NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
net.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "net.h" 00025 00026 #include "host.h" 00027 #include "ipaddr.h" 00028 #include "netservice.h" 00029 #include "if/net/netif.h" 00030 #include "if/net/nettcpsocket.h" 00031 #include "if/net/netudpsocket.h" 00032 #include "if/net/netdnsrequest.h" 00033 00034 //#define __DEBUG 00035 #include "dbg/dbg.h" 00036 00037 Net::Net() : m_defaultIf(NULL), m_lpIf(), m_lpNetTcpSocket(), m_lpNetUdpSocket() 00038 { 00039 00040 } 00041 00042 Net::~Net() 00043 { 00044 00045 } 00046 00047 00048 void Net::poll() 00049 { 00050 //DBG("\r\nNet : Services polling\r\n"); 00051 00052 //Poll Services 00053 NetService::servicesPoll(); 00054 00055 //DBG("\r\nNet : Interfaces polling\r\n"); 00056 00057 //Poll Interfaces 00058 list<NetIf*>::iterator pIfIt; 00059 00060 for ( pIfIt = net().m_lpIf.begin() ; pIfIt != net().m_lpIf.end(); pIfIt++ ) 00061 { 00062 (*pIfIt)->poll(); 00063 } 00064 00065 //DBG("\r\nNet : Sockets polling\r\n"); 00066 00067 //Poll Tcp Sockets 00068 list<NetTcpSocket*>::iterator pNetTcpSocketIt; 00069 00070 for ( pNetTcpSocketIt = net().m_lpNetTcpSocket.begin() ; pNetTcpSocketIt != net().m_lpNetTcpSocket.end(); ) 00071 { 00072 (*pNetTcpSocketIt)->poll(); 00073 00074 if( (*pNetTcpSocketIt)->m_closed && !((*pNetTcpSocketIt)->m_refs) ) 00075 { 00076 (*pNetTcpSocketIt)->m_removed = true; 00077 delete (*pNetTcpSocketIt); 00078 (*pNetTcpSocketIt) = NULL; 00079 pNetTcpSocketIt = net().m_lpNetTcpSocket.erase(pNetTcpSocketIt); 00080 } 00081 else 00082 { 00083 pNetTcpSocketIt++; 00084 } 00085 } 00086 00087 //Poll Udp Sockets 00088 list<NetUdpSocket*>::iterator pNetUdpSocketIt; 00089 00090 for ( pNetUdpSocketIt = net().m_lpNetUdpSocket.begin() ; pNetUdpSocketIt != net().m_lpNetUdpSocket.end(); ) 00091 { 00092 (*pNetUdpSocketIt)->poll(); 00093 00094 if( (*pNetUdpSocketIt)->m_closed && !((*pNetUdpSocketIt)->m_refs) ) 00095 { 00096 (*pNetUdpSocketIt)->m_removed = true; 00097 delete (*pNetUdpSocketIt); 00098 (*pNetUdpSocketIt) = NULL; 00099 pNetUdpSocketIt = net().m_lpNetUdpSocket.erase(pNetUdpSocketIt); 00100 } 00101 else 00102 { 00103 pNetUdpSocketIt++; 00104 } 00105 } 00106 00107 00108 } 00109 00110 NetTcpSocket* Net::tcpSocket(NetIf& netif) { 00111 NetTcpSocket* pNetTcpSocket = netif.tcpSocket(); 00112 pNetTcpSocket->m_refs++; 00113 return pNetTcpSocket; 00114 } 00115 00116 NetTcpSocket* Net::tcpSocket() { //NetTcpSocket on default if 00117 if ( net().m_defaultIf == NULL ) 00118 return NULL; 00119 NetTcpSocket* pNetTcpSocket = net().m_defaultIf->tcpSocket(); 00120 pNetTcpSocket->m_refs++; 00121 return pNetTcpSocket; 00122 } 00123 00124 void Net::releaseTcpSocket(NetTcpSocket* pNetTcpSocket) 00125 { 00126 pNetTcpSocket->m_refs--; 00127 if(!pNetTcpSocket->m_closed && !pNetTcpSocket->m_refs) 00128 pNetTcpSocket->close(); 00129 } 00130 00131 NetUdpSocket* Net::udpSocket(NetIf& netif) { 00132 NetUdpSocket* pNetUdpSocket = netif.udpSocket(); 00133 pNetUdpSocket->m_refs++; 00134 return pNetUdpSocket; 00135 } 00136 00137 NetUdpSocket* Net::udpSocket() { //NetTcpSocket on default if 00138 if ( net().m_defaultIf == NULL ) 00139 return NULL; 00140 NetUdpSocket* pNetUdpSocket = net().m_defaultIf->udpSocket(); 00141 pNetUdpSocket->m_refs++; 00142 return pNetUdpSocket; 00143 } 00144 00145 void Net::releaseUdpSocket(NetUdpSocket* pNetUdpSocket) 00146 { 00147 pNetUdpSocket->m_refs--; 00148 if(!pNetUdpSocket->m_closed && !pNetUdpSocket->m_refs) 00149 pNetUdpSocket->close(); 00150 } 00151 00152 NetDnsRequest* Net::dnsRequest(const char* hostname, NetIf& netif) { 00153 return netif.dnsRequest(hostname); 00154 } 00155 00156 NetDnsRequest* Net::dnsRequest(const char* hostname) { //Create a new NetDnsRequest object from default if 00157 if ( net().m_defaultIf == NULL ) 00158 return NULL; 00159 return net().m_defaultIf->dnsRequest(hostname); 00160 } 00161 00162 NetDnsRequest* Net::dnsRequest(Host* pHost, NetIf& netif) 00163 { 00164 return netif.dnsRequest(pHost); 00165 } 00166 00167 NetDnsRequest* Net::dnsRequest(Host* pHost) //Creats a new NetDnsRequest object from default if 00168 { 00169 if ( net().m_defaultIf == NULL ) 00170 return NULL; 00171 return net().m_defaultIf->dnsRequest(pHost); 00172 } 00173 00174 void Net::setDefaultIf(NetIf& netif) { 00175 net().m_defaultIf = &netif; 00176 } 00177 00178 void Net::setDefaultIf(NetIf* pIf) 00179 { 00180 net().m_defaultIf = pIf; 00181 } 00182 00183 NetIf* Net::getDefaultIf() { 00184 return net().m_defaultIf; 00185 } 00186 00187 void Net::registerIf(NetIf* pIf) 00188 { 00189 net().m_lpIf.push_back(pIf); 00190 } 00191 00192 void Net::unregisterIf(NetIf* pIf) 00193 { 00194 if( net().m_defaultIf == pIf ) 00195 net().m_defaultIf = NULL; 00196 net().m_lpIf.remove(pIf); 00197 } 00198 00199 void Net::registerNetTcpSocket(NetTcpSocket* pNetTcpSocket) 00200 { 00201 net().m_lpNetTcpSocket.push_back(pNetTcpSocket); 00202 DBG("\r\nNetTcpSocket [ + %p ] %d\r\n", (void*)pNetTcpSocket, net().m_lpNetTcpSocket.size()); 00203 } 00204 00205 void Net::unregisterNetTcpSocket(NetTcpSocket* pNetTcpSocket) 00206 { 00207 DBG("\r\nNetTcpSocket [ - %p ] %d\r\n", (void*)pNetTcpSocket, net().m_lpNetTcpSocket.size() - 1); 00208 if(!pNetTcpSocket->m_removed) 00209 net().m_lpNetTcpSocket.remove(pNetTcpSocket); 00210 } 00211 00212 void Net::registerNetUdpSocket(NetUdpSocket* pNetUdpSocket) 00213 { 00214 net().m_lpNetUdpSocket.push_back(pNetUdpSocket); 00215 DBG("\r\nNetUdpSocket [ + %p ] %d\r\n", (void*)pNetUdpSocket, net().m_lpNetUdpSocket.size()); 00216 } 00217 00218 void Net::unregisterNetUdpSocket(NetUdpSocket* pNetUdpSocket) 00219 { 00220 DBG("\r\nNetUdpSocket [ - %p ] %d\r\n", (void*)pNetUdpSocket, net().m_lpNetUdpSocket.size() - 1); 00221 if(!pNetUdpSocket->m_removed) 00222 net().m_lpNetUdpSocket.remove(pNetUdpSocket); 00223 } 00224 00225 Net& Net::net() 00226 { 00227 static Net* pInst = new Net(); //Called only once 00228 return *pInst; 00229 }
Generated on Tue Jul 12 2022 11:52:58 by
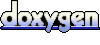