SNMP tree. 00353 */ 00354 #ifndef MEMP_NUM_SNMP_NODE 00355 #define MEMP_NUM_SNMP_NODE 50 00356 #endif 00357 00358 /** 00359 * MEMP_NUM_SNMP_ROOTNODE: the number of branches in the SNMP tree. 00360 * Every branch has one leaf (MEMP_NUM_SNMP_NODE) at least! 00361 */ 00362 #ifndef MEMP_NUM_SNMP_ROOTNODE 00363 #define MEMP_NUM_SNMP_ROOTNODE 30 00364 #endif 00365 00366 /** 00367 * MEMP_NUM_SNMP_VARBIND: the number of concurrent requests (does not have to 00368 * be changed normally) - 2 of these are used per request (1 for input, 00369 * 1 for output) 00370 */ 00371 #ifndef MEMP_NUM_SNMP_VARBIND 00372 #define MEMP_NUM_SNMP_VARBIND 2 00373 #endif 00374 00375 /** 00376 * MEMP_NUM_SNMP_VALUE: the number of OID or values concurrently used 00377 * (does not have to be changed normally) - 3 of these are used per request 00378 * (1 for the value read and 2 for OIDs - input and output) 00379 */ 00380 #ifndef MEMP_NUM_SNMP_VALUE 00381 #define MEMP_NUM_SNMP_VALUE 3 00382 #endif 00383 00384 /** 00385 * MEMP_NUM_NETDB: the number of concurrently running lwip_addrinfo() calls 00386 * (before freeing the corresponding memory using lwip_freeaddrinfo()). 00387 */ 00388 #ifndef MEMP_NUM_NETDB 00389 #define MEMP_NUM_NETDB 1 00390 #endif 00391 00392 /** 00393 * MEMP_NUM_LOCALHOSTLIST: the number of host entries in the local host list 00394 * if DNS_LOCAL_HOSTLIST_IS_DYNAMIC==1. 00395 */ 00396 #ifndef MEMP_NUM_LOCALHOSTLIST 00397 #define MEMP_NUM_LOCALHOSTLIST 1 00398 #endif 00399 00400 /** 00401 * MEMP_NUM_PPPOE_INTERFACES: the number of concurrently active PPPoE 00402 * interfaces (only used with PPPOE_SUPPORT==1) 00403 */ 00404 #ifndef MEMP_NUM_PPPOE_INTERFACES 00405 #define MEMP_NUM_PPPOE_INTERFACES 1 00406 #endif 00407 00408 /** 00409 * PBUF_POOL_SIZE: the number of buffers in the pbuf pool. 00410 */ 00411 #ifndef PBUF_POOL_SIZE 00412 #define PBUF_POOL_SIZE 16 00413 #endif 00414 00415 /* 00416 --------------------------------- 00417 ---------- ARP options ---------- 00418 --------------------------------- 00419 */ 00420 /** 00421 * LWIP_ARP==1: Enable ARP functionality. 00422 */ 00423 #ifndef LWIP_ARP 00424 #define LWIP_ARP 1 00425 #endif 00426 00427 /** 00428 * ARP_TABLE_SIZE: Number of active MAC-IP address pairs cached. 00429 */ 00430 #ifndef ARP_TABLE_SIZE 00431 #define ARP_TABLE_SIZE 10 00432 #endif 00433 00434 /** 00435 * ARP_QUEUEING==1: Outgoing packets are queued during hardware address 00436 * resolution. 00437 */ 00438 #ifndef ARP_QUEUEING 00439 #define ARP_QUEUEING 1 00440 #endif 00441 00442 /** 00443 * ETHARP_TRUST_IP_MAC==1: Incoming IP packets cause the ARP table to be 00444 * updated with the source MAC and IP addresses supplied in the packet. 00445 * You may want to disable this if you do not trust LAN peers to have the 00446 * correct addresses, or as a limited approach to attempt to handle 00447 * spoofing. If disabled, lwIP will need to make a new ARP request if 00448 * the peer is not already in the ARP table, adding a little latency. 00449 * The peer *is* in the ARP table if it requested our address before. 00450 * Also notice that this slows down input processing of every IP packet! 00451 */ 00452 #ifndef ETHARP_TRUST_IP_MAC 00453 #define ETHARP_TRUST_IP_MAC 0 00454 #endif 00455 00456 /** 00457 * ETHARP_SUPPORT_VLAN==1: support receiving ethernet packets with VLAN header. 00458 * Additionally, you can define ETHARP_VLAN_CHECK to an u16_t VLAN ID to check. 00459 * If ETHARP_VLAN_CHECK is defined, only VLAN-traffic for this VLAN is accepted. 00460 * If ETHARP_VLAN_CHECK is not defined, all traffic is accepted. 00461 */ 00462 #ifndef ETHARP_SUPPORT_VLAN 00463 #define ETHARP_SUPPORT_VLAN 0 00464 #endif 00465 00466 /** LWIP_ETHERNET==1: enable ethernet support for PPPoE even though ARP 00467 * might be disabled 00468 */ 00469 #ifndef LWIP_ETHERNET 00470 #define LWIP_ETHERNET (LWIP_ARP || PPPOE_SUPPORT) 00471 #endif 00472 00473 /** ETH_PAD_SIZE: number of bytes added before the ethernet header to ensure 00474 * alignment of payload after that header. Since the header is 14 bytes long, 00475 * without this padding e.g. addresses in the IP header will not be aligned 00476 * on a 32-bit boundary, so setting this to 2 can speed up 32-bit-platforms. 00477 */ 00478 #ifndef ETH_PAD_SIZE 00479 #define ETH_PAD_SIZE 0 00480 #endif 00481 00482 /** ETHARP_SUPPORT_STATIC_ENTRIES==1: enable code to support static ARP table 00483 * entries (using etharp_add_static_entry/etharp_remove_static_entry). 00484 */ 00485 #ifndef ETHARP_SUPPORT_STATIC_ENTRIES 00486 #define ETHARP_SUPPORT_STATIC_ENTRIES 0 00487 #endif 00488 00489 00490 /* 00491 -------------------------------- 00492 ---------- IP options ---------- 00493 -------------------------------- 00494 */ 00495 /** 00496 * IP_FORWARD==1: Enables the ability to forward IP packets across network 00497 * interfaces. If you are going to run lwIP on a device with only one network 00498 * interface, define this to 0. 00499 */ 00500 #ifndef IP_FORWARD 00501 #define IP_FORWARD 0 00502 #endif 00503 00504 /** 00505 * IP_OPTIONS_ALLOWED: Defines the behavior for IP options. 00506 * IP_OPTIONS_ALLOWED==0: All packets with IP options are dropped. 00507 * IP_OPTIONS_ALLOWED==1: IP options are allowed (but not parsed). 00508 */ 00509 #ifndef IP_OPTIONS_ALLOWED 00510 #define IP_OPTIONS_ALLOWED 1 00511 #endif 00512 00513 /** 00514 * IP_REASSEMBLY==1: Reassemble incoming fragmented IP packets. Note that 00515 * this option does not affect outgoing packet sizes, which can be controlled 00516 * via IP_FRAG. 00517 */ 00518 #ifndef IP_REASSEMBLY 00519 #define IP_REASSEMBLY 1 00520 #endif 00521 00522 /** 00523 * IP_FRAG==1: Fragment outgoing IP packets if their size exceeds MTU. Note 00524 * that this option does not affect incoming packet sizes, which can be 00525 * controlled via IP_REASSEMBLY. 00526 */ 00527 #ifndef IP_FRAG 00528 #define IP_FRAG 1 00529 #endif 00530 00531 /** 00532 * IP_REASS_MAXAGE: Maximum time (in multiples of IP_TMR_INTERVAL - so seconds, normally) 00533 * a fragmented IP packet waits for all fragments to arrive. If not all fragments arrived 00534 * in this time, the whole packet is discarded. 00535 */ 00536 #ifndef IP_REASS_MAXAGE 00537 #define IP_REASS_MAXAGE 3 00538 #endif 00539 00540 /** 00541 * IP_REASS_MAX_PBUFS: Total maximum amount of pbufs waiting to be reassembled. 00542 * Since the received pbufs are enqueued, be sure to configure 00543 * PBUF_POOL_SIZE > IP_REASS_MAX_PBUFS so that the stack is still able to receive 00544 * packets even if the maximum amount of fragments is enqueued for reassembly! 00545 */ 00546 #ifndef IP_REASS_MAX_PBUFS 00547 #define IP_REASS_MAX_PBUFS 10 00548 #endif 00549 00550 /** 00551 * IP_FRAG_USES_STATIC_BUF==1: Use a static MTU-sized buffer for IP 00552 * fragmentation. Otherwise pbufs are allocated and reference the original 00553 * packet data to be fragmented (or with LWIP_NETIF_TX_SINGLE_PBUF==1, 00554 * new PBUF_RAM pbufs are used for fragments). 00555 * ATTENTION: IP_FRAG_USES_STATIC_BUF==1 may not be used for DMA-enabled MACs! 00556 */ 00557 #ifndef IP_FRAG_USES_STATIC_BUF 00558 #define IP_FRAG_USES_STATIC_BUF 0 00559 #endif 00560 00561 /** 00562 * IP_FRAG_MAX_MTU: Assumed max MTU on any interface for IP frag buffer 00563 * (requires IP_FRAG_USES_STATIC_BUF==1) 00564 */ 00565 #if IP_FRAG_USES_STATIC_BUF && !defined(IP_FRAG_MAX_MTU) 00566 #define IP_FRAG_MAX_MTU 1500 00567 #endif 00568 00569 /** 00570 * IP_DEFAULT_TTL: Default value for Time-To-Live used by transport layers. 00571 */ 00572 #ifndef IP_DEFAULT_TTL 00573 #define IP_DEFAULT_TTL 255 00574 #endif 00575 00576 /** 00577 * IP_SOF_BROADCAST=1: Use the SOF_BROADCAST field to enable broadcast 00578 * filter per pcb on udp and raw send operations. To enable broadcast filter 00579 * on recv operations, you also have to set IP_SOF_BROADCAST_RECV=1. 00580 */ 00581 #ifndef IP_SOF_BROADCAST 00582 #define IP_SOF_BROADCAST 0 00583 #endif 00584 00585 /** 00586 * IP_SOF_BROADCAST_RECV (requires IP_SOF_BROADCAST=1) enable the broadcast 00587 * filter on recv operations. 00588 */ 00589 #ifndef IP_SOF_BROADCAST_RECV 00590 #define IP_SOF_BROADCAST_RECV 0 00591 #endif 00592 00593 /* 00594 ---------------------------------- 00595 ---------- ICMP options ---------- 00596 ---------------------------------- 00597 */ 00598 /** 00599 * LWIP_ICMP==1: Enable ICMP module inside the IP stack. 00600 * Be careful, disable that make your product non-compliant to RFC1122 00601 */ 00602 #ifndef LWIP_ICMP 00603 #define LWIP_ICMP 1 00604 #endif 00605 00606 /** 00607 * ICMP_TTL: Default value for Time-To-Live used by ICMP packets. 00608 */ 00609 #ifndef ICMP_TTL 00610 #define ICMP_TTL (IP_DEFAULT_TTL) 00611 #endif 00612 00613 /** 00614 * LWIP_BROADCAST_PING==1: respond to broadcast pings (default is unicast only) 00615 */ 00616 #ifndef LWIP_BROADCAST_PING 00617 #define LWIP_BROADCAST_PING 0 00618 #endif 00619 00620 /** 00621 * LWIP_MULTICAST_PING==1: respond to multicast pings (default is unicast only) 00622 */ 00623 #ifndef LWIP_MULTICAST_PING 00624 #define LWIP_MULTICAST_PING 0 00625 #endif 00626 00627 /* 00628 --------------------------------- 00629 ---------- RAW options ---------- 00630 --------------------------------- 00631 */ 00632 /** 00633 * LWIP_RAW==1: Enable application layer to hook into the IP layer itself. 00634 */ 00635 #ifndef LWIP_RAW 00636 #define LWIP_RAW 1 00637 #endif 00638 00639 /** 00640 * LWIP_RAW==1: Enable application layer to hook into the IP layer itself. 00641 */ 00642 #ifndef RAW_TTL 00643 #define RAW_TTL (IP_DEFAULT_TTL) 00644 #endif 00645 00646 /* 00647 ---------------------------------- 00648 ---------- DHCP options ---------- 00649 ---------------------------------- 00650 */ 00651 /** 00652 * LWIP_DHCP==1: Enable DHCP module. 00653 */ 00654 #ifndef LWIP_DHCP 00655 #define LWIP_DHCP 0 00656 #endif 00657 00658 /** 00659 * DHCP_DOES_ARP_CHECK==1: Do an ARP check on the offered address. 00660 */ 00661 #ifndef DHCP_DOES_ARP_CHECK 00662 #define DHCP_DOES_ARP_CHECK ((LWIP_DHCP) && (LWIP_ARP)) 00663 #endif 00664 00665 /* 00666 ------------------------------------ 00667 ---------- AUTOIP options ---------- 00668 ------------------------------------ 00669 */ 00670 /** 00671 * LWIP_AUTOIP==1: Enable AUTOIP module. 00672 */ 00673 #ifndef LWIP_AUTOIP 00674 #define LWIP_AUTOIP 0 00675 #endif 00676 00677 /** 00678 * LWIP_DHCP_AUTOIP_COOP==1: Allow DHCP and AUTOIP to be both enabled on 00679 * the same interface at the same time. 00680 */ 00681 #ifndef LWIP_DHCP_AUTOIP_COOP 00682 #define LWIP_DHCP_AUTOIP_COOP 0 00683 #endif 00684 00685 /** 00686 * LWIP_DHCP_AUTOIP_COOP_TRIES: Set to the number of DHCP DISCOVER probes 00687 * that should be sent before falling back on AUTOIP. This can be set 00688 * as low as 1 to get an AutoIP address very quickly, but you should 00689 * be prepared to handle a changing IP address when DHCP overrides 00690 * AutoIP. 00691 */ 00692 #ifndef LWIP_DHCP_AUTOIP_COOP_TRIES 00693 #define LWIP_DHCP_AUTOIP_COOP_TRIES 9 00694 #endif 00695 00696 /* 00697 ---------------------------------- 00698 ---------- SNMP options ---------- 00699 ---------------------------------- 00700 */ 00701 /** 00702 * LWIP_SNMP==1: Turn on SNMP module. UDP must be available for SNMP 00703 * transport. 00704 */ 00705 #ifndef LWIP_SNMP 00706 #define LWIP_SNMP 0 00707 #endif 00708 00709 /** 00710 * SNMP_CONCURRENT_REQUESTS: Number of concurrent requests the module will 00711 * allow. At least one request buffer is required. 00712 * Does not have to be changed unless external MIBs answer request asynchronously 00713 */ 00714 #ifndef SNMP_CONCURRENT_REQUESTS 00715 #define SNMP_CONCURRENT_REQUESTS 1 00716 #endif 00717 00718 /** 00719 * SNMP_TRAP_DESTINATIONS: Number of trap destinations. At least one trap 00720 * destination is required 00721 */ 00722 #ifndef SNMP_TRAP_DESTINATIONS 00723 #define SNMP_TRAP_DESTINATIONS 1 00724 #endif 00725 00726 /** 00727 * SNMP_PRIVATE_MIB: 00728 * When using a private MIB, you have to create a file 'private_mib.h' that contains 00729 * a 'struct mib_array_node mib_private' which contains your MIB. 00730 */ 00731 #ifndef SNMP_PRIVATE_MIB 00732 #define SNMP_PRIVATE_MIB 0 00733 #endif 00734 00735 /** 00736 * Only allow SNMP write actions that are 'safe' (e.g. disabeling netifs is not 00737 * a safe action and disabled when SNMP_SAFE_REQUESTS = 1). 00738 * Unsafe requests are disabled by default! 00739 */ 00740 #ifndef SNMP_SAFE_REQUESTS 00741 #define SNMP_SAFE_REQUESTS 1 00742 #endif 00743 00744 /** 00745 * The maximum length of strings used. This affects the size of 00746 * MEMP_SNMP_VALUE elements. 00747 */ 00748 #ifndef SNMP_MAX_OCTET_STRING_LEN 00749 #define SNMP_MAX_OCTET_STRING_LEN 127 00750 #endif 00751 00752 /** 00753 * The maximum depth of the SNMP tree. 00754 * With private MIBs enabled, this depends on your MIB! 00755 * This affects the size of MEMP_SNMP_VALUE elements. 00756 */ 00757 #ifndef SNMP_MAX_TREE_DEPTH 00758 #define SNMP_MAX_TREE_DEPTH 15 00759 #endif 00760 00761 /** 00762 * The size of the MEMP_SNMP_VALUE elements, normally calculated from 00763 * SNMP_MAX_OCTET_STRING_LEN and SNMP_MAX_TREE_DEPTH. 00764 */ 00765 #ifndef SNMP_MAX_VALUE_SIZE 00766 #define SNMP_MAX_VALUE_SIZE LWIP_MAX((SNMP_MAX_OCTET_STRING_LEN)+1, sizeof(s32_t)*(SNMP_MAX_TREE_DEPTH)) 00767 #endif 00768 00769 /* 00770 ---------------------------------- 00771 ---------- IGMP options ---------- 00772 ---------------------------------- 00773 */ 00774 /** 00775 * LWIP_IGMP==1: Turn on IGMP module. 00776 */ 00777 #ifndef LWIP_IGMP 00778 #define LWIP_IGMP 0 00779 #endif 00780 00781 /* 00782 ---------------------------------- 00783 ---------- DNS options ----------- 00784 ---------------------------------- 00785 */ 00786 /** 00787 * LWIP_DNS==1: Turn on DNS module. UDP must be available for DNS 00788 * transport. 00789 */ 00790 #ifndef LWIP_DNS 00791 #define LWIP_DNS 0 00792 #endif 00793 00794 /** DNS maximum number of entries to maintain locally. */ 00795 #ifndef DNS_TABLE_SIZE 00796 #define DNS_TABLE_SIZE 4 00797 #endif 00798 00799 /** DNS maximum host name length supported in the name table. */ 00800 #ifndef DNS_MAX_NAME_LENGTH 00801 #define DNS_MAX_NAME_LENGTH 256 00802 #endif 00803 00804 /** The maximum of DNS servers */ 00805 #ifndef DNS_MAX_SERVERS 00806 #define DNS_MAX_SERVERS 2 00807 #endif 00808 00809 /** DNS do a name checking between the query and the response. */ 00810 #ifndef DNS_DOES_NAME_CHECK 00811 #define DNS_DOES_NAME_CHECK 1 00812 #endif 00813 00814 /** DNS message max. size. Default value is RFC compliant. */ 00815 #ifndef DNS_MSG_SIZE 00816 #define DNS_MSG_SIZE 512 00817 #endif 00818 00819 /** DNS_LOCAL_HOSTLIST: Implements a local host-to-address list. If enabled, 00820 * you have to define 00821 * #define DNS_LOCAL_HOSTLIST_INIT {{"host1", 0x123}, {"host2", 0x234}} 00822 * (an array of structs name/address, where address is an u32_t in network 00823 * byte order). 00824 * 00825 * Instead, you can also use an external function: 00826 * #define DNS_LOOKUP_LOCAL_EXTERN(x) extern u32_t my_lookup_function(const char *name) 00827 * that returns the IP address or INADDR_NONE if not found. 00828 */ 00829 #ifndef DNS_LOCAL_HOSTLIST 00830 #define DNS_LOCAL_HOSTLIST 0 00831 #endif /* DNS_LOCAL_HOSTLIST */ 00832 00833 /** If this is turned on, the local host-list can be dynamically changed 00834 * at runtime. */ 00835 #ifndef DNS_LOCAL_HOSTLIST_IS_DYNAMIC 00836 #define DNS_LOCAL_HOSTLIST_IS_DYNAMIC 0 00837 #endif /* DNS_LOCAL_HOSTLIST_IS_DYNAMIC */ 00838 00839 /* 00840 --------------------------------- 00841 ---------- UDP options ---------- 00842 --------------------------------- 00843 */ 00844 /** 00845 * LWIP_UDP==1: Turn on UDP. 00846 */ 00847 #ifndef LWIP_UDP 00848 #define LWIP_UDP 1 00849 #endif 00850 00851 /** 00852 * LWIP_UDPLITE==1: Turn on UDP-Lite. (Requires LWIP_UDP) 00853 */ 00854 #ifndef LWIP_UDPLITE 00855 #define LWIP_UDPLITE 0 00856 #endif 00857 00858 /** 00859 * UDP_TTL: Default Time-To-Live value. 00860 */ 00861 #ifndef UDP_TTL 00862 #define UDP_TTL (IP_DEFAULT_TTL) 00863 #endif 00864 00865 /** 00866 * LWIP_NETBUF_RECVINFO==1: append destination addr and port to every netbuf. 00867 */ 00868 #ifndef LWIP_NETBUF_RECVINFO 00869 #define LWIP_NETBUF_RECVINFO 0 00870 #endif 00871 00872 /* 00873 --------------------------------- 00874 ---------- TCP options ---------- 00875 --------------------------------- 00876 */ 00877 /** 00878 * LWIP_TCP==1: Turn on TCP. 00879 */ 00880 #ifndef LWIP_TCP 00881 #define LWIP_TCP 1 00882 #endif 00883 00884 /** 00885 * TCP_TTL: Default Time-To-Live value. 00886 */ 00887 #ifndef TCP_TTL 00888 #define TCP_TTL (IP_DEFAULT_TTL) 00889 #endif 00890 00891 /** 00892 * TCP_WND: The size of a TCP window. This must be at least 00893 * (2 * TCP_MSS) for things to work well 00894 */ 00895 #ifndef TCP_WND 00896 #define TCP_WND (4 * TCP_MSS) 00897 #endif 00898 00899 /** 00900 * TCP_MAXRTX: Maximum number of retransmissions of data segments. 00901 */ 00902 #ifndef TCP_MAXRTX 00903 #define TCP_MAXRTX 12 00904 #endif 00905 00906 /** 00907 * TCP_SYNMAXRTX: Maximum number of retransmissions of SYN segments. 00908 */ 00909 #ifndef TCP_SYNMAXRTX 00910 #define TCP_SYNMAXRTX 6 00911 #endif 00912 00913 /** 00914 * TCP_QUEUE_OOSEQ==1: TCP will queue segments that arrive out of order. 00915 * Define to 0 if your device is low on memory. 00916 */ 00917 #ifndef TCP_QUEUE_OOSEQ 00918 #define TCP_QUEUE_OOSEQ (LWIP_TCP) 00919 #endif 00920 00921 /** 00922 * TCP_MSS: TCP Maximum segment size. (default is 536, a conservative default, 00923 * you might want to increase this.) 00924 * For the receive side, this MSS is advertised to the remote side 00925 * when opening a connection. For the transmit size, this MSS sets 00926 * an upper limit on the MSS advertised by the remote host. 00927 */ 00928 #ifndef TCP_MSS 00929 #define TCP_MSS 536 00930 #endif 00931 00932 /** 00933 * TCP_CALCULATE_EFF_SEND_MSS: "The maximum size of a segment that TCP really 00934 * sends, the 'effective send MSS,' MUST be the smaller of the send MSS (which 00935 * reflects the available reassembly buffer size at the remote host) and the 00936 * largest size permitted by the IP layer" (RFC 1122) 00937 * Setting this to 1 enables code that checks TCP_MSS against the MTU of the 00938 * netif used for a connection and limits the MSS if it would be too big otherwise. 00939 */ 00940 #ifndef TCP_CALCULATE_EFF_SEND_MSS 00941 #define TCP_CALCULATE_EFF_SEND_MSS 1 00942 #endif 00943 00944 00945 /** 00946 * TCP_SND_BUF: TCP sender buffer space (bytes). 00947 */ 00948 #ifndef TCP_SND_BUF 00949 #define TCP_SND_BUF 256 00950 #endif 00951 00952 /** 00953 * TCP_SND_QUEUELEN: TCP sender buffer space (pbufs). This must be at least 00954 * as much as (2 * TCP_SND_BUF/TCP_MSS) for things to work. 00955 */ 00956 #ifndef TCP_SND_QUEUELEN 00957 #define TCP_SND_QUEUELEN (4 * (TCP_SND_BUF)/(TCP_MSS)) 00958 #endif 00959 00960 /** 00961 * TCP_SNDLOWAT: TCP writable space (bytes). This must be less than 00962 * TCP_SND_BUF. It is the amount of space which must be available in the 00963 * TCP snd_buf for select to return writable (combined with TCP_SNDQUEUELOWAT). 00964 */ 00965 #ifndef TCP_SNDLOWAT 00966 #define TCP_SNDLOWAT ((TCP_SND_BUF)/2) 00967 #endif 00968 00969 /** 00970 * TCP_SNDQUEUELOWAT: TCP writable bufs (pbuf count). This must be grater 00971 * than TCP_SND_QUEUELEN. If the number of pbufs queued on a pcb drops below 00972 * this number, select returns writable (combined with TCP_SNDLOWAT). 00973 */ 00974 #ifndef TCP_SNDQUEUELOWAT 00975 #define TCP_SNDQUEUELOWAT ((TCP_SND_QUEUELEN)/2) 00976 #endif 00977 00978 /** 00979 * TCP_LISTEN_BACKLOG: Enable the backlog option for tcp listen pcb. 00980 */ 00981 #ifndef TCP_LISTEN_BACKLOG 00982 #define TCP_LISTEN_BACKLOG 0 00983 #endif 00984 00985 /** 00986 * The maximum allowed backlog for TCP listen netconns. 00987 * This backlog is used unless another is explicitly specified. 00988 * 0xff is the maximum (u8_t). 00989 */ 00990 #ifndef TCP_DEFAULT_LISTEN_BACKLOG 00991 #define TCP_DEFAULT_LISTEN_BACKLOG 0xff 00992 #endif 00993 00994 /** 00995 * TCP_OVERSIZE: The maximum number of bytes that tcp_write may 00996 * allocate ahead of time in an attempt to create shorter pbuf chains 00997 * for transmission. The meaningful range is 0 to TCP_MSS. Some 00998 * suggested values are: 00999 * 01000 * 0: Disable oversized allocation. Each tcp_write() allocates a new 01001 pbuf (old behaviour). 01002 * 1: Allocate size-aligned pbufs with minimal excess. Use this if your 01003 * scatter-gather DMA requires aligned fragments. 01004 * 128: Limit the pbuf/memory overhead to 20%. 01005 * TCP_MSS: Try to create unfragmented TCP packets. 01006 * TCP_MSS/4: Try to create 4 fragments or less per TCP packet. 01007 */ 01008 #ifndef TCP_OVERSIZE 01009 #define TCP_OVERSIZE TCP_MSS 01010 #endif 01011 01012 /** 01013 * LWIP_TCP_TIMESTAMPS==1: support the TCP timestamp option. 01014 */ 01015 #ifndef LWIP_TCP_TIMESTAMPS 01016 #define LWIP_TCP_TIMESTAMPS 0 01017 #endif 01018 01019 /** 01020 * TCP_WND_UPDATE_THRESHOLD: difference in window to trigger an 01021 * explicit window update 01022 */ 01023 #ifndef TCP_WND_UPDATE_THRESHOLD 01024 #define TCP_WND_UPDATE_THRESHOLD (TCP_WND / 4) 01025 #endif 01026 01027 /** 01028 * LWIP_EVENT_API and LWIP_CALLBACK_API: Only one of these should be set to 1. 01029 * LWIP_EVENT_API==1: The user defines lwip_tcp_event() to receive all 01030 * events (accept, sent, etc) that happen in the system. 01031 * LWIP_CALLBACK_API==1: The PCB callback function is called directly 01032 * for the event. 01033 */ 01034 #ifndef LWIP_EVENT_API 01035 #define LWIP_EVENT_API 0 01036 #define LWIP_CALLBACK_API 1 01037 #else 01038 #define LWIP_EVENT_API 1 01039 #define LWIP_CALLBACK_API 0 01040 #endif 01041 01042 01043 /* 01044 ---------------------------------- 01045 ---------- Pbuf options ---------- 01046 ---------------------------------- 01047 */ 01048 /** 01049 * PBUF_LINK_HLEN: the number of bytes that should be allocated for a 01050 * link level header. The default is 14, the standard value for 01051 * Ethernet. 01052 */ 01053 #ifndef PBUF_LINK_HLEN 01054 #define PBUF_LINK_HLEN (14 + ETH_PAD_SIZE) 01055 #endif 01056 01057 /** 01058 * PBUF_POOL_BUFSIZE: the size of each pbuf in the pbuf pool. The default is 01059 * designed to accomodate single full size TCP frame in one pbuf, including 01060 * TCP_MSS, IP header, and link header. 01061 */ 01062 #ifndef PBUF_POOL_BUFSIZE 01063 #define PBUF_POOL_BUFSIZE LWIP_MEM_ALIGN_SIZE(TCP_MSS+40+PBUF_LINK_HLEN) 01064 #endif 01065 01066 /* 01067 ------------------------------------------------ 01068 ---------- Network Interfaces options ---------- 01069 ------------------------------------------------ 01070 */ 01071 /** 01072 * LWIP_NETIF_HOSTNAME==1: use DHCP_OPTION_HOSTNAME with netif's hostname 01073 * field. 01074 */ 01075 #ifndef LWIP_NETIF_HOSTNAME 01076 #define LWIP_NETIF_HOSTNAME 0 01077 #endif 01078 01079 /** 01080 * LWIP_NETIF_API==1: Support netif api (in netifapi.c) 01081 */ 01082 #ifndef LWIP_NETIF_API 01083 #define LWIP_NETIF_API 0 01084 #endif 01085 01086 /** 01087 * LWIP_NETIF_STATUS_CALLBACK==1: Support a callback function whenever an interface 01088 * changes its up/down status (i.e., due to DHCP IP acquistion) 01089 */ 01090 #ifndef LWIP_NETIF_STATUS_CALLBACK 01091 #define LWIP_NETIF_STATUS_CALLBACK 0 01092 #endif 01093 01094 /** 01095 * LWIP_NETIF_LINK_CALLBACK==1: Support a callback function from an interface 01096 * whenever the link changes (i.e., link down) 01097 */ 01098 #ifndef LWIP_NETIF_LINK_CALLBACK 01099 #define LWIP_NETIF_LINK_CALLBACK 0 01100 #endif 01101 01102 /** 01103 * LWIP_NETIF_HWADDRHINT==1: Cache link-layer-address hints (e.g. table 01104 * indices) in struct netif. TCP and UDP can make use of this to prevent 01105 * scanning the ARP table for every sent packet. While this is faster for big 01106 * ARP tables or many concurrent connections, it might be counterproductive 01107 * if you have a tiny ARP table or if there never are concurrent connections. 01108 */ 01109 #ifndef LWIP_NETIF_HWADDRHINT 01110 #define LWIP_NETIF_HWADDRHINT 0 01111 #endif 01112 01113 /** 01114 * LWIP_NETIF_LOOPBACK==1: Support sending packets with a destination IP 01115 * address equal to the netif IP address, looping them back up the stack. 01116 */ 01117 #ifndef LWIP_NETIF_LOOPBACK 01118 #define LWIP_NETIF_LOOPBACK 0 01119 #endif 01120 01121 /** 01122 * LWIP_LOOPBACK_MAX_PBUFS: Maximum number of pbufs on queue for loopback 01123 * sending for each netif (0 = disabled) 01124 */ 01125 #ifndef LWIP_LOOPBACK_MAX_PBUFS 01126 #define LWIP_LOOPBACK_MAX_PBUFS 0 01127 #endif 01128 01129 /** 01130 * LWIP_NETIF_LOOPBACK_MULTITHREADING: Indicates whether threading is enabled in 01131 * the system, as netifs must change how they behave depending on this setting 01132 * for the LWIP_NETIF_LOOPBACK option to work. 01133 * Setting this is needed to avoid reentering non-reentrant functions like 01134 * tcp_input(). 01135 * LWIP_NETIF_LOOPBACK_MULTITHREADING==1: Indicates that the user is using a 01136 * multithreaded environment like tcpip.c. In this case, netif->input() 01137 * is called directly. 01138 * LWIP_NETIF_LOOPBACK_MULTITHREADING==0: Indicates a polling (or NO_SYS) setup. 01139 * The packets are put on a list and netif_poll() must be called in 01140 * the main application loop. 01141 */ 01142 #ifndef LWIP_NETIF_LOOPBACK_MULTITHREADING 01143 #define LWIP_NETIF_LOOPBACK_MULTITHREADING (!NO_SYS) 01144 #endif 01145 01146 /** 01147 * LWIP_NETIF_TX_SINGLE_PBUF: if this is set to 1, lwIP tries to put all data 01148 * to be sent into one single pbuf. This is for compatibility with DMA-enabled 01149 * MACs that do not support scatter-gather. 01150 * Beware that this might involve CPU-memcpy before transmitting that would not 01151 * be needed without this flag! Use this only if you need to! 01152 * 01153 * @todo: TCP and IP-frag do not work with this, yet: 01154 */ 01155 #ifndef LWIP_NETIF_TX_SINGLE_PBUF 01156 #define LWIP_NETIF_TX_SINGLE_PBUF 0 01157 #endif /* LWIP_NETIF_TX_SINGLE_PBUF */ 01158 01159 /* 01160 ------------------------------------ 01161 ---------- LOOPIF options ---------- 01162 ------------------------------------ 01163 */ 01164 /** 01165 * LWIP_HAVE_LOOPIF==1: Support loop interface (127.0.0.1) and loopif.c 01166 */ 01167 #ifndef LWIP_HAVE_LOOPIF 01168 #define LWIP_HAVE_LOOPIF 0 01169 #endif 01170 01171 /* 01172 ------------------------------------ 01173 ---------- SLIPIF options ---------- 01174 ------------------------------------ 01175 */ 01176 /** 01177 * LWIP_HAVE_SLIPIF==1: Support slip interface and slipif.c 01178 */ 01179 #ifndef LWIP_HAVE_SLIPIF 01180 #define LWIP_HAVE_SLIPIF 0 01181 #endif 01182 01183 /* 01184 ------------------------------------ 01185 ---------- Thread options ---------- 01186 ------------------------------------ 01187 */ 01188 /** 01189 * TCPIP_THREAD_NAME: The name assigned to the main tcpip thread. 01190 */ 01191 #ifndef TCPIP_THREAD_NAME 01192 #define TCPIP_THREAD_NAME "tcpip_thread" 01193 #endif 01194 01195 /** 01196 * TCPIP_THREAD_STACKSIZE: The stack size used by the main tcpip thread. 01197 * The stack size value itself is platform-dependent, but is passed to 01198 * sys_thread_new() when the thread is created. 01199 */ 01200 #ifndef TCPIP_THREAD_STACKSIZE 01201 #define TCPIP_THREAD_STACKSIZE 0 01202 #endif 01203 01204 /** 01205 * TCPIP_THREAD_PRIO: The priority assigned to the main tcpip thread. 01206 * The priority value itself is platform-dependent, but is passed to 01207 * sys_thread_new() when the thread is created. 01208 */ 01209 #ifndef TCPIP_THREAD_PRIO 01210 #define TCPIP_THREAD_PRIO 1 01211 #endif 01212 01213 /** 01214 * TCPIP_MBOX_SIZE: The mailbox size for the tcpip thread messages 01215 * The queue size value itself is platform-dependent, but is passed to 01216 * sys_mbox_new() when tcpip_init is called. 01217 */ 01218 #ifndef TCPIP_MBOX_SIZE 01219 #define TCPIP_MBOX_SIZE 0 01220 #endif 01221 01222 /** 01223 * SLIPIF_THREAD_NAME: The name assigned to the slipif_loop thread. 01224 */ 01225 #ifndef SLIPIF_THREAD_NAME 01226 #define SLIPIF_THREAD_NAME "slipif_loop" 01227 #endif 01228 01229 /** 01230 * SLIP_THREAD_STACKSIZE: The stack size used by the slipif_loop thread. 01231 * The stack size value itself is platform-dependent, but is passed to 01232 * sys_thread_new() when the thread is created. 01233 */ 01234 #ifndef SLIPIF_THREAD_STACKSIZE 01235 #define SLIPIF_THREAD_STACKSIZE 0 01236 #endif 01237 01238 /** 01239 * SLIPIF_THREAD_PRIO: The priority assigned to the slipif_loop thread. 01240 * The priority value itself is platform-dependent, but is passed to 01241 * sys_thread_new() when the thread is created. 01242 */ 01243 #ifndef SLIPIF_THREAD_PRIO 01244 #define SLIPIF_THREAD_PRIO 1 01245 #endif 01246 01247 /** 01248 * PPP_THREAD_NAME: The name assigned to the pppInputThread. 01249 */ 01250 #ifndef PPP_THREAD_NAME 01251 #define PPP_THREAD_NAME "pppInputThread" 01252 #endif 01253 01254 /** 01255 * PPP_THREAD_STACKSIZE: The stack size used by the pppInputThread. 01256 * The stack size value itself is platform-dependent, but is passed to 01257 * sys_thread_new() when the thread is created. 01258 */ 01259 #ifndef PPP_THREAD_STACKSIZE 01260 #define PPP_THREAD_STACKSIZE 0 01261 #endif 01262 01263 /** 01264 * PPP_THREAD_PRIO: The priority assigned to the pppInputThread. 01265 * The priority value itself is platform-dependent, but is passed to 01266 * sys_thread_new() when the thread is created. 01267 */ 01268 #ifndef PPP_THREAD_PRIO 01269 #define PPP_THREAD_PRIO 1 01270 #endif 01271 01272 /** 01273 * DEFAULT_THREAD_NAME: The name assigned to any other lwIP thread. 01274 */ 01275 #ifndef DEFAULT_THREAD_NAME 01276 #define DEFAULT_THREAD_NAME "lwIP" 01277 #endif 01278 01279 /** 01280 * DEFAULT_THREAD_STACKSIZE: The stack size used by any other lwIP thread. 01281 * The stack size value itself is platform-dependent, but is passed to 01282 * sys_thread_new() when the thread is created. 01283 */ 01284 #ifndef DEFAULT_THREAD_STACKSIZE 01285 #define DEFAULT_THREAD_STACKSIZE 0 01286 #endif 01287 01288 /** 01289 * DEFAULT_THREAD_PRIO: The priority assigned to any other lwIP thread. 01290 * The priority value itself is platform-dependent, but is passed to 01291 * sys_thread_new() when the thread is created. 01292 */ 01293 #ifndef DEFAULT_THREAD_PRIO 01294 #define DEFAULT_THREAD_PRIO 1 01295 #endif 01296 01297 /** 01298 * DEFAULT_RAW_RECVMBOX_SIZE: The mailbox size for the incoming packets on a 01299 * NETCONN_RAW. The queue size value itself is platform-dependent, but is passed 01300 * to sys_mbox_new() when the recvmbox is created. 01301 */ 01302 #ifndef DEFAULT_RAW_RECVMBOX_SIZE 01303 #define DEFAULT_RAW_RECVMBOX_SIZE 0 01304 #endif 01305 01306 /** 01307 * DEFAULT_UDP_RECVMBOX_SIZE: The mailbox size for the incoming packets on a 01308 * NETCONN_UDP. The queue size value itself is platform-dependent, but is passed 01309 * to sys_mbox_new() when the recvmbox is created. 01310 */ 01311 #ifndef DEFAULT_UDP_RECVMBOX_SIZE 01312 #define DEFAULT_UDP_RECVMBOX_SIZE 0 01313 #endif 01314 01315 /** 01316 * DEFAULT_TCP_RECVMBOX_SIZE: The mailbox size for the incoming packets on a 01317 * NETCONN_TCP. The queue size value itself is platform-dependent, but is passed 01318 * to sys_mbox_new() when the recvmbox is created. 01319 */ 01320 #ifndef DEFAULT_TCP_RECVMBOX_SIZE 01321 #define DEFAULT_TCP_RECVMBOX_SIZE 0 01322 #endif 01323 01324 /** 01325 * DEFAULT_ACCEPTMBOX_SIZE: The mailbox size for the incoming connections. 01326 * The queue size value itself is platform-dependent, but is passed to 01327 * sys_mbox_new() when the acceptmbox is created. 01328 */ 01329 #ifndef DEFAULT_ACCEPTMBOX_SIZE 01330 #define DEFAULT_ACCEPTMBOX_SIZE 0 01331 #endif 01332 01333 /* 01334 ---------------------------------------------- 01335 ---------- Sequential layer options ---------- 01336 ---------------------------------------------- 01337 */ 01338 /** 01339 * LWIP_TCPIP_CORE_LOCKING: (EXPERIMENTAL!) 01340 * Don't use it if you're not an active lwIP project member 01341 */ 01342 #ifndef LWIP_TCPIP_CORE_LOCKING 01343 #define LWIP_TCPIP_CORE_LOCKING 0 01344 #endif 01345 01346 /** 01347 * LWIP_TCPIP_CORE_LOCKING_INPUT: (EXPERIMENTAL!) 01348 * Don't use it if you're not an active lwIP project member 01349 */ 01350 #ifndef LWIP_TCPIP_CORE_LOCKING_INPUT 01351 #define LWIP_TCPIP_CORE_LOCKING_INPUT 0 01352 #endif 01353 01354 /** 01355 * LWIP_NETCONN==1: Enable Netconn API (require to use api_lib.c) 01356 */ 01357 #ifndef LWIP_NETCONN 01358 #define LWIP_NETCONN 1 01359 #endif 01360 01361 /** LWIP_TCPIP_TIMEOUT==1: Enable tcpip_timeout/tcpip_untimeout tod create 01362 * timers running in tcpip_thread from another thread. 01363 */ 01364 #ifndef LWIP_TCPIP_TIMEOUT 01365 #define LWIP_TCPIP_TIMEOUT 1 01366 #endif 01367 01368 /* 01369 ------------------------------------ 01370 ---------- Socket options ---------- 01371 ------------------------------------ 01372 */ 01373 /** 01374 * LWIP_SOCKET==1: Enable Socket API (require to use sockets.c) 01375 */ 01376 #ifndef LWIP_SOCKET 01377 #define LWIP_SOCKET 1 01378 #endif 01379 01380 /** 01381 * LWIP_COMPAT_SOCKETS==1: Enable BSD-style sockets functions names. 01382 * (only used if you use sockets.c) 01383 */ 01384 #ifndef LWIP_COMPAT_SOCKETS 01385 #define LWIP_COMPAT_SOCKETS 1 01386 #endif 01387 01388 /** 01389 * LWIP_POSIX_SOCKETS_IO_NAMES==1: Enable POSIX-style sockets functions names. 01390 * Disable this option if you use a POSIX operating system that uses the same 01391 * names (read, write & close). (only used if you use sockets.c) 01392 */ 01393 #ifndef LWIP_POSIX_SOCKETS_IO_NAMES 01394 #define LWIP_POSIX_SOCKETS_IO_NAMES 1 01395 #endif 01396 01397 /** 01398 * LWIP_TCP_KEEPALIVE==1: Enable TCP_KEEPIDLE, TCP_KEEPINTVL and TCP_KEEPCNT 01399 * options processing. Note that TCP_KEEPIDLE and TCP_KEEPINTVL have to be set 01400 * in seconds. (does not require sockets.c, and will affect tcp.c) 01401 */ 01402 #ifndef LWIP_TCP_KEEPALIVE 01403 #define LWIP_TCP_KEEPALIVE 0 01404 #endif 01405 01406 /** 01407 * LWIP_SO_RCVTIMEO==1: Enable SO_RCVTIMEO processing. 01408 */ 01409 #ifndef LWIP_SO_RCVTIMEO 01410 #define LWIP_SO_RCVTIMEO 0 01411 #endif 01412 01413 /** 01414 * LWIP_SO_RCVBUF==1: Enable SO_RCVBUF processing. 01415 */ 01416 #ifndef LWIP_SO_RCVBUF 01417 #define LWIP_SO_RCVBUF 0 01418 #endif 01419 01420 /** 01421 * If LWIP_SO_RCVBUF is used, this is the default value for recv_bufsize. 01422 */ 01423 #ifndef RECV_BUFSIZE_DEFAULT 01424 #define RECV_BUFSIZE_DEFAULT INT_MAX 01425 #endif 01426 01427 /** 01428 * SO_REUSE==1: Enable SO_REUSEADDR option. 01429 */ 01430 #ifndef SO_REUSE 01431 #define SO_REUSE 0 01432 #endif 01433 01434 /** 01435 * SO_REUSE_RXTOALL==1: Pass a copy of incoming broadcast/multicast packets 01436 * to all local matches if SO_REUSEADDR is turned on. 01437 * WARNING: Adds a memcpy for every packet if passing to more than one pcb! 01438 */ 01439 #ifndef SO_REUSE_RXTOALL 01440 #define SO_REUSE_RXTOALL 0 01441 #endif 01442 01443 /* 01444 ---------------------------------------- 01445 ---------- Statistics options ---------- 01446 ---------------------------------------- 01447 */ 01448 /** 01449 * LWIP_STATS==1: Enable statistics collection in lwip_stats. 01450 */ 01451 #ifndef LWIP_STATS 01452 #define LWIP_STATS 1 01453 #endif 01454 01455 #if LWIP_STATS 01456 01457 /** 01458 * LWIP_STATS_DISPLAY==1: Compile in the statistics output functions. 01459 */ 01460 #ifndef LWIP_STATS_DISPLAY 01461 #define LWIP_STATS_DISPLAY 0 01462 #endif 01463 01464 /** 01465 * LINK_STATS==1: Enable link stats. 01466 */ 01467 #ifndef LINK_STATS 01468 #define LINK_STATS 1 01469 #endif 01470 01471 /** 01472 * ETHARP_STATS==1: Enable etharp stats. 01473 */ 01474 #ifndef ETHARP_STATS 01475 #define ETHARP_STATS (LWIP_ARP) 01476 #endif 01477 01478 /** 01479 * IP_STATS==1: Enable IP stats. 01480 */ 01481 #ifndef IP_STATS 01482 #define IP_STATS 1 01483 #endif 01484 01485 /** 01486 * IPFRAG_STATS==1: Enable IP fragmentation stats. Default is 01487 * on if using either frag or reass. 01488 */ 01489 #ifndef IPFRAG_STATS 01490 #define IPFRAG_STATS (IP_REASSEMBLY || IP_FRAG) 01491 #endif 01492 01493 /** 01494 * ICMP_STATS==1: Enable ICMP stats. 01495 */ 01496 #ifndef ICMP_STATS 01497 #define ICMP_STATS 1 01498 #endif 01499 01500 /** 01501 * IGMP_STATS==1: Enable IGMP stats. 01502 */ 01503 #ifndef IGMP_STATS 01504 #define IGMP_STATS (LWIP_IGMP) 01505 #endif 01506 01507 /** 01508 * UDP_STATS==1: Enable UDP stats. Default is on if 01509 * UDP enabled, otherwise off. 01510 */ 01511 #ifndef UDP_STATS 01512 #define UDP_STATS (LWIP_UDP) 01513 #endif 01514 01515 /** 01516 * TCP_STATS==1: Enable TCP stats. Default is on if TCP 01517 * enabled, otherwise off. 01518 */ 01519 #ifndef TCP_STATS 01520 #define TCP_STATS (LWIP_TCP) 01521 #endif 01522 01523 /** 01524 * MEM_STATS==1: Enable mem.c stats. 01525 */ 01526 #ifndef MEM_STATS 01527 #define MEM_STATS ((MEM_LIBC_MALLOC == 0) && (MEM_USE_POOLS == 0)) 01528 #endif 01529 01530 /** 01531 * MEMP_STATS==1: Enable memp.c pool stats. 01532 */ 01533 #ifndef MEMP_STATS 01534 #define MEMP_STATS (MEMP_MEM_MALLOC == 0) 01535 #endif 01536 01537 /** 01538 * SYS_STATS==1: Enable system stats (sem and mbox counts, etc). 01539 */ 01540 #ifndef SYS_STATS 01541 #define SYS_STATS (NO_SYS == 0) 01542 #endif 01543 01544 #else 01545 01546 #define LINK_STATS 0 01547 #define IP_STATS 0 01548 #define IPFRAG_STATS 0 01549 #define ICMP_STATS 0 01550 #define IGMP_STATS 0 01551 #define UDP_STATS 0 01552 #define TCP_STATS 0 01553 #define MEM_STATS 0 01554 #define MEMP_STATS 0 01555 #define SYS_STATS 0 01556 #define LWIP_STATS_DISPLAY 0 01557 01558 #endif /* LWIP_STATS */ 01559 01560 /* 01561 --------------------------------- 01562 ---------- PPP options ---------- 01563 --------------------------------- 01564 */ 01565 /** 01566 * PPP_SUPPORT==1: Enable PPP. 01567 */ 01568 #ifndef PPP_SUPPORT 01569 #define PPP_SUPPORT 0 01570 #endif 01571 01572 /** 01573 * PPPOE_SUPPORT==1: Enable PPP Over Ethernet 01574 */ 01575 #ifndef PPPOE_SUPPORT 01576 #define PPPOE_SUPPORT 0 01577 #endif 01578 01579 /** 01580 * PPPOS_SUPPORT==1: Enable PPP Over Serial 01581 */ 01582 #ifndef PPPOS_SUPPORT 01583 #define PPPOS_SUPPORT PPP_SUPPORT 01584 #endif 01585 01586 #if PPP_SUPPORT 01587 01588 /** 01589 * NUM_PPP: Max PPP sessions. 01590 */ 01591 #ifndef NUM_PPP 01592 #define NUM_PPP 1 01593 #endif 01594 01595 /** 01596 * PAP_SUPPORT==1: Support PAP. 01597 */ 01598 #ifndef PAP_SUPPORT 01599 #define PAP_SUPPORT 0 01600 #endif 01601 01602 /** 01603 * CHAP_SUPPORT==1: Support CHAP. 01604 */ 01605 #ifndef CHAP_SUPPORT 01606 #define CHAP_SUPPORT 0 01607 #endif 01608 01609 /** 01610 * MSCHAP_SUPPORT==1: Support MSCHAP. CURRENTLY NOT SUPPORTED! DO NOT SET! 01611 */ 01612 #ifndef MSCHAP_SUPPORT 01613 #define MSCHAP_SUPPORT 0 01614 #endif 01615 01616 /** 01617 * CBCP_SUPPORT==1: Support CBCP. CURRENTLY NOT SUPPORTED! DO NOT SET! 01618 */ 01619 #ifndef CBCP_SUPPORT 01620 #define CBCP_SUPPORT 0 01621 #endif 01622 01623 /** 01624 * CCP_SUPPORT==1: Support CCP. CURRENTLY NOT SUPPORTED! DO NOT SET! 01625 */ 01626 #ifndef CCP_SUPPORT 01627 #define CCP_SUPPORT 0 01628 #endif 01629 01630 /** 01631 * VJ_SUPPORT==1: Support VJ header compression. 01632 */ 01633 #ifndef VJ_SUPPORT 01634 #define VJ_SUPPORT 0 01635 #endif 01636 01637 /** 01638 * MD5_SUPPORT==1: Support MD5 (see also CHAP). 01639 */ 01640 #ifndef MD5_SUPPORT 01641 #define MD5_SUPPORT 0 01642 #endif 01643 01644 /* 01645 * Timeouts 01646 */ 01647 #ifndef FSM_DEFTIMEOUT 01648 #define FSM_DEFTIMEOUT 6 /* Timeout time in seconds */ 01649 #endif 01650 01651 #ifndef FSM_DEFMAXTERMREQS 01652 #define FSM_DEFMAXTERMREQS 2 /* Maximum Terminate-Request transmissions */ 01653 #endif 01654 01655 #ifndef FSM_DEFMAXCONFREQS 01656 #define FSM_DEFMAXCONFREQS 10 /* Maximum Configure-Request transmissions */ 01657 #endif 01658 01659 #ifndef FSM_DEFMAXNAKLOOPS 01660 #define FSM_DEFMAXNAKLOOPS 5 /* Maximum number of nak loops */ 01661 #endif 01662 01663 #ifndef UPAP_DEFTIMEOUT 01664 #define UPAP_DEFTIMEOUT 6 /* Timeout (seconds) for retransmitting req */ 01665 #endif 01666 01667 #ifndef UPAP_DEFREQTIME 01668 #define UPAP_DEFREQTIME 30 /* Time to wait for auth-req from peer */ 01669 #endif 01670 01671 #ifndef CHAP_DEFTIMEOUT 01672 #define CHAP_DEFTIMEOUT 6 /* Timeout time in seconds */ 01673 #endif 01674 01675 #ifndef CHAP_DEFTRANSMITS 01676 #define CHAP_DEFTRANSMITS 10 /* max # times to send challenge */ 01677 #endif 01678 01679 /* Interval in seconds between keepalive echo requests, 0 to disable. */ 01680 #ifndef LCP_ECHOINTERVAL 01681 #define LCP_ECHOINTERVAL 0 01682 #endif 01683 01684 /* Number of unanswered echo requests before failure. */ 01685 #ifndef LCP_MAXECHOFAILS 01686 #define LCP_MAXECHOFAILS 3 01687 #endif 01688 01689 /* Max Xmit idle time (in jiffies) before resend flag char. */ 01690 #ifndef PPP_MAXIDLEFLAG 01691 #define PPP_MAXIDLEFLAG 100 01692 #endif 01693 01694 /* 01695 * Packet sizes 01696 * 01697 * Note - lcp shouldn't be allowed to negotiate stuff outside these 01698 * limits. See lcp.h in the pppd directory. 01699 * (XXX - these constants should simply be shared by lcp.c instead 01700 * of living in lcp.h) 01701 */ 01702 #define PPP_MTU 1500 /* Default MTU (size of Info field) */ 01703 #ifndef PPP_MAXMTU 01704 /* #define PPP_MAXMTU 65535 - (PPP_HDRLEN + PPP_FCSLEN) */ 01705 #define PPP_MAXMTU 1500 /* Largest MTU we allow */ 01706 #endif 01707 #define PPP_MINMTU 64 01708 #define PPP_MRU 1500 /* default MRU = max length of info field */ 01709 #define PPP_MAXMRU 1500 /* Largest MRU we allow */ 01710 #ifndef PPP_DEFMRU 01711 #define PPP_DEFMRU 296 /* Try for this */ 01712 #endif 01713 #define PPP_MINMRU 128 /* No MRUs below this */ 01714 01715 #ifndef MAXNAMELEN 01716 #define MAXNAMELEN 256 /* max length of hostname or name for auth */ 01717 #endif 01718 #ifndef MAXSECRETLEN 01719 #define MAXSECRETLEN 256 /* max length of password or secret */ 01720 #endif 01721 01722 #endif /* PPP_SUPPORT */ 01723 01724 /* 01725 -------------------------------------- 01726 ---------- Checksum options ---------- 01727 -------------------------------------- 01728 */ 01729 /** 01730 * CHECKSUM_GEN_IP==1: Generate checksums in software for outgoing IP packets. 01731 */ 01732 #ifndef CHECKSUM_GEN_IP 01733 #define CHECKSUM_GEN_IP 1 01734 #endif 01735 01736 /** 01737 * CHECKSUM_GEN_UDP==1: Generate checksums in software for outgoing UDP packets. 01738 */ 01739 #ifndef CHECKSUM_GEN_UDP 01740 #define CHECKSUM_GEN_UDP 1 01741 #endif 01742 01743 /** 01744 * CHECKSUM_GEN_TCP==1: Generate checksums in software for outgoing TCP packets. 01745 */ 01746 #ifndef CHECKSUM_GEN_TCP 01747 #define CHECKSUM_GEN_TCP 1 01748 #endif 01749 01750 /** 01751 * CHECKSUM_CHECK_IP==1: Check checksums in software for incoming IP packets. 01752 */ 01753 #ifndef CHECKSUM_CHECK_IP 01754 #define CHECKSUM_CHECK_IP 1 01755 #endif 01756 01757 /** 01758 * CHECKSUM_CHECK_UDP==1: Check checksums in software for incoming UDP packets. 01759 */ 01760 #ifndef CHECKSUM_CHECK_UDP 01761 #define CHECKSUM_CHECK_UDP 1 01762 #endif 01763 01764 /** 01765 * CHECKSUM_CHECK_TCP==1: Check checksums in software for incoming TCP packets. 01766 */ 01767 #ifndef CHECKSUM_CHECK_TCP 01768 #define CHECKSUM_CHECK_TCP 1 01769 #endif 01770 01771 /** 01772 * LWIP_CHECKSUM_ON_COPY==1: Calculate checksum when copying data from 01773 * application buffers to pbufs. 01774 */ 01775 #ifndef LWIP_CHECKSUM_ON_COPY 01776 #define LWIP_CHECKSUM_ON_COPY 0 01777 #endif 01778 01779 /* 01780 --------------------------------------- 01781 ---------- Debugging options ---------- 01782 --------------------------------------- 01783 */ 01784 /** 01785 * LWIP_DBG_MIN_LEVEL: After masking, the value of the debug is 01786 * compared against this value. If it is smaller, then debugging 01787 * messages are written. 01788 */ 01789 #ifndef LWIP_DBG_MIN_LEVEL 01790 #define LWIP_DBG_MIN_LEVEL LWIP_DBG_LEVEL_ALL 01791 #endif 01792 01793 /** 01794 * LWIP_DBG_TYPES_ON: A mask that can be used to globally enable/disable 01795 * debug messages of certain types. 01796 */ 01797 #ifndef LWIP_DBG_TYPES_ON 01798 #define LWIP_DBG_TYPES_ON LWIP_DBG_ON 01799 #endif 01800 01801 /** 01802 * ETHARP_DEBUG: Enable debugging in etharp.c. 01803 */ 01804 #ifndef ETHARP_DEBUG 01805 #define ETHARP_DEBUG LWIP_DBG_OFF 01806 #endif 01807 01808 /** 01809 * NETIF_DEBUG: Enable debugging in netif.c. 01810 */ 01811 #ifndef NETIF_DEBUG 01812 #define NETIF_DEBUG LWIP_DBG_OFF 01813 #endif 01814 01815 /** 01816 * PBUF_DEBUG: Enable debugging in pbuf.c. 01817 */ 01818 #ifndef PBUF_DEBUG 01819 #define PBUF_DEBUG LWIP_DBG_OFF 01820 #endif 01821 01822 /** 01823 * API_LIB_DEBUG: Enable debugging in api_lib.c. 01824 */ 01825 #ifndef API_LIB_DEBUG 01826 #define API_LIB_DEBUG LWIP_DBG_OFF 01827 #endif 01828 01829 /** 01830 * API_MSG_DEBUG: Enable debugging in api_msg.c. 01831 */ 01832 #ifndef API_MSG_DEBUG 01833 #define API_MSG_DEBUG LWIP_DBG_OFF 01834 #endif 01835 01836 /** 01837 * SOCKETS_DEBUG: Enable debugging in sockets.c. 01838 */ 01839 #ifndef SOCKETS_DEBUG 01840 #define SOCKETS_DEBUG LWIP_DBG_OFF 01841 #endif 01842 01843 /** 01844 * ICMP_DEBUG: Enable debugging in icmp.c. 01845 */ 01846 #ifndef ICMP_DEBUG 01847 #define ICMP_DEBUG LWIP_DBG_OFF 01848 #endif 01849 01850 /** 01851 * IGMP_DEBUG: Enable debugging in igmp.c. 01852 */ 01853 #ifndef IGMP_DEBUG 01854 #define IGMP_DEBUG LWIP_DBG_OFF 01855 #endif 01856 01857 /** 01858 * INET_DEBUG: Enable debugging in inet.c. 01859 */ 01860 #ifndef INET_DEBUG 01861 #define INET_DEBUG LWIP_DBG_OFF 01862 #endif 01863 01864 /** 01865 * IP_DEBUG: Enable debugging for IP. 01866 */ 01867 #ifndef IP_DEBUG 01868 #define IP_DEBUG LWIP_DBG_OFF 01869 #endif 01870 01871 /** 01872 * IP_REASS_DEBUG: Enable debugging in ip_frag.c for both frag & reass. 01873 */ 01874 #ifndef IP_REASS_DEBUG 01875 #define IP_REASS_DEBUG LWIP_DBG_OFF 01876 #endif 01877 01878 /** 01879 * RAW_DEBUG: Enable debugging in raw.c. 01880 */ 01881 #ifndef RAW_DEBUG 01882 #define RAW_DEBUG LWIP_DBG_OFF 01883 #endif 01884 01885 /** 01886 * MEM_DEBUG: Enable debugging in mem.c. 01887 */ 01888 #ifndef MEM_DEBUG 01889 #define MEM_DEBUG LWIP_DBG_OFF 01890 #endif 01891 01892 /** 01893 * MEMP_DEBUG: Enable debugging in memp.c. 01894 */ 01895 #ifndef MEMP_DEBUG 01896 #define MEMP_DEBUG LWIP_DBG_OFF 01897 #endif 01898 01899 /** 01900 * SYS_DEBUG: Enable debugging in sys.c. 01901 */ 01902 #ifndef SYS_DEBUG 01903 #define SYS_DEBUG LWIP_DBG_OFF 01904 #endif 01905 01906 /** 01907 * TIMERS_DEBUG: Enable debugging in timers.c. 01908 */ 01909 #ifndef TIMERS_DEBUG 01910 #define TIMERS_DEBUG LWIP_DBG_OFF 01911 #endif 01912 01913 /** 01914 * TCP_DEBUG: Enable debugging for TCP. 01915 */ 01916 #ifndef TCP_DEBUG 01917 #define TCP_DEBUG LWIP_DBG_OFF 01918 #endif 01919 01920 /** 01921 * TCP_INPUT_DEBUG: Enable debugging in tcp_in.c for incoming debug. 01922 */ 01923 #ifndef TCP_INPUT_DEBUG 01924 #define TCP_INPUT_DEBUG LWIP_DBG_OFF 01925 #endif 01926 01927 /** 01928 * TCP_FR_DEBUG: Enable debugging in tcp_in.c for fast retransmit. 01929 */ 01930 #ifndef TCP_FR_DEBUG 01931 #define TCP_FR_DEBUG LWIP_DBG_OFF 01932 #endif 01933 01934 /** 01935 * TCP_RTO_DEBUG: Enable debugging in TCP for retransmit 01936 * timeout. 01937 */ 01938 #ifndef TCP_RTO_DEBUG 01939 #define TCP_RTO_DEBUG LWIP_DBG_OFF 01940 #endif 01941 01942 /** 01943 * TCP_CWND_DEBUG: Enable debugging for TCP congestion window. 01944 */ 01945 #ifndef TCP_CWND_DEBUG 01946 #define TCP_CWND_DEBUG LWIP_DBG_OFF 01947 #endif 01948 01949 /** 01950 * TCP_WND_DEBUG: Enable debugging in tcp_in.c for window updating. 01951 */ 01952 #ifndef TCP_WND_DEBUG 01953 #define TCP_WND_DEBUG LWIP_DBG_OFF 01954 #endif 01955 01956 /** 01957 * TCP_OUTPUT_DEBUG: Enable debugging in tcp_out.c output functions. 01958 */ 01959 #ifndef TCP_OUTPUT_DEBUG 01960 #define TCP_OUTPUT_DEBUG LWIP_DBG_OFF 01961 #endif 01962 01963 /** 01964 * TCP_RST_DEBUG: Enable debugging for TCP with the RST message. 01965 */ 01966 #ifndef TCP_RST_DEBUG 01967 #define TCP_RST_DEBUG LWIP_DBG_OFF 01968 #endif 01969 01970 /** 01971 * TCP_QLEN_DEBUG: Enable debugging for TCP queue lengths. 01972 */ 01973 #ifndef TCP_QLEN_DEBUG 01974 #define TCP_QLEN_DEBUG LWIP_DBG_OFF 01975 #endif 01976 01977 /** 01978 * UDP_DEBUG: Enable debugging in UDP. 01979 */ 01980 #ifndef UDP_DEBUG 01981 #define UDP_DEBUG LWIP_DBG_OFF 01982 #endif 01983 01984 /** 01985 * TCPIP_DEBUG: Enable debugging in tcpip.c. 01986 */ 01987 #ifndef TCPIP_DEBUG 01988 #define TCPIP_DEBUG LWIP_DBG_OFF 01989 #endif 01990 01991 /** 01992 * PPP_DEBUG: Enable debugging for PPP. 01993 */ 01994 #ifndef PPP_DEBUG 01995 #define PPP_DEBUG LWIP_DBG_OFF 01996 #endif 01997 01998 /** 01999 * SLIP_DEBUG: Enable debugging in slipif.c. 02000 */ 02001 #ifndef SLIP_DEBUG 02002 #define SLIP_DEBUG LWIP_DBG_OFF 02003 #endif 02004 02005 /** 02006 * DHCP_DEBUG: Enable debugging in dhcp.c. 02007 */ 02008 #ifndef DHCP_DEBUG 02009 #define DHCP_DEBUG LWIP_DBG_OFF 02010 #endif 02011 02012 /** 02013 * AUTOIP_DEBUG: Enable debugging in autoip.c. 02014 */ 02015 #ifndef AUTOIP_DEBUG 02016 #define AUTOIP_DEBUG LWIP_DBG_OFF 02017 #endif 02018 02019 /** 02020 * SNMP_MSG_DEBUG: Enable debugging for SNMP messages. 02021 */ 02022 #ifndef SNMP_MSG_DEBUG 02023 #define SNMP_MSG_DEBUG LWIP_DBG_OFF 02024 #endif 02025 02026 /** 02027 * SNMP_MIB_DEBUG: Enable debugging for SNMP MIBs. 02028 */ 02029 #ifndef SNMP_MIB_DEBUG 02030 #define SNMP_MIB_DEBUG LWIP_DBG_OFF 02031 #endif 02032 02033 /** 02034 * DNS_DEBUG: Enable debugging for DNS. 02035 */ 02036 #ifndef DNS_DEBUG 02037 #define DNS_DEBUG LWIP_DBG_OFF 02038 #endif 02039 02040 #endif /* __LWIP_OPT_H__ */
Generated on Tue Jul 12 2022 11:52:58 by
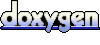