NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
HTTPRequestDispatcher.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "core/netservice.h" 00025 #include "HTTPRequestDispatcher.h" 00026 #include "HTTPRequestHandler.h" 00027 #include <string.h> 00028 00029 //#define __DEBUG 00030 #include "dbg/dbg.h" 00031 00032 HTTPRequestDispatcher::HTTPRequestDispatcher(HTTPServer* pSvr, TCPSocket* pTCPSocket) : NetService(), m_pSvr(pSvr), m_pTCPSocket(pTCPSocket), m_watchdog(), m_closed(false) 00033 { 00034 m_pTCPSocket->setOnEvent(this, &HTTPRequestDispatcher::onTCPSocketEvent); 00035 m_watchdog.attach_us<HTTPRequestDispatcher>(this, &HTTPRequestDispatcher::onTimeout, HTTP_REQUEST_TIMEOUT * 1000); 00036 } 00037 00038 HTTPRequestDispatcher::~HTTPRequestDispatcher() 00039 { 00040 close(); 00041 } 00042 00043 void HTTPRequestDispatcher::dispatchRequest() 00044 { 00045 string path; 00046 string meth; 00047 HTTP_METH methCode; 00048 00049 DBG("Dispatching req\r\n"); 00050 00051 if( !getRequest(&path, &meth ) ) 00052 { 00053 close(); 00054 return; //Invalid request 00055 } 00056 00057 if( !meth.compare("GET") ) 00058 { 00059 methCode = HTTP_GET; 00060 } 00061 else if( !meth.compare("POST") ) 00062 { 00063 methCode = HTTP_POST; 00064 } 00065 else if( !meth.compare("HEAD") ) 00066 { 00067 methCode = HTTP_HEAD; 00068 } 00069 else 00070 { 00071 close(); //Parse error 00072 return; 00073 } 00074 00075 DBG("Looking for a handler\r\n"); 00076 00077 map< string, HTTPRequestHandler*(*)(const char*, const char*, TCPSocket*) >::iterator it; 00078 // it = m_pSvr->m_lpHandlers.find(rootPath); //We are friends so we can do that 00079 // NEW CODE START: 00080 int root_len = 0; 00081 for (it = m_pSvr->m_lpHandlers.begin(); it != m_pSvr->m_lpHandlers.end(); it++) 00082 { 00083 DBG("Checking %s...\n", (*it).first.c_str()); 00084 root_len = (*it).first.length(); 00085 if ( root_len && 00086 !path.compare( 0, root_len, (*it).first ) && 00087 (path[root_len] == '/' || path[root_len] == '\0')) 00088 { 00089 DBG("Found (%s)\n", (*it).first.c_str()); 00090 // Found! 00091 break; // for 00092 } 00093 } 00094 // NEW CODE END 00095 if((it == m_pSvr->m_lpHandlers.end()) && !(m_pSvr->m_lpHandlers.empty())) 00096 { 00097 DBG("Using default handler\n"); 00098 it = m_pSvr->m_lpHandlers.end(); 00099 it--; //Get the last element 00100 if( ! (((*it).first.length() == 0) || !(*it).first.compare("/")) ) //This is not the default handler 00101 it = m_pSvr->m_lpHandlers.end(); 00102 root_len = 0; 00103 } 00104 if(it == m_pSvr->m_lpHandlers.end()) 00105 { 00106 DBG("No handler found\n"); 00107 close(); //No handler found 00108 return; 00109 } 00110 00111 DBG("Handler found.\r\n"); 00112 00113 //HTTPRequestHandler* pHdlr = (*it).second(rootPath.c_str(), subPath.c_str(), m_pTCPSocket); 00114 //NEW CODE 1 LINE: 00115 HTTPRequestHandler* pHdlr = (*it).second((*it).first.c_str(), path.c_str() + root_len, m_pTCPSocket); 00116 m_pTCPSocket = NULL; //We don't own it anymore 00117 00118 switch(methCode) 00119 { 00120 case HTTP_GET: 00121 pHdlr->doGet(); 00122 break; 00123 case HTTP_POST: 00124 pHdlr->doPost(); 00125 break; 00126 case HTTP_HEAD: 00127 pHdlr->doHead(); 00128 break; 00129 } 00130 00131 DBG("Req handled (or being handled)\r\n"); 00132 close(); 00133 } 00134 00135 void HTTPRequestDispatcher::close() //Close socket and destroy data 00136 { 00137 if(m_closed) 00138 return; 00139 m_closed = true; //Prevent recursive calling or calling on an object being destructed by someone else 00140 m_watchdog.detach(); 00141 if(m_pTCPSocket) //m_pTCPSocket Should only be destroyed if ownership not passed to an handler 00142 { 00143 m_pTCPSocket->resetOnEvent(); 00144 m_pTCPSocket->close(); 00145 delete m_pTCPSocket; //This fn might have been called by this socket (through an event), so DO NOT DESTROY IT HERE 00146 } 00147 NetService::close(); 00148 } 00149 00150 00151 void HTTPRequestDispatcher::onTimeout() //Connection has timed out 00152 { 00153 close(); 00154 } 00155 00156 bool HTTPRequestDispatcher::getRequest(string* path, string* meth) 00157 { 00158 char req[128]; 00159 char c_path[128]; 00160 char c_meth[128]; 00161 const int maxLen = 128; 00162 char* p = req; 00163 //Read Line 00164 int ret; 00165 int len = 0; 00166 for(int i = 0; i < maxLen - 1; i++) 00167 { 00168 ret = m_pTCPSocket->recv(p, 1); 00169 if(!ret) 00170 { 00171 break; 00172 } 00173 if( (len > 1) && *(p-1)=='\r' && *p=='\n' ) 00174 { 00175 p--; 00176 len-=2; 00177 break; 00178 } 00179 else if( *p=='\n' ) 00180 { 00181 len--; 00182 break; 00183 } 00184 p++; 00185 len++; 00186 } 00187 *p = 0; 00188 00189 DBG("Parsing request : %s\r\n", req); 00190 00191 ret = sscanf(req, "%s %s HTTP/%*d.%*d", c_meth, c_path); 00192 if(ret !=2) 00193 return false; 00194 00195 *meth = string(c_meth); 00196 // NEW CODE (old code removed): 00197 *path = string(c_path); 00198 return true; 00199 } 00200 00201 00202 00203 void HTTPRequestDispatcher::onTCPSocketEvent(TCPSocketEvent e) 00204 { 00205 00206 DBG("\r\nEvent %d\r\n", e); 00207 00208 if(m_closed) 00209 { 00210 DBG("\r\nWARN: Discarded\r\n"); 00211 return; 00212 } 00213 00214 switch(e) 00215 { 00216 case TCPSOCKET_READABLE: 00217 m_watchdog.detach(); 00218 m_pTCPSocket->resetOnEvent(); 00219 //Req arrived, dispatch : 00220 dispatchRequest(); 00221 break; 00222 case TCPSOCKET_CONTIMEOUT: 00223 case TCPSOCKET_CONRST: 00224 case TCPSOCKET_CONABRT: 00225 case TCPSOCKET_ERROR: 00226 case TCPSOCKET_DISCONNECTED: 00227 close(); 00228 break; 00229 } 00230 00231 }
Generated on Tue Jul 12 2022 11:52:57 by
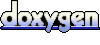