NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
inet.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_INET_H__ 00033 #define __LWIP_INET_H__ 00034 00035 #include "lwip/opt.h" 00036 #include "lwip/def.h" 00037 #include "lwip/ip_addr.h" 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /* For compatibility with BSD code */ 00044 struct in_addr { 00045 u32_t s_addr; 00046 }; 00047 00048 /** 255.255.255.255 */ 00049 #define INADDR_NONE IPADDR_NONE 00050 /** 127.0.0.1 */ 00051 #define INADDR_LOOPBACK IPADDR_LOOPBACK 00052 /** 0.0.0.0 */ 00053 #define INADDR_ANY IPADDR_ANY 00054 /** 255.255.255.255 */ 00055 #define INADDR_BROADCAST IPADDR_BROADCAST 00056 00057 /* Definitions of the bits in an Internet address integer. 00058 00059 On subnets, host and network parts are found according to 00060 the subnet mask, not these masks. */ 00061 #define IN_CLASSA(a) IP_CLASSA(a) 00062 #define IN_CLASSA_NET IP_CLASSA_NET 00063 #define IN_CLASSA_NSHIFT IP_CLASSA_NSHIFT 00064 #define IN_CLASSA_HOST IP_CLASSA_HOST 00065 #define IN_CLASSA_MAX IP_CLASSA_MAX 00066 00067 #define IN_CLASSB(b) IP_CLASSB(b) 00068 #define IN_CLASSB_NET IP_CLASSB_NET 00069 #define IN_CLASSB_NSHIFT IP_CLASSB_NSHIFT 00070 #define IN_CLASSB_HOST IP_CLASSB_HOST 00071 #define IN_CLASSB_MAX IP_CLASSB_MAX 00072 00073 #define IN_CLASSC(c) IP_CLASSC(c) 00074 #define IN_CLASSC_NET IP_CLASSC_NET 00075 #define IN_CLASSC_NSHIFT IP_CLASSC_NSHIFT 00076 #define IN_CLASSC_HOST IP_CLASSC_HOST 00077 #define IN_CLASSC_MAX IP_CLASSC_MAX 00078 00079 #define IN_CLASSD(d) IP_CLASSD(d) 00080 #define IN_CLASSD_NET IP_CLASSD_NET /* These ones aren't really */ 00081 #define IN_CLASSD_NSHIFT IP_CLASSD_NSHIFT /* net and host fields, but */ 00082 #define IN_CLASSD_HOST IP_CLASSD_HOST /* routing needn't know. */ 00083 #define IN_CLASSD_MAX IP_CLASSD_MAX 00084 00085 #define IN_MULTICAST(a) IP_MULTICAST(a) 00086 00087 #define IN_EXPERIMENTAL(a) IP_EXPERIMENTAL(a) 00088 #define IN_BADCLASS(a) IP_BADCLASS(a) 00089 00090 #define IN_LOOPBACKNET IP_LOOPBACKNET 00091 00092 #define inet_addr_from_ipaddr(target_inaddr, source_ipaddr) ((target_inaddr)->s_addr = ip4_addr_get_u32(source_ipaddr)) 00093 #define inet_addr_to_ipaddr(target_ipaddr, source_inaddr) (ip4_addr_set_u32(target_ipaddr, (source_inaddr)->s_addr)) 00094 /* ATTENTION: the next define only works because both s_addr and ip_addr_t are an u32_t effectively! */ 00095 #define inet_addr_to_ipaddr_p(target_ipaddr_p, source_inaddr) ((target_ipaddr_p) = (ip_addr_t*)&((source_inaddr)->s_addr)) 00096 00097 /* directly map this to the lwip internal functions */ 00098 #define inet_addr(cp) ipaddr_addr(cp) 00099 #define inet_aton(cp, addr) ipaddr_aton(cp, (ip_addr_t*)addr) 00100 #define inet_ntoa(addr) ipaddr_ntoa((ip_addr_t*)&(addr)) 00101 #define inet_ntoa_r(addr, buf, buflen) ipaddr_ntoa_r((ip_addr_t*)&(addr), buf, buflen) 00102 00103 #ifdef __cplusplus 00104 } 00105 #endif 00106 00107 #endif /* __LWIP_INET_H__ */
Generated on Tue Jul 12 2022 11:52:57 by
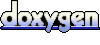