NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
UMTSStickNetIf.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /** \file 00025 UMTS Stick network interface header file 00026 */ 00027 00028 #ifndef UMTSSTICKNETIF_H 00029 #define UMTSSTICKNETIF_H 00030 00031 #include "mbed.h" 00032 00033 #include "core/net.h" 00034 #include "if/ppp/PPPNetIf.h" 00035 00036 #include "drv/umtsstick/UMTSStick.h" 00037 00038 ///UMTS Stick network interface 00039 /** 00040 This class provides connectivity to the stack using a 3G (or LTE etc...) stick 00041 Plug it to your USB host using two Pull-down resistors on the D+/D- lines 00042 */ 00043 class UMTSStickNetIf : public LwipNetIf, protected PPPNetIf 00044 { 00045 public: 00046 ///Instantiates the Interface and register it against the stack 00047 UMTSStickNetIf(); 00048 virtual ~UMTSStickNetIf(); 00049 00050 ///Tries to connect to the stick 00051 /** 00052 This method tries to obtain a virtual serial port interface from the stick 00053 It waits for a stick to be connected, switches it from CDFS to virtual serial port mode if needed, 00054 and obtains a virtual serial port from it 00055 @return : A negative error code on error or 0 on success 00056 */ 00057 UMTSStickErr setup(); //UMTSStickErr is from /drv/umtsstick/UMTSStick.h 00058 00059 ///Establishes a PPP connection 00060 /** 00061 This method opens an AT interface on the serial interface, initializes and configures the stick, 00062 then opens a PPP connection and authenticates with the parameters 00063 \param apn : APN of the interface, if NULL uses the SIM default value 00064 \param userId : user with which to authenticate during the PPP connection, if NULL does not authenticate 00065 \param password : associated password 00066 @return : A negative error code on error or 0 on success 00067 */ 00068 PPPErr connect(const char* apn = NULL, const char* userId = NULL, const char* password = NULL); //Connect using GPRS 00069 00070 ///Disconnects the PPP connection 00071 PPPErr disconnect(); 00072 00073 private: 00074 UMTSStick m_umtsStick; 00075 UsbSerial* m_pUsbSerial; 00076 00077 }; 00078 00079 #endif 00080
Generated on Tue Jul 12 2022 11:52:58 by
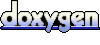