Sprint game for GR-PEACH.
Dependencies: GR-PEACH_video GraphicsFramework LCD_shield_config R_BSP
main.cpp
00001 #include<stdio.h> 00002 #include<stdlib.h> 00003 #include<time.h> 00004 #include "mbed.h" 00005 #include "DisplayBace.h" 00006 #include "RGA.h" 00007 #include "rtos.h" 00008 #include "BinaryImage_RZ_A1H.h" 00009 #include "LCD_shield_config_4_3inch.h" 00010 00011 #define GRAPHICS_FORMAT (DisplayBase::GRAPHICS_FORMAT_RGB565) 00012 #define WR_RD_WRSWA (DisplayBase::WR_RD_WRSWA_32_16BIT) 00013 00014 /* FRAME BUFFER Parameter */ 00015 #define FRAME_BUFFER_BYTE_PER_PIXEL (2) 00016 #define FRAME_BUFFER_STRIDE (((LCD_PIXEL_WIDTH * FRAME_BUFFER_BYTE_PER_PIXEL) + 31u) & ~31u) 00017 00018 #define GOAL_POINT (15000) 00019 #define NO_IMAGE (0xFFFFFFFF) 00020 #define LANGUAGE_NUM (2) 00021 00022 typedef struct { 00023 int now_pos; 00024 int tutorial_seq; 00025 int total_time; 00026 int record_time; 00027 int result_seq; 00028 int hito_ofs; 00029 int max_speed; 00030 int goal_seq; 00031 int hosi_seq; 00032 int speed; 00033 uint32_t hito_no; 00034 uint32_t comment_no; 00035 uint32_t ready_set_go; 00036 const graphics_image_t * p_hito; 00037 bool tutorial; 00038 bool swipe_on; 00039 bool goal_on; 00040 bool result_on; 00041 bool new_record; 00042 bool hosi; 00043 bool first_record; 00044 bool time_and_speed; 00045 } disp_func_t; 00046 00047 static DisplayBase Display; 00048 static Canvas2D_ContextClass canvas2d; 00049 static DigitalOut lcd_pwon(P7_15); 00050 static DigitalOut lcd_blon(P8_1); 00051 static PwmOut lcd_cntrst(P8_15); 00052 static Serial pc(USBTX, USBRX); 00053 static Semaphore sem_touch_int(0); 00054 static TouckKey_LCD_shield touch(P4_0, P2_13, I2C_SDA, I2C_SCL); 00055 static Timer stop_watch; 00056 static InterruptIn button(USER_BUTTON0); 00057 static disp_func_t disp_info; 00058 static bool touch_click = false; 00059 static bool touch_start = false; 00060 static bool race_start = false; 00061 static int total_move_x = 0; 00062 static int wk_wait = 0; 00063 static int pic_idx = 0; 00064 static int tukare = 0; 00065 static int table_no = 0; 00066 static int language = 0; /* 0:English */ 00067 00068 #if defined(__ICCARM__) 00069 #pragma data_alignment=32 00070 static uint8_t user_frame_buffer1[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00071 #pragma data_alignment=32 00072 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00073 #else 00074 static uint8_t user_frame_buffer1[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); /* 32 bytes aligned */ 00075 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); /* 32 bytes aligned */ 00076 #endif 00077 static frame_buffer_t frame_buffer_info; 00078 static volatile int32_t vsync_count = 0; 00079 00080 static const graphics_image_t * hito_tukare[] = {tukare1_File, tukare2_File}; 00081 static const graphics_image_t * hito_speed_1[] = {run1_File, run2_File, run3_File, run4_File, run5_File, run6_File, run7_File, run6_File, run5_File, run4_File, run3_File, run2_File}; 00082 static const graphics_image_t * hito_speed_2[] = {dash1_File, dash2_File, dash3_File, dash4_File, dash3_File, dash2_File}; 00083 static const graphics_image_t * hito_speed_3[] = {sdash1_File, sdash2_File, sdash3_File, sdash2_File}; 00084 static const graphics_image_t * comment_tbl[LANGUAGE_NUM][5] = { 00085 {comment1_en_File, comment2_en_File, comment3_en_File, comment4_en_File, comment5_en_File}, /* English */ 00086 {comment1_jp_File, comment2_jp_File, comment3_jp_File, comment4_jp_File, comment5_jp_File} /* Japanese */ 00087 }; 00088 static const graphics_image_t * ready_set_go_tbl[] = {go_File, start1_File, start2_File, start3_File}; 00089 static const graphics_image_t * num_tbl[] = {num_0_File, num_1_File, num_2_File, num_3_File, num_4_File, num_5_File, num_6_File, num_7_File, num_8_File, num_9_File}; 00090 00091 static void IntCallbackFunc_LoVsync(DisplayBase::int_type_t int_type) { 00092 /* Interrupt callback function for Vsync interruption */ 00093 if (vsync_count > 0) { 00094 vsync_count--; 00095 } 00096 } 00097 00098 static void Wait_Vsync(const int32_t wait_count) { 00099 /* Wait for the specified number of times Vsync occurs */ 00100 vsync_count = wait_count; 00101 while (vsync_count > 0) { 00102 /* Do nothing */ 00103 } 00104 } 00105 00106 static void Init_LCD_Display(void) { 00107 DisplayBase::graphics_error_t error; 00108 DisplayBase::lcd_config_t lcd_config; 00109 PinName lvds_pin[8] = { 00110 /* data pin */ 00111 P5_7, P5_6, P5_5, P5_4, P5_3, P5_2, P5_1, P5_0 00112 }; 00113 00114 lcd_pwon = 0; 00115 lcd_blon = 0; 00116 Thread::wait(100); 00117 lcd_pwon = 1; 00118 lcd_blon = 1; 00119 00120 Display.Graphics_Lvds_Port_Init(lvds_pin, 8); 00121 00122 /* Graphics initialization process */ 00123 lcd_config = LcdCfgTbl_LCD_shield; 00124 error = Display.Graphics_init(&lcd_config); 00125 if (error != DisplayBase::GRAPHICS_OK) { 00126 printf("Line %d, error %d\n", __LINE__, error); 00127 mbed_die(); 00128 } 00129 00130 #if(1) /* When needing LCD Vsync interrupt, please make it effective. */ 00131 /* Interrupt callback function setting (Vsync signal output from scaler 0) */ 00132 error = Display.Graphics_Irq_Handler_Set(DisplayBase::INT_TYPE_S0_LO_VSYNC, 0, IntCallbackFunc_LoVsync); 00133 if (error != DisplayBase::GRAPHICS_OK) { 00134 printf("Line %d, error %d\n", __LINE__, error); 00135 mbed_die(); 00136 } 00137 #endif 00138 } 00139 00140 static void Start_LCD_Display(uint8_t * p_buf) { 00141 DisplayBase::rect_t rect; 00142 00143 rect.vs = 0; 00144 rect.vw = LCD_PIXEL_HEIGHT; 00145 rect.hs = 0; 00146 rect.hw = LCD_PIXEL_WIDTH; 00147 Display.Graphics_Read_Setting( 00148 DisplayBase::GRAPHICS_LAYER_0, 00149 (void *)p_buf, 00150 FRAME_BUFFER_STRIDE, 00151 GRAPHICS_FORMAT, 00152 WR_RD_WRSWA, 00153 &rect 00154 ); 00155 Display.Graphics_Start(DisplayBase::GRAPHICS_LAYER_0); 00156 } 00157 00158 static void Update_LCD_Display(frame_buffer_t * frmbuf_info) { 00159 Display.Graphics_Read_Change(DisplayBase::GRAPHICS_LAYER_0, 00160 (void *)frmbuf_info->buffer_address[frmbuf_info->draw_buffer_index]); 00161 Wait_Vsync(1); 00162 } 00163 00164 static void Swap_FrameBuffer(frame_buffer_t * frmbuf_info) { 00165 if (frmbuf_info->draw_buffer_index == 1) { 00166 frmbuf_info->draw_buffer_index = 0; 00167 } else { 00168 frmbuf_info->draw_buffer_index = 1; 00169 } 00170 } 00171 00172 static void draw_time(int time_num, int pos_x, int pos_y, int disp_flg) { 00173 int wk_num; 00174 00175 wk_num = (time_num / 100) % 10; 00176 if ((wk_num != 0) || (disp_flg != false)) { 00177 canvas2d.drawImage(num_tbl[wk_num], pos_x + 17 * 0, pos_y); /* 400x42 */ 00178 R_OSPL_CLEAR_ERROR(); 00179 disp_flg = true; 00180 } 00181 wk_num = (time_num / 10) % 10; 00182 if ((wk_num != 0) || (disp_flg != false)) { 00183 canvas2d.drawImage(num_tbl[wk_num], pos_x + 17 * 1, pos_y); /* 400x42 */ 00184 R_OSPL_CLEAR_ERROR(); 00185 } 00186 wk_num = time_num % 10; 00187 canvas2d.drawImage(num_tbl[wk_num], pos_x + 17 * 2, pos_y); /* 400x42 */ 00188 R_OSPL_CLEAR_ERROR(); 00189 } 00190 00191 static void draw_total_time(int time_ms, int x, int y) { 00192 int wk_time_sec = time_ms / 1000; /* sec */ 00193 int wk_time_ms = time_ms % 1000; /* ms */ 00194 00195 if (wk_time_sec > 999) { 00196 wk_time_sec = 999; 00197 wk_time_ms = 999; 00198 } 00199 draw_time(wk_time_sec, x, y, false); 00200 draw_time(wk_time_ms, x + 61, y, true); 00201 } 00202 00203 static void touch_int_callback(void) { 00204 sem_touch_int.release(); 00205 } 00206 00207 void touch_task(void const *) { 00208 TouchKey::touch_pos_t touch_pos; 00209 int touch_num = 0; 00210 int touch_num_last = 0; 00211 int move_x = 0; 00212 uint32_t last_x = 0; 00213 00214 /* Callback setting */ 00215 touch.SetCallback(&touch_int_callback); 00216 00217 /* Reset touch IC */ 00218 touch.Reset(); 00219 00220 while (1) { 00221 /* Wait touch event */ 00222 sem_touch_int.wait(); 00223 00224 /* Get touch coordinates */ 00225 touch_num = touch.GetCoordinates(1, &touch_pos); 00226 00227 if (touch_num != 0) { 00228 if (touch_num_last == 0) { 00229 /* key On */ 00230 } else { 00231 /* Move */ 00232 if (touch_start) { 00233 move_x = (int)touch_pos.x - (int)last_x; 00234 if (move_x > 0) { 00235 total_move_x += move_x; 00236 } 00237 } 00238 } 00239 } else { 00240 /* key Off */ 00241 touch_click = true; 00242 } 00243 00244 touch_num_last = touch_num; 00245 last_x = touch_pos.x; 00246 } 00247 } 00248 00249 static void display_func(frame_buffer_t* frmbuf_info) { 00250 int wk_osf; 00251 int wk_pos; 00252 00253 Swap_FrameBuffer(frmbuf_info); 00254 00255 /* Clear */ 00256 canvas2d.clearRect(0, 0, frmbuf_info->width, frmbuf_info->height); 00257 00258 /* Distant background */ 00259 wk_osf = ((disp_info.now_pos / 8) % 1000) + LCD_PIXEL_WIDTH - 1000; 00260 canvas2d.globalAlpha = 0.6f; 00261 if (wk_osf > 0) { 00262 canvas2d.drawImage(haikei_File, -1000 + wk_osf, 40); /* 1000x173 */ 00263 R_OSPL_CLEAR_ERROR(); 00264 } 00265 canvas2d.drawImage(haikei_File, wk_osf, 40); 00266 R_OSPL_CLEAR_ERROR(); 00267 00268 /* Nearby background */ 00269 wk_osf = ((disp_info.now_pos / 2) % 500) + LCD_PIXEL_WIDTH - 500; 00270 canvas2d.globalAlpha = 1.0f; 00271 if (wk_osf > 0) { 00272 canvas2d.drawImage(haikei_temae_File, -500 + wk_osf, 91); /* 500x130 */ 00273 R_OSPL_CLEAR_ERROR(); 00274 } 00275 canvas2d.drawImage(haikei_temae_File, wk_osf, 91); /* 500x130 */ 00276 R_OSPL_CLEAR_ERROR(); 00277 00278 /* Progress */ 00279 canvas2d.globalAlpha = 1.0f; 00280 canvas2d.drawImage(logo_goal_File, 10, 5); /* 23x20 */ 00281 R_OSPL_CLEAR_ERROR(); 00282 canvas2d.drawImage(logo_start_File, LCD_PIXEL_WIDTH - 23 - 10, 5); /* 23x20 */ 00283 R_OSPL_CLEAR_ERROR(); 00284 00285 wk_pos = disp_info.now_pos; 00286 00287 if (wk_pos > GOAL_POINT) { 00288 wk_pos = GOAL_POINT; 00289 } 00290 canvas2d.drawImage(logo_genzai_File, 00291 (int)(((float_t)LCD_PIXEL_WIDTH - 75) * ((float_t)(GOAL_POINT - wk_pos) / GOAL_POINT) + 26), 5); 00292 R_OSPL_CLEAR_ERROR(); 00293 00294 /* Human */ 00295 if (disp_info.p_hito != NULL) { 00296 canvas2d.globalAlpha = 1.0f; 00297 canvas2d.drawImage(disp_info.p_hito, (LCD_PIXEL_WIDTH - 143) / 2 + disp_info.hito_ofs, LCD_PIXEL_HEIGHT - 210); 00298 R_OSPL_CLEAR_ERROR(); 00299 } 00300 00301 /* Serif */ 00302 canvas2d.globalAlpha = 1.0f; 00303 if (disp_info.tutorial) { 00304 canvas2d.drawImage(comment_tbl[language][0], 43, 50); 00305 R_OSPL_CLEAR_ERROR(); 00306 canvas2d.drawImage(comment_tbl[language][1], 275, 50); 00307 R_OSPL_CLEAR_ERROR(); 00308 } 00309 if (disp_info.comment_no != NO_IMAGE) { 00310 canvas2d.drawImage(comment_tbl[language][disp_info.comment_no], 265, 50); 00311 R_OSPL_CLEAR_ERROR(); 00312 } 00313 00314 /* Swipe */ 00315 if (disp_info.swipe_on) { 00316 canvas2d.globalAlpha = 0.9f; 00317 canvas2d.drawImage(swipe_File, disp_info.tutorial_seq * 6, (LCD_PIXEL_HEIGHT / 2) - 10); 00318 disp_info.tutorial_seq++; 00319 if ((disp_info.tutorial_seq * 6) >= LCD_PIXEL_WIDTH) { 00320 disp_info.tutorial_seq = 0; 00321 } 00322 } 00323 00324 /* Ready Set Go! */ 00325 if (disp_info.ready_set_go != NO_IMAGE) { 00326 canvas2d.globalAlpha = 0.82f; 00327 canvas2d.drawImage(ready_set_go_tbl[disp_info.ready_set_go], (LCD_PIXEL_WIDTH - 100) / 2, 00328 (LCD_PIXEL_HEIGHT - 100) / 2); 00329 R_OSPL_CLEAR_ERROR(); 00330 } 00331 00332 /* Goooal! */ 00333 if (disp_info.goal_on) { 00334 canvas2d.globalAlpha = (0.04f * disp_info.goal_seq); 00335 canvas2d.drawImage(goooal_File, 00336 (LCD_PIXEL_WIDTH - 260 * (2.0 - (float_t)disp_info.goal_seq / 25)) / 2, 00337 (LCD_PIXEL_HEIGHT - 53 * (2.0 - (float_t)disp_info.goal_seq / 25)) / 2, 00338 260 * (2.0 - (float_t)disp_info.goal_seq / 25), 00339 53 * (2.0 - (float_t)disp_info.goal_seq / 25)); /* 260x53 */ 00340 R_OSPL_CLEAR_ERROR(); 00341 } 00342 00343 /* Result */ 00344 if (disp_info.result_on) { 00345 /* record */ 00346 canvas2d.globalAlpha = (0.025f * disp_info.result_seq); 00347 canvas2d.drawImage(result_File, (LCD_PIXEL_WIDTH - 400) / 2, 00348 LCD_PIXEL_HEIGHT / 2 + 32 - disp_info.result_seq); /* 400x68 */ 00349 R_OSPL_CLEAR_ERROR(); 00350 draw_total_time(disp_info.total_time, (LCD_PIXEL_WIDTH / 2 - 200 + 185), 00351 (LCD_PIXEL_HEIGHT / 2 + 9) + 32 - disp_info.result_seq); 00352 draw_time(disp_info.max_speed, (LCD_PIXEL_WIDTH / 2 - 200 + 185 + 34), 00353 (LCD_PIXEL_HEIGHT / 2 + 20 + 9) + 32 - disp_info.result_seq, false); 00354 } 00355 00356 /* 1st record */ 00357 if (disp_info.first_record) { 00358 canvas2d.drawImage(record_1st_File, 145, 201); 00359 R_OSPL_CLEAR_ERROR(); 00360 draw_total_time(disp_info.record_time, 145 + 20, 201); 00361 canvas2d.drawImage(dot_File, 145 + 20 + 52, 201 + 11); /* . */ 00362 R_OSPL_CLEAR_ERROR(); 00363 canvas2d.drawImage(sec_File, 145 + 20 + 115, 201); /* sec */ 00364 R_OSPL_CLEAR_ERROR(); 00365 } 00366 00367 /* New Record */ 00368 if (disp_info.new_record) { 00369 canvas2d.globalAlpha = 1.0f; 00370 canvas2d.drawImage(new_record_File, (LCD_PIXEL_WIDTH - 194) / 2, 20); 00371 R_OSPL_CLEAR_ERROR(); 00372 } 00373 00374 /* Star */ 00375 if (disp_info.hosi) { 00376 int wk_seq; 00377 00378 if (disp_info.hosi_seq < 25) { 00379 wk_seq = disp_info.hosi_seq; 00380 canvas2d.globalAlpha = 1.0f - (0.04f * wk_seq); 00381 canvas2d.drawImage(hosi_File, 300, 60); 00382 } else if (disp_info.hosi_seq < 50) { 00383 wk_seq = disp_info.hosi_seq - 25; 00384 canvas2d.globalAlpha = 1.0f - (0.04f * wk_seq); 00385 canvas2d.drawImage(hosi_File, 70, 100); 00386 } else { 00387 wk_seq = disp_info.hosi_seq - 50; 00388 canvas2d.globalAlpha = 1.0f - (0.04f * wk_seq); 00389 canvas2d.drawImage(hosi_File, 270, 170); 00390 } 00391 R_OSPL_CLEAR_ERROR(); 00392 disp_info.hosi_seq++; 00393 if (disp_info.hosi_seq >= 75) { 00394 disp_info.hosi_seq = 0; 00395 } 00396 } 00397 00398 /* Speed and Time */ 00399 if (disp_info.time_and_speed) { 00400 int wk_ofs; 00401 00402 canvas2d.globalAlpha = 1.0f; 00403 00404 /* Speed */ 00405 if (disp_info.speed >= 100) { 00406 wk_ofs = 10; 00407 } else if (disp_info.speed >= 10) { 00408 wk_ofs = 10 - 17; 00409 } else { 00410 wk_ofs = 10 - 17 - 17; 00411 } 00412 draw_time(disp_info.speed, wk_ofs, LCD_PIXEL_HEIGHT - 19 - 5, false); 00413 /* Time */ 00414 draw_total_time(stop_watch.read_ms(), LCD_PIXEL_WIDTH - 112 - 5, LCD_PIXEL_HEIGHT - 19 - 5); 00415 canvas2d.drawImage(dot_File, LCD_PIXEL_WIDTH - 112 - 5 + 52, LCD_PIXEL_HEIGHT - 19 - 5 + 11); /* . */ 00416 R_OSPL_CLEAR_ERROR(); 00417 } 00418 00419 /* Complete drawing */ 00420 R_GRAPHICS_Finish(canvas2d.c_LanguageContext); 00421 Update_LCD_Display(frmbuf_info); 00422 } 00423 00424 static void set_hito_pic(void) { 00425 int new_table_no = 0; 00426 int bonus; 00427 float_t bonu_rate; 00428 00429 disp_info.speed = total_move_x / 50; 00430 bonu_rate = ((float_t)disp_info.speed / 100.00); 00431 if (bonu_rate > 0.70) { 00432 bonu_rate = 0.70; 00433 } 00434 bonus = (int)((float_t)disp_info.speed * bonu_rate); 00435 00436 if (disp_info.speed > disp_info.max_speed) { 00437 disp_info.max_speed = disp_info.speed; 00438 } 00439 00440 if (disp_info.speed >= 50) { 00441 disp_info.comment_no = NO_IMAGE; 00442 new_table_no = 3; 00443 } else if (disp_info.speed >= 30) { 00444 tukare = 8; 00445 if ((race_start != false) && (disp_info.comment_no == 3)) { 00446 disp_info.comment_no = 4; 00447 } 00448 new_table_no = 2; 00449 } else if (disp_info.speed >= 1) { 00450 if ((race_start != false) && (disp_info.comment_no == 2)) { 00451 disp_info.comment_no = 3; 00452 } 00453 new_table_no = 1; 00454 } else { 00455 if (race_start != false) { 00456 disp_info.comment_no = 2; 00457 } 00458 new_table_no = 0; 00459 } 00460 00461 if (new_table_no != table_no) { 00462 table_no = new_table_no; 00463 pic_idx = 0; 00464 } 00465 00466 if (wk_wait == 0) { 00467 switch (table_no) { 00468 case 0: 00469 if (tukare > 0) { 00470 tukare--; 00471 disp_info.p_hito = hito_tukare[pic_idx++]; 00472 if (pic_idx >= (sizeof(hito_tukare) / sizeof(graphics_image_t *))) { 00473 pic_idx = 0; 00474 } 00475 wk_wait = 20; 00476 } else { 00477 disp_info.p_hito = start_File; 00478 pic_idx = 0; 00479 wk_wait = 3; 00480 } 00481 break; 00482 case 1: 00483 disp_info.p_hito = hito_speed_1[pic_idx++]; 00484 if (pic_idx >= (sizeof(hito_speed_1) / sizeof(graphics_image_t *))) { 00485 pic_idx = 0; 00486 } 00487 if (disp_info.speed <= 1) { 00488 wk_wait = 6; 00489 } else if (disp_info.speed <= 3) { 00490 wk_wait = 5; 00491 } else if (disp_info.speed <= 6) { 00492 wk_wait = 4; 00493 } else if (disp_info.speed <= 12) { 00494 wk_wait = 3; 00495 } else if (disp_info.speed <= 20) { 00496 wk_wait = 2; 00497 } else { 00498 wk_wait = 1; 00499 } 00500 break; 00501 case 2: 00502 disp_info.p_hito = hito_speed_2[pic_idx++]; 00503 if (pic_idx >= (sizeof(hito_speed_2) / sizeof(graphics_image_t *))) { 00504 pic_idx = 0; 00505 } 00506 if (disp_info.speed <= 40) { 00507 wk_wait = 1; 00508 } else { 00509 wk_wait = 0; 00510 } 00511 break; 00512 case 3: 00513 disp_info.p_hito = hito_speed_3[pic_idx++]; 00514 if (pic_idx >= (sizeof(hito_speed_3) / sizeof(graphics_image_t *))) { 00515 pic_idx = 0; 00516 } 00517 wk_wait = 0; 00518 break; 00519 default: 00520 break; 00521 } 00522 } else { 00523 wk_wait--; 00524 } 00525 00526 disp_info.now_pos += disp_info.speed; 00527 if (disp_info.now_pos > GOAL_POINT) { 00528 disp_info.now_pos = GOAL_POINT; 00529 } 00530 total_move_x -= (disp_info.speed - bonus); 00531 } 00532 00533 static void button_fall(void) { 00534 if ((language + 1) < LANGUAGE_NUM) { 00535 language++; 00536 } else { 00537 language = 0; 00538 } 00539 } 00540 00541 int main(void) { 00542 errnum_t err; 00543 Canvas2D_ContextConfigClass config; 00544 00545 /* Change the baud rate of the printf() */ 00546 pc.baud(921600); 00547 00548 button.fall(&button_fall); 00549 00550 /* Initialization of LCD */ 00551 Init_LCD_Display(); 00552 00553 memset(user_frame_buffer1, 0, sizeof(user_frame_buffer1)); 00554 memset(user_frame_buffer2, 0, sizeof(user_frame_buffer2)); 00555 frame_buffer_info.buffer_address[0] = user_frame_buffer1; 00556 frame_buffer_info.buffer_address[1] = user_frame_buffer2; 00557 frame_buffer_info.buffer_count = 2; 00558 frame_buffer_info.show_buffer_index = 0; 00559 frame_buffer_info.draw_buffer_index = 0; 00560 frame_buffer_info.width = LCD_PIXEL_WIDTH; 00561 frame_buffer_info.byte_per_pixel = FRAME_BUFFER_BYTE_PER_PIXEL; 00562 frame_buffer_info.stride = LCD_PIXEL_WIDTH * FRAME_BUFFER_BYTE_PER_PIXEL; 00563 frame_buffer_info.height = LCD_PIXEL_HEIGHT; 00564 frame_buffer_info.pixel_format = PIXEL_FORMAT_RGB565; 00565 00566 config.frame_buffer = &frame_buffer_info; 00567 canvas2d = R_RGA_New_Canvas2D_ContextClass(config); 00568 err = R_OSPL_GetErrNum(); 00569 if (err != 0) { 00570 printf("Line %d, error %d\n", __LINE__, err); 00571 mbed_die(); 00572 } 00573 00574 /* Start of LCD */ 00575 Start_LCD_Display(frame_buffer_info.buffer_address[0]); 00576 00577 /* Backlight on */ 00578 Thread::wait(200); 00579 lcd_cntrst.write(1.0); 00580 00581 Thread touchTask(touch_task, NULL, osPriorityNormal, 1024 * 2); 00582 Timer system_timer; 00583 00584 disp_info.tutorial = true; 00585 disp_info.record_time = 15000; 00586 stop_watch.reset(); 00587 system_timer.reset(); 00588 00589 while (1) { 00590 if (disp_info.tutorial) { 00591 touch_start = false; 00592 stop_watch.reset(); 00593 stop_watch.stop(); 00594 disp_info.speed = 0; 00595 disp_info.ready_set_go = NO_IMAGE; 00596 disp_info.now_pos = 0; 00597 disp_info.comment_no = NO_IMAGE; 00598 disp_info.p_hito = start_File; 00599 disp_info.hito_ofs = 0; 00600 disp_info.max_speed = 0; 00601 disp_info.swipe_on = false; 00602 disp_info.goal_on = false; 00603 disp_info.result_on = false; 00604 disp_info.new_record = false; 00605 disp_info.hosi = false; 00606 disp_info.first_record = true; 00607 disp_info.time_and_speed = false; 00608 disp_info.total_time = 0; 00609 disp_info.swipe_on = true; 00610 disp_info.tutorial_seq = 0; 00611 total_move_x = 0; 00612 race_start = false; 00613 pic_idx = 0; 00614 wk_wait = 0; 00615 tukare = 0; 00616 touch_click = false; 00617 while (touch_click == false) { 00618 display_func(&frame_buffer_info); 00619 } 00620 disp_info.swipe_on = false; 00621 disp_info.tutorial = false; 00622 00623 /* Ready Go! */ 00624 system_timer.reset(); 00625 system_timer.start(); 00626 disp_info.time_and_speed = true; 00627 disp_info.first_record = false; 00628 disp_info.ready_set_go = 3; 00629 disp_info.now_pos = 0; 00630 disp_info.speed = 0; 00631 total_move_x = 0; 00632 pic_idx = 0; 00633 table_no = 0; 00634 } 00635 00636 if (disp_info.ready_set_go != NO_IMAGE) { 00637 int wk_system_time = system_timer.read_ms(); 00638 if (wk_system_time > 4000) { 00639 disp_info.ready_set_go = NO_IMAGE; 00640 if (disp_info.now_pos == 0) { 00641 disp_info.swipe_on = true; 00642 disp_info.tutorial_seq = 0; 00643 } 00644 } else if (wk_system_time > 3000) { 00645 if (disp_info.ready_set_go != 0) { 00646 disp_info.ready_set_go = 0; 00647 touch_start = true; 00648 race_start = true; 00649 stop_watch.start(); 00650 disp_info.comment_no = 0; 00651 } 00652 } else if (wk_system_time > 2000) { 00653 disp_info.ready_set_go = 1; 00654 } else if (wk_system_time > 1000) { 00655 disp_info.ready_set_go = 2; 00656 } else { 00657 /* do nothing */ 00658 } 00659 } 00660 if (disp_info.now_pos != 0) { 00661 disp_info.swipe_on = false; 00662 } 00663 00664 set_hito_pic(); 00665 display_func(&frame_buffer_info); 00666 00667 disp_info.total_time = stop_watch.read_ms(); 00668 if ((disp_info.total_time > 35000) && (disp_info.speed == 0)) { 00669 disp_info.tutorial = true; 00670 } 00671 if (disp_info.now_pos >= GOAL_POINT) { 00672 int over_run = 0; 00673 int wk_ofs; 00674 int back_speed; 00675 int i; 00676 00677 stop_watch.stop(); 00678 race_start = false; 00679 disp_info.comment_no = NO_IMAGE; 00680 00681 /* Through the goal */ 00682 disp_info.goal_on = true; 00683 disp_info.goal_seq = 0; 00684 00685 system_timer.reset(); 00686 system_timer.start(); 00687 00688 while (system_timer.read_ms() < 1500) { 00689 total_move_x = total_move_x * 0.9; 00690 set_hito_pic(); 00691 disp_info.comment_no = NO_IMAGE; 00692 disp_info.hito_ofs -= disp_info.speed; 00693 over_run += disp_info.speed; 00694 display_func(&frame_buffer_info); 00695 if (disp_info.goal_seq < 25) { 00696 disp_info.goal_seq++; 00697 } 00698 } 00699 00700 /* Catch up background */ 00701 disp_info.time_and_speed = false; 00702 back_speed = over_run / 60 + 1; 00703 00704 for (i = 0; i < 60; i++) { 00705 if (over_run > back_speed) { 00706 wk_ofs = back_speed; 00707 if (wk_wait == 0) { 00708 disp_info.p_hito = hito_tukare[pic_idx++]; 00709 if (pic_idx >= (sizeof(hito_tukare) / sizeof(graphics_image_t *))) { 00710 pic_idx = 0; 00711 } 00712 wk_wait = 20; 00713 } else { 00714 wk_wait--; 00715 } 00716 } else { 00717 wk_ofs = over_run; 00718 disp_info.p_hito = goal_File; 00719 } 00720 if (over_run != 0) { 00721 over_run -= wk_ofs; 00722 disp_info.now_pos += wk_ofs; 00723 disp_info.hito_ofs += wk_ofs; 00724 } 00725 display_func(&frame_buffer_info); 00726 if (disp_info.goal_seq > 1) { 00727 disp_info.goal_seq--; 00728 } else { 00729 disp_info.goal_on = false; 00730 } 00731 } 00732 00733 disp_info.result_on = true; 00734 disp_info.first_record = true; 00735 for (disp_info.result_seq = 1; disp_info.result_seq < 40; disp_info.result_seq++) { 00736 display_func(&frame_buffer_info); 00737 } 00738 00739 if (disp_info.total_time < disp_info.record_time) { 00740 disp_info.record_time = disp_info.total_time; 00741 disp_info.new_record = true; 00742 disp_info.hosi = true; 00743 disp_info.hosi_seq = 0; 00744 touch_click = false; 00745 } 00746 00747 touch_click = false; 00748 while (touch_click == false) { 00749 display_func(&frame_buffer_info); 00750 } 00751 disp_info.tutorial = true; 00752 } 00753 } 00754 }
Generated on Wed Jul 13 2022 21:30:27 by
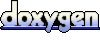