FTP client library for mbed-os
Embed:
(wiki syntax)
Show/hide line numbers
FTPClient.h
00001 /* FTP client library 00002 * Copyright (c) 2018 Renesas Electronics Corporation 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef FTP_CLIENT_H 00018 #define FTP_CLIENT_H 00019 #include "mbed.h" 00020 00021 /** FTPClient class. 00022 */ 00023 class FTPClient { 00024 public: 00025 /** Constructor 00026 * 00027 * @param net FTP server port 00028 * @param root User name 00029 */ 00030 FTPClient(NetworkInterface *net, const char* root); 00031 ~FTPClient(); 00032 00033 /** Opens address. 00034 * 00035 * @param ip_addr FTP server IP address 00036 * @param port FTP server port 00037 * @param user User name 00038 * @param pass Password 00039 * @return true = success, false = failure 00040 */ 00041 bool open(const char* ip_addr, int port, const char* user, const char* pass); 00042 00043 /** Exits from FTP. 00044 * 00045 * @return true = success, false = failure 00046 */ 00047 bool quit(); 00048 00049 /** Get file from the remote computer. 00050 * 00051 * @param file_name File name 00052 * @return true = success, false = failure 00053 */ 00054 bool get(const char* file_name); 00055 00056 /** Send one file. 00057 * 00058 * @param file_name File name 00059 * @return true = success, false = failure 00060 */ 00061 bool put(const char* file_name); 00062 00063 /** Deletes a file. 00064 * 00065 * @param file_name File name 00066 * @return true = success, false = failure 00067 */ 00068 bool del(const char* file_name); 00069 00070 /** Make directory. 00071 * 00072 * @param dir_name Directory name 00073 * @return true = success, false = failure 00074 */ 00075 bool mkdir(const char* dir_name); 00076 00077 /** Changes directory. 00078 * 00079 * @param dir_name Directory name 00080 * @return true = success, false = failure 00081 */ 00082 bool cd(const char* dir_name); 00083 00084 /** Lists files, if connected. 00085 * 00086 * @param list_buf Buffer to store results 00087 * @param buf_size Size of list_buf 00088 * @return true = success, false = failure 00089 */ 00090 bool dir(char* list_buf, int buf_size); 00091 00092 /** Lists files of the remotely connected computer. 00093 * 00094 * @param list_buf Buffer to store results 00095 * @param buf_size Size of list_buf 00096 * @return true = success, false = failure 00097 */ 00098 bool ls(char* list_buf, int buf_size); 00099 00100 private: 00101 NetworkInterface * p_network; 00102 TCPSocket FTPClientControlSock; 00103 TCPSocket FTPClientDataSock; 00104 bool _ctr_open; 00105 bool _login; 00106 char ftp_data_ip_addr[20]; 00107 char * p_ftp_buf; 00108 char _root[20]; 00109 00110 bool open_data_sock(); 00111 }; 00112 #endif
Generated on Thu Jul 14 2022 08:33:19 by
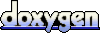