NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
api.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_API_H__ 00033 #define __LWIP_API_H__ 00034 00035 #include "lwip/opt.h" 00036 00037 #if LWIP_NETCONN /* don't build if not configured for use in lwipopts.h */ 00038 00039 #include <stddef.h> /* for size_t */ 00040 00041 #include "lwip/netbuf.h" 00042 #include "lwip/sys.h" 00043 #include "lwip/ip_addr.h" 00044 #include "lwip/err.h" 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /* Throughout this file, IP addresses and port numbers are expected to be in 00051 * the same byte order as in the corresponding pcb. 00052 */ 00053 00054 /* Flags for netconn_write (u8_t) */ 00055 #define NETCONN_NOFLAG 0x00 00056 #define NETCONN_NOCOPY 0x00 /* Only for source code compatibility */ 00057 #define NETCONN_COPY 0x01 00058 #define NETCONN_MORE 0x02 00059 #define NETCONN_DONTBLOCK 0x04 00060 00061 /* Flags for struct netconn.flags (u8_t) */ 00062 /** TCP: when data passed to netconn_write doesn't fit into the send buffer, 00063 this temporarily stores whether to wake up the original application task 00064 if data couldn't be sent in the first try. */ 00065 #define NETCONN_FLAG_WRITE_DELAYED 0x01 00066 /** Should this netconn avoid blocking? */ 00067 #define NETCONN_FLAG_NON_BLOCKING 0x02 00068 /** Was the last connect action a non-blocking one? */ 00069 #define NETCONN_FLAG_IN_NONBLOCKING_CONNECT 0x04 00070 /** If this is set, a TCP netconn must call netconn_recved() to update 00071 the TCP receive window (done automatically if not set). */ 00072 #define NETCONN_FLAG_NO_AUTO_RECVED 0x08 00073 /** If a nonblocking write has been rejected before, poll_tcp needs to 00074 check if the netconn is writable again */ 00075 #define NETCONN_FLAG_CHECK_WRITESPACE 0x10 00076 00077 00078 /* Helpers to process several netconn_types by the same code */ 00079 #define NETCONNTYPE_GROUP(t) (t&0xF0) 00080 #define NETCONNTYPE_DATAGRAM(t) (t&0xE0) 00081 00082 /** Protocol family and type of the netconn */ 00083 enum netconn_type { 00084 NETCONN_INVALID = 0, 00085 /* NETCONN_TCP Group */ 00086 NETCONN_TCP = 0x10, 00087 /* NETCONN_UDP Group */ 00088 NETCONN_UDP = 0x20, 00089 NETCONN_UDPLITE = 0x21, 00090 NETCONN_UDPNOCHKSUM= 0x22, 00091 /* NETCONN_RAW Group */ 00092 NETCONN_RAW = 0x40 00093 }; 00094 00095 /** Current state of the netconn. Non-TCP netconns are always 00096 * in state NETCONN_NONE! */ 00097 enum netconn_state { 00098 NETCONN_NONE, 00099 NETCONN_WRITE, 00100 NETCONN_LISTEN, 00101 NETCONN_CONNECT, 00102 NETCONN_CLOSE 00103 }; 00104 00105 /** Use to inform the callback function about changes */ 00106 enum netconn_evt { 00107 NETCONN_EVT_RCVPLUS, 00108 NETCONN_EVT_RCVMINUS, 00109 NETCONN_EVT_SENDPLUS, 00110 NETCONN_EVT_SENDMINUS, 00111 NETCONN_EVT_ERROR 00112 }; 00113 00114 #if LWIP_IGMP 00115 /** Used for netconn_join_leave_group() */ 00116 enum netconn_igmp { 00117 NETCONN_JOIN, 00118 NETCONN_LEAVE 00119 }; 00120 #endif /* LWIP_IGMP */ 00121 00122 /* forward-declare some structs to avoid to include their headers */ 00123 struct ip_pcb; 00124 struct tcp_pcb; 00125 struct udp_pcb; 00126 struct raw_pcb; 00127 struct netconn; 00128 struct api_msg_msg; 00129 00130 /** A callback prototype to inform about events for a netconn */ 00131 typedef void (* netconn_callback)(struct netconn *, enum netconn_evt, u16_t len); 00132 00133 /** A netconn descriptor */ 00134 struct netconn { 00135 /** type of the netconn (TCP, UDP or RAW) */ 00136 enum netconn_type type; 00137 /** current state of the netconn */ 00138 enum netconn_state state; 00139 /** the lwIP internal protocol control block */ 00140 union { 00141 struct ip_pcb *ip; 00142 struct tcp_pcb *tcp; 00143 struct udp_pcb *udp; 00144 struct raw_pcb *raw; 00145 } pcb; 00146 /** the last error this netconn had */ 00147 err_t last_err; 00148 /** sem that is used to synchroneously execute functions in the core context */ 00149 sys_sem_t op_completed; 00150 /** mbox where received packets are stored until they are fetched 00151 by the netconn application thread (can grow quite big) */ 00152 sys_mbox_t recvmbox; 00153 #if LWIP_TCP 00154 /** mbox where new connections are stored until processed 00155 by the application thread */ 00156 sys_mbox_t acceptmbox; 00157 #endif /* LWIP_TCP */ 00158 /** only used for socket layer */ 00159 #if LWIP_SOCKET 00160 int socket; 00161 #endif /* LWIP_SOCKET */ 00162 #if LWIP_SO_RCVTIMEO 00163 /** timeout to wait for new data to be received 00164 (or connections to arrive for listening netconns) */ 00165 int recv_timeout; 00166 #endif /* LWIP_SO_RCVTIMEO */ 00167 #if LWIP_SO_RCVBUF 00168 /** maximum amount of bytes queued in recvmbox 00169 not used for TCP: adjust TCP_WND instead! */ 00170 int recv_bufsize; 00171 #endif /* LWIP_SO_RCVBUF */ 00172 /** number of bytes currently in recvmbox to be received, 00173 tested against recv_bufsize to limit bytes on recvmbox 00174 for UDP and RAW 00175 @todo: should only be necessary with LWIP_SO_RCVBUF==1 */ 00176 s16_t recv_avail; 00177 /** flags holding more netconn-internal state, see NETCONN_FLAG_* defines */ 00178 u8_t flags; 00179 #if LWIP_TCP 00180 /** TCP: when data passed to netconn_write doesn't fit into the send buffer, 00181 this temporarily stores how much is already sent. */ 00182 size_t write_offset; 00183 /** TCP: when data passed to netconn_write doesn't fit into the send buffer, 00184 this temporarily stores the message. 00185 Also used during connect and close. */ 00186 struct api_msg_msg *current_msg; 00187 #endif /* LWIP_TCP */ 00188 /** A callback function that is informed about events for this netconn */ 00189 netconn_callback callback; 00190 }; 00191 00192 /** Register an Network connection event */ 00193 #define API_EVENT(c,e,l) if (c->callback) { \ 00194 (*c->callback)(c, e, l); \ 00195 } 00196 00197 /** Set conn->last_err to err but don't overwrite fatal errors */ 00198 #define NETCONN_SET_SAFE_ERR(conn, err) do { \ 00199 SYS_ARCH_DECL_PROTECT(lev); \ 00200 SYS_ARCH_PROTECT(lev); \ 00201 if (!ERR_IS_FATAL((conn)->last_err)) { \ 00202 (conn)->last_err = err; \ 00203 } \ 00204 SYS_ARCH_UNPROTECT(lev); \ 00205 } while(0); 00206 00207 /* Network connection functions: */ 00208 #define netconn_new(t) netconn_new_with_proto_and_callback(t, 0, NULL) 00209 #define netconn_new_with_callback(t, c) netconn_new_with_proto_and_callback(t, 0, c) 00210 struct 00211 netconn *netconn_new_with_proto_and_callback(enum netconn_type t, u8_t proto, 00212 netconn_callback callback); 00213 err_t netconn_delete(struct netconn *conn); 00214 /** Get the type of a netconn (as enum netconn_type). */ 00215 #define netconn_type(conn) (conn->type) 00216 00217 err_t netconn_getaddr(struct netconn *conn, ip_addr_t *addr, 00218 u16_t *port, u8_t local); 00219 #define netconn_peer(c,i,p) netconn_getaddr(c,i,p,0) 00220 #define netconn_addr(c,i,p) netconn_getaddr(c,i,p,1) 00221 00222 err_t netconn_bind(struct netconn *conn, ip_addr_t *addr, u16_t port); 00223 err_t netconn_connect(struct netconn *conn, ip_addr_t *addr, u16_t port); 00224 err_t netconn_disconnect (struct netconn *conn); 00225 err_t netconn_listen_with_backlog(struct netconn *conn, u8_t backlog); 00226 #define netconn_listen(conn) netconn_listen_with_backlog(conn, TCP_DEFAULT_LISTEN_BACKLOG) 00227 err_t netconn_accept(struct netconn *conn, struct netconn **new_conn); 00228 err_t netconn_recv(struct netconn *conn, struct netbuf **new_buf); 00229 err_t netconn_recv_tcp_pbuf(struct netconn *conn, struct pbuf **new_buf); 00230 void netconn_recved(struct netconn *conn, u32_t length); 00231 err_t netconn_sendto(struct netconn *conn, struct netbuf *buf, 00232 ip_addr_t *addr, u16_t port); 00233 err_t netconn_send(struct netconn *conn, struct netbuf *buf); 00234 err_t netconn_write(struct netconn *conn, const void *dataptr, size_t size, 00235 u8_t apiflags); 00236 err_t netconn_close(struct netconn *conn); 00237 err_t netconn_shutdown(struct netconn *conn, u8_t shut_rx, u8_t shut_tx); 00238 00239 #if LWIP_IGMP 00240 err_t netconn_join_leave_group(struct netconn *conn, ip_addr_t *multiaddr, 00241 ip_addr_t *netif_addr, enum netconn_igmp join_or_leave); 00242 #endif /* LWIP_IGMP */ 00243 #if LWIP_DNS 00244 err_t netconn_gethostbyname(const char *name, ip_addr_t *addr); 00245 #endif /* LWIP_DNS */ 00246 00247 #define netconn_err(conn) ((conn)->last_err) 00248 #define netconn_recv_bufsize(conn) ((conn)->recv_bufsize) 00249 00250 /** Set the blocking status of netconn calls (@todo: write/send is missing) */ 00251 #define netconn_set_nonblocking(conn, val) do { if(val) { \ 00252 (conn)->flags |= NETCONN_FLAG_NON_BLOCKING; \ 00253 } else { \ 00254 (conn)->flags &= ~ NETCONN_FLAG_NON_BLOCKING; }} while(0) 00255 /** Get the blocking status of netconn calls (@todo: write/send is missing) */ 00256 #define netconn_is_nonblocking(conn) (((conn)->flags & NETCONN_FLAG_NON_BLOCKING) != 0) 00257 00258 /** TCP: Set the no-auto-recved status of netconn calls (see NETCONN_FLAG_NO_AUTO_RECVED) */ 00259 #define netconn_set_noautorecved(conn, val) do { if(val) { \ 00260 (conn)->flags |= NETCONN_FLAG_NO_AUTO_RECVED; \ 00261 } else { \ 00262 (conn)->flags &= ~ NETCONN_FLAG_NO_AUTO_RECVED; }} while(0) 00263 /** TCP: Get the no-auto-recved status of netconn calls (see NETCONN_FLAG_NO_AUTO_RECVED) */ 00264 #define netconn_get_noautorecved(conn) (((conn)->flags & NETCONN_FLAG_NO_AUTO_RECVED) != 0) 00265 00266 #if LWIP_SO_RCVTIMEO 00267 /** Set the receive timeout in milliseconds */ 00268 #define netconn_set_recvtimeout(conn, timeout) ((conn)->recv_timeout = (timeout)) 00269 /** Get the receive timeout in milliseconds */ 00270 #define netconn_get_recvtimeout(conn) ((conn)->recv_timeout) 00271 #endif /* LWIP_SO_RCVTIMEO */ 00272 #if LWIP_SO_RCVBUF 00273 /** Set the receive buffer in bytes */ 00274 #define netconn_set_recvbufsize(conn, recvbufsize) ((conn)->recv_bufsize = (recvbufsize)) 00275 /** Get the receive buffer in bytes */ 00276 #define netconn_get_recvbufsize(conn) ((conn)->recv_bufsize) 00277 #endif /* LWIP_SO_RCVBUF*/ 00278 00279 #ifdef __cplusplus 00280 } 00281 #endif 00282 00283 #endif /* LWIP_NETCONN */ 00284 00285 #endif /* __LWIP_API_H__ */
Generated on Tue Jul 12 2022 11:52:56 by
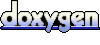