NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
netudpsocket.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef NETUDPSOCKET_H 00025 #define NETUDPSOCKET_H 00026 00027 #include "net.h" 00028 #include "host.h" 00029 00030 #include <queue> 00031 using std::queue; 00032 00033 //Implements a Berkeley-like socket if 00034 //Can be interfaced either to lwip or a Telit module 00035 00036 enum NetUdpSocketErr 00037 { 00038 __NETUDPSOCKET_MIN = -0xFFFF, 00039 NETUDPSOCKET_SETUP, //NetUdpSocket not properly configured 00040 NETUDPSOCKET_IF, //If has problems 00041 NETUDPSOCKET_MEM, //Not enough mem 00042 NETUDPSOCKET_INUSE, //If/Port is in use 00043 //... 00044 NETUDPSOCKET_OK = 0 00045 }; 00046 00047 enum NetUdpSocketEvent //Only one lonely event here... but who knows, maybe some day there'll be another one! 00048 { 00049 NETUDPSOCKET_READABLE, //Data in buf 00050 }; 00051 00052 00053 class NetUdpSocket 00054 { 00055 public: 00056 NetUdpSocket(); 00057 virtual ~NetUdpSocket(); //close() 00058 00059 virtual NetUdpSocketErr bind(const Host& me) = 0; 00060 00061 virtual int /*if < 0 : NetUdpSocketErr*/ sendto(const char* buf, int len, Host* pHost) = 0; 00062 virtual int /*if < 0 : NetUdpSocketErr*/ recvfrom(char* buf, int len, Host* pHost) = 0; 00063 00064 /* TODO NTH : printf / scanf helpers that call send/recv */ 00065 00066 virtual NetUdpSocketErr close() = 0; 00067 00068 virtual NetUdpSocketErr poll() = 0; 00069 00070 class CDummy; 00071 //Callbacks 00072 template<class T> 00073 //Linker bug : Must be defined here :( 00074 void setOnEvent( T* pItem, void (T::*pMethod)(NetUdpSocketEvent) ) 00075 { 00076 m_pCbItem = (CDummy*) pItem; 00077 m_pCbMeth = (void (CDummy::*)(NetUdpSocketEvent)) pMethod; 00078 } 00079 00080 void resetOnEvent(); //Disable callback 00081 00082 protected: 00083 void queueEvent(NetUdpSocketEvent e); 00084 void discardEvents(); 00085 void flushEvents(); //to be called during polling 00086 00087 Host m_host; 00088 Host m_client; 00089 00090 friend class Net; 00091 int m_refs; 00092 00093 bool m_closed; 00094 bool m_removed; 00095 00096 private: 00097 //We do not want to execute user code in interrupt routines, so we queue events until the server is polled 00098 //If we port this to a multithreaded OS, we could avoid this (however some functions here are not thread-safe, so beware ;) ) 00099 void onEvent(NetUdpSocketEvent e); //To be called on poll 00100 CDummy* m_pCbItem; 00101 void (CDummy::*m_pCbMeth)(NetUdpSocketEvent); 00102 queue<NetUdpSocketEvent> m_events; 00103 00104 }; 00105 00106 #endif
Generated on Tue Jul 12 2022 11:52:58 by
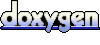