NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
PPPNetIf.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /** \file 00025 PPP Generic network interface header file 00026 */ 00027 00028 //This is a backend for PPP, using lwIP 00029 00030 #ifndef PPPNETIF_H 00031 #define PPPNETIF_H 00032 00033 #include "mbed.h" 00034 00035 //For now, only a GPRS Modem is supported, 00036 //but we could easily split this class into a base class + two derived classes 00037 // (PPP over GPRS + PPP over Serial Modem) 00038 #include "drv/gprs/GPRSModem.h" 00039 #include "if/lwip/LwipNetIf.h" 00040 00041 ///PPP connection error codes 00042 enum PPPErr 00043 { 00044 __PPP_MIN = -0xFFFF, 00045 PPP_MODEM, ///<AT error returned 00046 PPP_NETWORK, ///<Network is down 00047 PPP_PROTOCOL, ///<PPP Protocol error 00048 PPP_CLOSED, ///<Connection is closed 00049 PPP_OK = 0 ///<Success 00050 }; 00051 00052 enum PPPStatus 00053 { 00054 PPP_CONNECTING, 00055 PPP_CONNECTED, 00056 PPP_DISCONNECTED, 00057 }; 00058 00059 class PPPNetIf : public LwipNetIf 00060 { 00061 public: 00062 PPPNetIf(GPRSModem* pIf); 00063 virtual ~PPPNetIf(); 00064 00065 #if 0 00066 PPPErr open(Serial* pSerial); 00067 #endif 00068 00069 PPPErr GPRSConnect(const char* apn, const char* userId, const char* password); //Connect using GPRS 00070 PPPErr ATConnect(const char* number); //Connect using a "classic" voice modem or GSM 00071 00072 virtual void poll(); 00073 00074 PPPErr disconnect(); 00075 00076 #if 0 00077 PPPErr close(); 00078 #endif 00079 00080 protected: 00081 GPRSModem* m_pIf; 00082 00083 private: 00084 void pppCallback(int errCode, void *arg); 00085 00086 static void sPppCallback(void *ctx, int errCode, void *arg) //Callback from ppp.c 00087 { return ((PPPNetIf*)ctx)->pppCallback(errCode, arg); } 00088 00089 bool m_connected; 00090 /*bool m_open;*/ 00091 volatile PPPStatus m_status; 00092 volatile int m_fd; //PPP Session descriptor 00093 00094 //int m_id; 00095 00096 uint8_t* m_buf; 00097 00098 }; 00099 00100 #endif
Generated on Tue Jul 12 2022 11:52:58 by
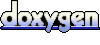