NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
HTTPFile.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /** \file 00025 HTTP File data source/sink header file 00026 */ 00027 00028 #ifndef HTTP_FILE_H 00029 #define HTTP_FILE_H 00030 00031 #include "../HTTPData.h" 00032 #include "mbed.h" 00033 00034 ///HTTP Client data container for files 00035 /** 00036 This class provides file access/storage for HTTP requests and responses' data payloads. 00037 00038 00039 */ 00040 class HTTPFile : public HTTPData //Read or Write data from a file 00041 { 00042 public: 00043 ///Instantiates data source/sink with file in param. 00044 /** 00045 Uses file at path @a path. 00046 It will be opened when some data has to be read/written from/to it and closed when this operation is complete or on destruction of the instance. 00047 Note that the file will be opened with mode "w" for writing and mode "r" for reading, so the file will be cleared between each request if you are using it for writing. 00048 00049 @note 00050 Note that to use this you must instantiate a proper file system (such as the LocalFileSystem or the SDFileSystem). 00051 */ 00052 HTTPFile(const char* path); 00053 virtual ~HTTPFile(); 00054 00055 ///Forces file closure 00056 virtual void clear(); 00057 00058 protected: 00059 virtual int read(char* buf, int len); 00060 virtual int write(const char* buf, int len); 00061 00062 virtual string getDataType(); //Internet media type for Content-Type header 00063 virtual void setDataType(const string& type); //Internet media type from Content-Type header 00064 00065 virtual bool getIsChunked(); //For Transfer-Encoding header 00066 virtual void setIsChunked(bool chunked); //From Transfer-Encoding header virtual 00067 00068 virtual int getDataLen(); //For Content-Length header 00069 virtual void setDataLen(int len); //From Content-Length header 00070 00071 private: 00072 bool openFile(const char* mode); //true on success, false otherwise 00073 void closeFile(); 00074 00075 FILE* m_fp; 00076 string m_path; 00077 int m_len; 00078 bool m_chunked; 00079 }; 00080 00081 #endif
Generated on Tue Jul 12 2022 11:52:57 by
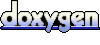