NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
ATIf.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef ATIF_H 00025 #define ATIF_H 00026 00027 #include "netCfg.h" 00028 00029 #include "mbed.h" 00030 #include "drv/serial/buf/SerialBuf.h" 00031 #include <list> 00032 using std::list; 00033 00034 //class Serial; //Pb w forward decl 00035 00036 enum ATErr 00037 { 00038 __AT_MIN = -0xFFFF, 00039 AT_CLOSED, 00040 AT_NOANSWER, 00041 AT_ERROR, 00042 AT_TIMEOUT, 00043 AT_BUSY, 00044 AT_PARSE, 00045 AT_INCOMPLETE, 00046 AT_OK = 0 00047 }; 00048 00049 class ATIf : public SerialBuf 00050 { 00051 public: 00052 00053 ATIf(); 00054 virtual ~ATIf(); 00055 00056 #if 0 00057 template<class T> 00058 //void attachSignal( const char* sigName, T* pItem, bool (T::*pMethod)(ATIf*, bool, bool*) ); //Attach Signal ("Unsollicited response code" in Telit_AT_Reference_Guide.pdf) to an handler fn 00059 //Linker bug : Must be defined here :( 00060 void attachSignal( const char* sigName, T* pItem, bool (T::*pMethod)(ATIf*, bool, bool*) ) //Attach Signal ("Unsollicited response code" in Telit_AT_Reference_Guide.pdf) to an handler fn 00061 { 00062 ATSigHandler sig(sigName, (ATSigHandler::CDummy*)pItem, (bool (ATSigHandler::CDummy::*)(ATIf*, bool, bool*))pMethod); 00063 m_signals.push_back(sig); 00064 } 00065 void detachSignal( const char* sigName ); 00066 #endif 00067 00068 ATErr open(Serial* pSerial); //Deactivate echo, etc 00069 #if NET_USB_SERIAL 00070 ATErr open(UsbSerial* pUsbSerial); //Deactivate echo, etc 00071 #endif 00072 ATErr close(); //Release port 00073 00074 int printf(const char* format, ... ); 00075 int scanf(const char* format, ... ); 00076 void setTimeout(int timeout); //used by scanf 00077 void setLineMode(bool lineMode); //Switch btw line & raw fns 00078 //void setSignals(bool signalsEnable); 00079 ATErr flushBuffer(); //Discard input buffer 00080 ATErr flushLine(int timeout); //Discard input buffer until CRLF is encountered 00081 00082 protected: 00083 //virtual bool onRead(); //Inherited from SerialBuf, return true if data is incomplete 00084 ATErr rawOpen(Serial* pSerial, int baudrate); //Simple open function for similar non-conforming protocols 00085 00086 public: 00087 /* ATErr command(const char* cmd, char* result, int resultLen, int timeout); */ //Kinda useless 00088 ATErr write(const char* cmd, bool lineMode = false); 00089 ATErr read(char* result, int resultMaxLen, int timeout, bool lineMode = false, int resultMinLen = 0); 00090 bool isOpen(); 00091 ATErr checkOK(); //Helper fn to quickly check that OK has been returned 00092 00093 private: 00094 int readLine(char* line, int maxLen, int timeout); //Read a single line from serial port 00095 int writeLine(const char* line); //Write a single line to serial port 00096 00097 int readRaw(char* str, int maxLen, int timeout = 0, int minLen = 0); //Read from serial port in buf 00098 int writeRaw(const char* str); //Write directly to serial port 00099 00100 volatile int m_readTimeout; 00101 volatile bool m_lineMode; 00102 //bool m_signalsEnable; 00103 bool m_isOpen; 00104 00105 char* m_tmpBuf; 00106 00107 #if 0 00108 class ATSigHandler 00109 { 00110 class CDummy; 00111 public: 00112 ATSigHandler(const char* name, CDummy* cbObj, bool (CDummy::*cbMeth)(ATIf* pIf, bool beg, bool* pMoreData)) : m_cbObj(cbObj), m_cbMeth(cbMeth), m_name(name) 00113 {} 00114 protected: 00115 CDummy* m_cbObj; 00116 bool (CDummy::*m_cbMeth)(ATIf* pIf, bool beg, bool* pMoreData); //*pMoreData set to true if needs to read next line, beg = true if beginning of new code 00117 const char* m_name; 00118 00119 friend class ATIf; 00120 }; 00121 00122 volatile ATSigHandler* m_pCurrentSignal; //Signal that asked more data 00123 00124 bool handleSignal(); //Returns true if signal has been handled 00125 list<ATSigHandler> m_signals; 00126 #endif 00127 00128 }; 00129 00130 #endif
Generated on Tue Jul 12 2022 11:52:56 by
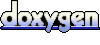