NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
def.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_DEF_H__ 00033 #define __LWIP_DEF_H__ 00034 00035 /* arch.h might define NULL already */ 00036 #include "lwip/arch.h" 00037 #include "lwip/opt.h" 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 #define LWIP_MAX(x , y) (((x) > (y)) ? (x) : (y)) 00044 #define LWIP_MIN(x , y) (((x) < (y)) ? (x) : (y)) 00045 00046 #ifndef NULL 00047 #define NULL ((void *)0) 00048 #endif 00049 00050 /** Get the absolute difference between 2 u32_t values (correcting overflows) 00051 * 'a' is expected to be 'higher' (without overflow) than 'b'. */ 00052 #define LWIP_U32_DIFF(a, b) (((a) >= (b)) ? ((a) - (b)) : (((a) + ((b) ^ 0xFFFFFFFF) + 1))) 00053 00054 /* Endianess-optimized shifting of two u8_t to create one u16_t */ 00055 #if BYTE_ORDER == LITTLE_ENDIAN 00056 #define LWIP_MAKE_U16(a, b) ((a << 8) | b) 00057 #else 00058 #define LWIP_MAKE_U16(a, b) ((b << 8) | a) 00059 #endif 00060 00061 #ifndef LWIP_PLATFORM_BYTESWAP 00062 #define LWIP_PLATFORM_BYTESWAP 0 00063 #endif 00064 00065 #ifndef LWIP_PREFIX_BYTEORDER_FUNCS 00066 /* workaround for naming collisions on some platforms */ 00067 00068 #ifdef htons 00069 #undef htons 00070 #endif /* htons */ 00071 #ifdef htonl 00072 #undef htonl 00073 #endif /* htonl */ 00074 #ifdef ntohs 00075 #undef ntohs 00076 #endif /* ntohs */ 00077 #ifdef ntohl 00078 #undef ntohl 00079 #endif /* ntohl */ 00080 00081 #define htons(x) lwip_htons(x) 00082 #define ntohs(x) lwip_ntohs(x) 00083 #define htonl(x) lwip_htonl(x) 00084 #define ntohl(x) lwip_ntohl(x) 00085 #endif /* LWIP_PREFIX_BYTEORDER_FUNCS */ 00086 00087 #if BYTE_ORDER == BIG_ENDIAN 00088 #define lwip_htons(x) (x) 00089 #define lwip_ntohs(x) (x) 00090 #define lwip_htonl(x) (x) 00091 #define lwip_ntohl(x) (x) 00092 #define PP_HTONS(x) (x) 00093 #define PP_NTOHS(x) (x) 00094 #define PP_HTONL(x) (x) 00095 #define PP_NTOHL(x) (x) 00096 #else /* BYTE_ORDER != BIG_ENDIAN */ 00097 #if LWIP_PLATFORM_BYTESWAP 00098 #define lwip_htons(x) LWIP_PLATFORM_HTONS(x) 00099 #define lwip_ntohs(x) LWIP_PLATFORM_HTONS(x) 00100 #define lwip_htonl(x) LWIP_PLATFORM_HTONL(x) 00101 #define lwip_ntohl(x) LWIP_PLATFORM_HTONL(x) 00102 #else /* LWIP_PLATFORM_BYTESWAP */ 00103 u16_t lwip_htons(u16_t x); 00104 u16_t lwip_ntohs(u16_t x); 00105 u32_t lwip_htonl(u32_t x); 00106 u32_t lwip_ntohl(u32_t x); 00107 #endif /* LWIP_PLATFORM_BYTESWAP */ 00108 00109 /* These macros should be calculated by the preprocessor and are used 00110 with compile-time constants only (so that there is no little-endian 00111 overhead at runtime). */ 00112 #define PP_HTONS(x) ((((x) & 0xff) << 8) | (((x) & 0xff00) >> 8)) 00113 #define PP_NTOHS(x) PP_HTONS(x) 00114 #define PP_HTONL(x) ((((x) & 0xff) << 24) | \ 00115 (((x) & 0xff00) << 8) | \ 00116 (((x) & 0xff0000UL) >> 8) | \ 00117 (((x) & 0xff000000UL) >> 24)) 00118 #define PP_NTOHL(x) PP_HTONL(x) 00119 00120 #endif /* BYTE_ORDER == BIG_ENDIAN */ 00121 00122 #ifdef __cplusplus 00123 } 00124 #endif 00125 00126 #endif /* __LWIP_DEF_H__ */ 00127
Generated on Tue Jul 12 2022 11:52:56 by
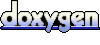