NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
UsbDevice.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "UsbDevice.h" 00025 00026 #include "netCfg.h" 00027 #if NET_USB 00028 00029 //#define __DEBUG 00030 #include "dbg/dbg.h" 00031 00032 UsbDevice::UsbDevice( UsbHostMgr* pMgr, int hub, int port, int addr ) : m_pControlEp(NULL), /*m_controlEp( this, 0x00, false, USB_CONTROL, 8 ),*/ 00033 m_pMgr(pMgr), m_connected(false), m_enumerated(false), m_hub(hub), m_port(port), m_addr(addr), m_refs(0), 00034 m_vid(0), m_pid(0) 00035 { 00036 00037 } 00038 00039 UsbDevice::~UsbDevice() 00040 { 00041 if(m_pControlEp) 00042 delete m_pControlEp; 00043 } 00044 00045 UsbErr UsbDevice::enumerate() 00046 { 00047 // USB_INT32S rc; 00048 00049 UsbErr rc; 00050 00051 DBG("Starting enumeration (m_pMgr = %p)\n", m_pMgr); 00052 00053 #if 1 00054 m_pMgr->resetPort(m_hub, m_port); 00055 #else 00056 wait_ms(100); /* USB 2.0 spec says atleast 50ms delay beore port reset */ 00057 LPC_USB->HcRhPortStatus1 = OR_RH_PORT_PRS; // Initiate port reset 00058 while (LPC_USB->HcRhPortStatus1 & OR_RH_PORT_PRS) 00059 __WFI(); // Wait for port reset to complete... 00060 LPC_USB->HcRhPortStatus1 = OR_RH_PORT_PRSC; // ...and clear port reset signal 00061 wait_ms(200); /* Wait for 100 MS after port reset */ 00062 #endif 00063 00064 DBG("Port reset\n"); 00065 00066 wait_ms(200); 00067 00068 m_pControlEp = new UsbEndpoint( this, 0x00, false, USB_CONTROL, 8, 0 ); 00069 00070 //EDCtrl->Control = 8 << 16;/* Put max pkt size = 8 */ 00071 /* Read first 8 bytes of device desc */ 00072 rc = controlReceive(USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, GET_DESCRIPTOR, (USB_DESCRIPTOR_TYPE_DEVICE << 8)|(0), 0, m_controlDataBuf, 8); 00073 if (rc) 00074 { 00075 DBG("RC=%d",rc); 00076 return (rc); 00077 } 00078 00079 DBG("Got descriptor, max ep size is %d\n", m_controlDataBuf[7]); 00080 00081 m_pControlEp->updateSize(m_controlDataBuf[7]); /* Get max pkt size of endpoint 0 */ 00082 rc = controlSend(USB_HOST_TO_DEVICE | USB_RECIPIENT_DEVICE, SET_ADDRESS, m_addr, 0, NULL, 0); /* Set the device address to m_addr */ 00083 if (rc) 00084 { 00085 // PRINT_Err(rc); 00086 return (rc); 00087 } 00088 wait_ms(2); 00089 //EDCtrl->Control = (EDCtrl->Control) | 1; /* Modify control pipe with address 1 */ 00090 00091 //Update address 00092 m_pControlEp->updateAddr(m_addr); 00093 DBG("Ep addr is now %d", m_addr); 00094 /**/ 00095 00096 //rc = HOST_GET_DESCRIPTOR(USB_DESCRIPTOR_TYPE_DEVICE, 0, TDBuffer, 17); //Read full device descriptor 00097 rc = controlReceive(USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, GET_DESCRIPTOR, (USB_DESCRIPTOR_TYPE_DEVICE << 8)|(0), 0, m_controlDataBuf, 17); 00098 if (rc) 00099 { 00100 //PRINT_Err(rc); 00101 return (rc); 00102 } 00103 /* 00104 rc = SerialCheckVidPid(); 00105 if (rc != OK) { 00106 PRINT_Err(rc); 00107 return (rc); 00108 } 00109 */ 00110 /**/ 00111 00112 m_vid = *((uint16_t*)&m_controlDataBuf[8]); 00113 m_pid = *((uint16_t*)&m_controlDataBuf[10]); 00114 00115 DBG("VID: %02x, PID: %02x\n", m_vid, m_pid); 00116 /* Get the configuration descriptor */ 00117 //rc = HOST_GET_DESCRIPTOR(USB_DESCRIPTOR_TYPE_CONFIGURATION, 0, TDBuffer, 9); 00118 rc = controlReceive(USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, GET_DESCRIPTOR, (USB_DESCRIPTOR_TYPE_CONFIGURATION << 8)|(0), 0, m_controlDataBuf, 9); 00119 if (rc) 00120 { 00121 //PRINT_Err(rc); 00122 return (rc); 00123 } 00124 /* Get the first configuration data */ 00125 //rc = HOST_GET_DESCRIPTOR(USB_DESCRIPTOR_TYPE_CONFIGURATION, 0, TDBuffer, *((uint16_t*)&TDBuffer[2])); 00126 rc = controlReceive(USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, GET_DESCRIPTOR, (USB_DESCRIPTOR_TYPE_CONFIGURATION << 8)|(0), 0, m_controlDataBuf, *((uint16_t*)&m_controlDataBuf[2])); 00127 if (rc) 00128 { 00129 //PRINT_Err(rc); 00130 return (rc); 00131 } 00132 00133 DBG("Desc len is %d\n", *((uint16_t*)&m_controlDataBuf[2])); 00134 00135 DBG("Set configuration\n"); 00136 00137 //rc = USBH_SET_CONFIGURATION(1);/* Select device configuration 1 */ 00138 rc = controlSend(USB_HOST_TO_DEVICE | USB_RECIPIENT_DEVICE, SET_CONFIGURATION, 1, 0, NULL, 0); 00139 if (rc) 00140 { 00141 // PRINT_Err(rc); 00142 return rc; 00143 } 00144 wait_ms(100);/* Some devices may require this delay */ 00145 00146 m_enumerated = true; 00147 return USBERR_OK; 00148 } 00149 00150 bool UsbDevice::connected() 00151 { 00152 return m_connected; 00153 } 00154 00155 bool UsbDevice::enumerated() 00156 { 00157 return m_enumerated; 00158 } 00159 00160 int UsbDevice::getPid() 00161 { 00162 return m_pid; 00163 } 00164 00165 int UsbDevice::getVid() 00166 { 00167 return m_vid; 00168 } 00169 00170 UsbErr UsbDevice::getConfigurationDescriptor(int config, uint8_t** pBuf) 00171 { 00172 //For now olny one config 00173 *pBuf = m_controlDataBuf; 00174 return USBERR_OK; 00175 } 00176 00177 UsbErr UsbDevice::getInterfaceDescriptor(int config, int item, uint8_t** pBuf) 00178 { 00179 byte* desc_ptr = m_controlDataBuf; 00180 00181 /* if (desc_ptr[1] != USB_DESCRIPTOR_TYPE_CONFIGURATION) 00182 { 00183 return USBERR_BADCONFIG; 00184 }*/ 00185 00186 if(item>=m_controlDataBuf[4])//Interfaces count 00187 return USBERR_NOTFOUND; 00188 00189 desc_ptr += desc_ptr[0]; 00190 00191 *pBuf = NULL; 00192 00193 while (desc_ptr < m_controlDataBuf + *((uint16_t*)&m_controlDataBuf[2])) 00194 { 00195 00196 switch (desc_ptr[1]) { 00197 case USB_DESCRIPTOR_TYPE_INTERFACE: 00198 if(desc_ptr[2] == item) 00199 { 00200 *pBuf = desc_ptr; 00201 return USBERR_OK; 00202 } 00203 desc_ptr += desc_ptr[0]; // Move to next descriptor start 00204 break; 00205 } 00206 00207 } 00208 00209 if(*pBuf == NULL) 00210 return USBERR_NOTFOUND; 00211 00212 return USBERR_OK; 00213 } 00214 00215 00216 UsbErr UsbDevice::setConfiguration(int config) 00217 { 00218 return USBERR_OK; 00219 } 00220 00221 UsbErr UsbDevice::controlSend(byte requestType, byte request, word value, word index, const byte* buf, int len) 00222 { 00223 UsbErr rc; 00224 fillControlBuf(requestType, request, value, index, len); 00225 m_pControlEp->setNextToken(TD_SETUP); 00226 rc = m_pControlEp->transfer(m_controlBuf, 8); 00227 while(m_pControlEp->status() == USBERR_PROCESSING); 00228 rc = (UsbErr) MIN(0, m_pControlEp->status()); 00229 if(rc) 00230 return rc; 00231 if(len) 00232 { 00233 m_pControlEp->setNextToken(TD_OUT); 00234 rc = m_pControlEp->transfer((byte*)buf, len); 00235 while(m_pControlEp->status() == USBERR_PROCESSING); 00236 rc = (UsbErr) MIN(0, m_pControlEp->status()); 00237 if(rc) 00238 return rc; 00239 } 00240 m_pControlEp->setNextToken(TD_IN); 00241 rc = m_pControlEp->transfer(NULL, 0); 00242 while(m_pControlEp->status() == USBERR_PROCESSING); 00243 rc = (UsbErr) MIN(0, m_pControlEp->status()); 00244 if(rc) 00245 return rc; 00246 return USBERR_OK; 00247 } 00248 00249 UsbErr UsbDevice::controlReceive(byte requestType, byte request, word value, word index, const byte* buf, int len) 00250 { 00251 UsbErr rc; 00252 fillControlBuf(requestType, request, value, index, len); 00253 m_pControlEp->setNextToken(TD_SETUP); 00254 rc = m_pControlEp->transfer(m_controlBuf, 8); 00255 while(m_pControlEp->status() == USBERR_PROCESSING); 00256 rc = (UsbErr) MIN(0, m_pControlEp->status()); 00257 if(rc) 00258 return rc; 00259 if(len) 00260 { 00261 m_pControlEp->setNextToken(TD_IN); 00262 rc = m_pControlEp->transfer( (byte*) buf, len); 00263 while(m_pControlEp->status() == USBERR_PROCESSING); 00264 rc = (UsbErr) MIN(0, m_pControlEp->status()); 00265 if(rc) 00266 return rc; 00267 } 00268 m_pControlEp->setNextToken(TD_OUT); 00269 rc = m_pControlEp->transfer(NULL, 0); 00270 while(m_pControlEp->status() == USBERR_PROCESSING); 00271 rc = (UsbErr) MIN(0, m_pControlEp->status()); 00272 if(rc) 00273 return rc; 00274 return USBERR_OK; 00275 } 00276 00277 void UsbDevice::fillControlBuf(byte requestType, byte request, word value, word index, int len) 00278 { 00279 #ifdef __BIG_ENDIAN 00280 #error "Must implement BE to LE conv here" 00281 #endif 00282 m_controlBuf[0] = requestType; 00283 m_controlBuf[1] = request; 00284 //We are in LE so it's fine 00285 *((word*)&m_controlBuf[2]) = value; 00286 *((word*)&m_controlBuf[4]) = index; 00287 *((word*)&m_controlBuf[6]) = (word) len; 00288 } 00289 00290 00291 #endif
Generated on Tue Jul 12 2022 11:52:58 by
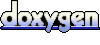