NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
UsbSerial.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef USB_SERIAL_H 00025 #define USB_SERIAL_H 00026 00027 //DG 2010 00028 //Essentially a clone of Serial if 00029 #include "Stream.h" 00030 #include "mbed.h" 00031 00032 #include "drv/usb/UsbDevice.h" 00033 #include "drv/usb/UsbEndpoint.h" 00034 00035 namespace mbed { 00036 00037 class UsbSerial : public Stream { 00038 00039 public: 00040 00041 UsbSerial(UsbDevice* pDevice, int epIn, int epOut, const char* name = NULL); 00042 virtual ~UsbSerial(); 00043 //Apart from the ctor/dtor, exactly the same protos as Serial 00044 00045 void baud(int baudrate); 00046 00047 enum Parity { 00048 None = 0, 00049 Odd = 1, 00050 Even = 2, 00051 Forced1 = 3, 00052 Forced0 = 4 00053 }; 00054 00055 enum IrqType { 00056 RxIrq = 0 00057 , TxIrq 00058 }; 00059 00060 void format(int bits, int parity, int stop); 00061 00062 class CDummy; 00063 template <class T> 00064 void attach(T* pCbItem, void (T::*pCbMeth)(), IrqType type = RxIrq) 00065 { 00066 if(type == RxIrq) 00067 { 00068 m_pInCbItem = (CDummy*) pCbItem; 00069 m_pInCbMeth = (void (CDummy::*)()) pCbMeth; 00070 } 00071 else 00072 { 00073 m_pOutCbItem = (CDummy*) pCbItem; 00074 m_pOutCbMeth = (void (CDummy::*)()) pCbMeth; 00075 } 00076 } 00077 00078 #if 0 // Inhereted from Stream, for documentation only 00079 00080 /* Function: putc 00081 * Write a character 00082 * 00083 * Variables: 00084 * c - The character to write to the serial port 00085 */ 00086 int putc(int c); 00087 00088 /* Function: getc 00089 * Read a character 00090 * 00091 * Variables: 00092 * returns - The character read from the serial port 00093 */ 00094 int getc(); 00095 00096 /* Function: printf 00097 * Write a formated string 00098 * 00099 * Variables: 00100 * format - A printf-style format string, followed by the 00101 * variables to use in formating the string. 00102 */ 00103 int printf(const char* format, ...); 00104 00105 /* Function: scanf 00106 * Read a formated string 00107 * 00108 * Variables: 00109 * format - A scanf-style format string, 00110 * followed by the pointers to variables to store the results. 00111 */ 00112 int scanf(const char* format, ...); 00113 00114 #endif 00115 00116 /* Function: readable 00117 * Determine if there is a character available to read 00118 * 00119 * Variables: 00120 * returns - 1 if there is a character available to read, else 0 00121 */ 00122 int readable(); 00123 00124 /* Function: writeable 00125 * Determine if there is space available to write a character 00126 * 00127 * Variables: 00128 * returns - 1 if there is space to write a character, else 0 00129 */ 00130 int writeable(); 00131 00132 #ifdef MBED_RPC 00133 virtual const struct rpc_method *get_rpc_methods(); 00134 static struct rpc_class *get_rpc_class(); 00135 #endif 00136 00137 protected: 00138 00139 virtual int _getc(); 00140 virtual int _putc(int c); 00141 00142 void onReadable(); 00143 void onWriteable(); 00144 00145 void onEpInTransfer(); 00146 void onEpOutTransfer(); 00147 00148 private: 00149 00150 UsbEndpoint m_epIn; 00151 UsbEndpoint m_epOut; 00152 00153 CDummy* m_pInCbItem; 00154 void (CDummy::*m_pInCbMeth)(); 00155 00156 CDummy* m_pOutCbItem; 00157 void (CDummy::*m_pOutCbMeth)(); 00158 00159 void startTx(); 00160 void startRx(); 00161 00162 Timeout m_txTimeout; 00163 volatile bool m_timeout; 00164 00165 volatile char* m_inBufEven; 00166 volatile char* m_inBufOdd; 00167 volatile char* m_inBufUsr; 00168 volatile char* m_inBufTrmt; 00169 00170 volatile char* m_outBufEven; 00171 volatile char* m_outBufOdd; 00172 volatile char* m_outBufUsr; 00173 volatile char* m_outBufTrmt; 00174 00175 volatile int m_inBufLen; 00176 volatile int m_outBufLen; 00177 00178 volatile char* m_pInBufPos; 00179 volatile char* m_pOutBufPos; 00180 00181 00182 00183 }; 00184 00185 } 00186 00187 00188 00189 #endif
Generated on Tue Jul 12 2022 11:52:59 by
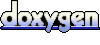