NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
lwipNetTcpSocket.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef LWIPNETTCPSOCKET_H 00025 #define LWIPNETTCPSOCKET_H 00026 00027 #define NET_LWIP_STACK 1 00028 #include "if/net/nettcpsocket.h" 00029 #include "LwipNetIf.h" 00030 00031 #include "stdint.h" 00032 00033 //Implements NetTcpSockets over lwIP raw API 00034 00035 struct tcp_pcb; //Represents a Tcp Connection, "Protocol Control Block", see rawapi.txt & tcp.h 00036 struct pbuf; //Lwip Buffer Container 00037 00038 typedef signed char err_t; 00039 typedef uint16_t u16_t; 00040 00041 class LwipNetTcpSocket: public NetTcpSocket 00042 { 00043 public: 00044 LwipNetTcpSocket(tcp_pcb* pPcb = NULL); //Passes a pcb if already created (by an accept req for instance), in that case transfers ownership 00045 virtual ~LwipNetTcpSocket(); 00046 00047 virtual NetTcpSocketErr bind(const Host& me); 00048 virtual NetTcpSocketErr listen(); 00049 virtual NetTcpSocketErr connect(const Host& host); 00050 virtual NetTcpSocketErr accept(Host* pClient, NetTcpSocket** ppNewNetTcpSocket); 00051 00052 virtual int /*if < 0 : NetTcpSocketErr*/ send(const char* buf, int len); 00053 virtual int /*if < 0 : NetTcpSocketErr*/ recv(char* buf, int len); 00054 00055 virtual NetTcpSocketErr close(); 00056 00057 virtual NetTcpSocketErr poll(); 00058 00059 protected: 00060 volatile tcp_pcb* m_pPcb; 00061 00062 //Events callbacks from lwIp 00063 err_t acceptCb(tcp_pcb* newpcb, err_t err); 00064 err_t connectedCb(tcp_pcb* tpcb, err_t err); 00065 00066 void errCb(err_t err); 00067 00068 err_t sentCb(tcp_pcb* tpcb, u16_t len); 00069 err_t recvCb(tcp_pcb* tpcb, pbuf *p, err_t err); 00070 00071 private: 00072 void cleanUp(); //Flush input buffer 00073 00074 // queue<tcp_pcb*> m_lpInPcb; //Incoming connections that have not been accepted yet 00075 queue<LwipNetTcpSocket*> m_lpInNetTcpSocket; //Incoming connections that have not been accepted yet 00076 00077 volatile pbuf* m_pReadPbuf; //Ptr to read buffer 00078 00079 //Static callbacks : Transforms into a C++ callback 00080 static err_t sAcceptCb(void *arg, struct tcp_pcb *newpcb, err_t err); 00081 static err_t sConnectedCb(void *arg, struct tcp_pcb *tpcb, err_t err); 00082 00083 static void sErrCb(void *arg, err_t err); 00084 00085 static err_t sSentCb(void *arg, struct tcp_pcb *tpcb, u16_t len); 00086 static err_t sRecvCb(void *arg, struct tcp_pcb *tpcb, struct pbuf *p, err_t err); 00087 00088 }; 00089 00090 #endif
Generated on Tue Jul 12 2022 11:52:57 by
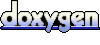