NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
GPRSModuleNetIf.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "netCfg.h" 00025 #if NET_GPRS_MODULE 00026 00027 #include "GPRSModuleNetIf.h" 00028 00029 #define __DEBUG 00030 #include "dbg/dbg.h" 00031 00032 GPRSModuleNetIf::GPRSModuleNetIf(PinName tx, PinName rx, int baud /*= 115200*/) : PPPNetIf(NULL), m_serial(tx, rx) 00033 { 00034 PPPNetIf::m_pIf = new GPRSModem(); 00035 m_serial.baud(baud); 00036 } 00037 00038 GPRSModuleNetIf::~GPRSModuleNetIf() 00039 { 00040 delete PPPNetIf::m_pIf; 00041 } 00042 00043 PPPErr GPRSModuleNetIf::connect(const char* apn /*= NULL*/, const char* userId /*= NULL*/, const char* password /*= NULL*/) //Connect using GPRS 00044 { 00045 00046 DBG("Powering on module.\n") 00047 if(!setOn()) //Could not power on module 00048 { 00049 DBG("Could not power on module.\n"); 00050 return PPP_MODEM; 00051 } 00052 00053 //wait(10); //Wait for module to init. 00054 00055 ATErr atErr; 00056 for(int i=0; i<3; i++) 00057 { 00058 atErr = m_pIf->open(&m_serial); //3 tries 00059 if(!atErr) 00060 break; 00061 DBG("Could not open AT If, trying again.\n"); 00062 wait(4); 00063 } 00064 00065 if(atErr) 00066 { 00067 setOff(); 00068 return PPP_MODEM; 00069 } 00070 00071 DBG("AT If opened.\n"); 00072 00073 PPPErr pppErr; 00074 for(int i=0; i<3; i++) 00075 { 00076 DBG("Trying to connect.\n"); 00077 pppErr = PPPNetIf::GPRSConnect(apn, userId, password); 00078 if(!pppErr) 00079 break; 00080 DBG("Could not connect.\n"); 00081 wait(4); 00082 } 00083 if(pppErr) 00084 { 00085 setOff(); 00086 return pppErr; 00087 } 00088 00089 DBG("Connected.\n"); 00090 00091 return PPP_OK; 00092 } 00093 00094 PPPErr GPRSModuleNetIf::disconnect() 00095 { 00096 DBG("Disconnecting...\n"); 00097 PPPErr pppErr = PPPNetIf::disconnect(); 00098 if(pppErr) 00099 return pppErr; 00100 00101 m_pIf->close(); 00102 00103 DBG("Powering off module.\n") 00104 setOff(); //Power off module 00105 00106 DBG("Off.\n") 00107 00108 return PPP_OK; 00109 } 00110 00111 00112 #endif
Generated on Tue Jul 12 2022 11:52:57 by
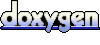