
GR-PEACH Digital Signage sample for DISPLAY SHIELD. USB edition.
Dependencies: GR-PEACH_video GraphicsFramework R_BSP USBHost mbed scan_folder
main.cpp
00001 /* 00002 Permission is hereby granted, free of charge, to any person obtaining a copy 00003 of this software and associated documentation files (the "Software"), to deal 00004 in the Software without restriction, including without limitation the rights 00005 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00006 copies of the Software, and to permit persons to whom the Software is 00007 furnished to do so, subject to the following conditions: 00008 00009 The above copyright notice and this permission notice shall be included in 00010 all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00013 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00014 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00015 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00016 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00018 THE SOFTWARE. 00019 */ 00020 00021 #include "mbed.h" 00022 #include "DisplayBace.h" 00023 #include "RGA.h" 00024 #include "rtos.h" 00025 #include "USBHostMSD.h" 00026 #include "USBHostMouse.h" 00027 #include "BinaryImage_RZ_A1H.h" 00028 #include "scan_folder.h" 00029 00030 #define SVGA (0u) /* 800x600@60 37.9kHz/60Hz */ 00031 #define XGA (1u) /* 1024x768@60 48.4kHz/60Hz */ 00032 #define HD_720p (2u) /* 1280x720@60 45.0kHz/60Hz */ 00033 00034 /**** User Selection *********/ 00035 #define WAIT_TIME (10000) /* wait time (ms) */ 00036 #define DISSOLVE_STEP_NUM (16) /* minimum 1 */ 00037 #define SCROLL_STEP_NUM (8) /* minimum 1 */ 00038 #define SCROLL_DIRECTION (-1) /* Select 1(left to right) or -1(right to left) */ 00039 #define LCD_SIZE HD_720p /* Select SVGA, XGA, or HD_720p */ 00040 /*****************************/ 00041 00042 #if (SCROLL_DIRECTION == -1) 00043 #define SCROLL_DIRECTION_NEXT (-1) 00044 #define SCROLL_DIRECTION_PREV (1) 00045 #else 00046 #define SCROLL_DIRECTION_NEXT (1) 00047 #define SCROLL_DIRECTION_PREV (-1) 00048 #endif 00049 00050 /* LCD Parameter */ 00051 #define LCD_INPUT_CLOCK (66.67) 00052 #if ( LCD_SIZE == SVGA ) 00053 #define LCD_OUTPUT_CLOCK (40.0003) 00054 #define LCD_PIXEL_WIDTH (800u) 00055 #define LCD_PIXEL_HEIGHT (600u) 00056 #define LCD_H_BACK_PORCH (88u) 00057 #define LCD_H_FRONT_PORCH (40u) 00058 #define LCD_H_SYNC_WIDTH (128u) 00059 #define LCD_V_BACK_PORCH (23u) 00060 #define LCD_V_FRONT_PORCH (1u) 00061 #define LCD_V_SYNC_WIDTH (4u) 00062 #elif ( LCD_SIZE == XGA ) 00063 #define LCD_OUTPUT_CLOCK (65.0002) 00064 #define LCD_PIXEL_WIDTH (1024u) 00065 #define LCD_PIXEL_HEIGHT (768u) 00066 #define LCD_H_BACK_PORCH (160u) 00067 #define LCD_H_FRONT_PORCH (24u) 00068 #define LCD_H_SYNC_WIDTH (136u) 00069 #define LCD_V_BACK_PORCH (29u) 00070 #define LCD_V_FRONT_PORCH (3u) 00071 #define LCD_V_SYNC_WIDTH (6u) 00072 #elif ( LCD_SIZE == HD_720p ) 00073 #define LCD_OUTPUT_CLOCK (74.1800) 00074 #define LCD_PIXEL_WIDTH (1280u) 00075 #define LCD_PIXEL_HEIGHT (720u) 00076 #define LCD_H_BACK_PORCH (220u) 00077 #define LCD_H_FRONT_PORCH (70u) 00078 #define LCD_H_SYNC_WIDTH (80u) 00079 #define LCD_V_BACK_PORCH (20u) 00080 #define LCD_V_FRONT_PORCH (5u) 00081 #define LCD_V_SYNC_WIDTH (5u) 00082 #endif 00083 00084 /* FRAME BUFFER Parameter */ 00085 #define FRAME_BUFFER_BYTE_PER_PIXEL (2) 00086 #define FRAME_BUFFER_STRIDE (((LCD_PIXEL_WIDTH * FRAME_BUFFER_BYTE_PER_PIXEL) + 31u) & ~31u) 00087 00088 #define EVENT_NONE (0) 00089 #define EVENT_NEXT (1) 00090 #define EVENT_PREV (2) 00091 00092 #define MAX_JPEG_SIZE (1024 * 450) 00093 #define MOUNT_NAME "usb" 00094 static const char_t * extension_tbl[] = { 00095 ".jpg", 00096 NULL 00097 }; 00098 DigitalIn button(USER_BUTTON0); 00099 I2C i2c(I2C_SDA, I2C_SCL); 00100 DisplayBase Display; 00101 Canvas2D_ContextClass canvas2d; 00102 00103 #if defined(__ICCARM__) 00104 #pragma data_alignment=32 00105 static uint8_t user_frame_buffer[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00106 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00107 #pragma data_alignment=8 00108 static uint8_t JpegBuffer[MAX_JPEG_SIZE]@ ".mirrorram"; //8 bytes aligned!; 00109 #pragma data_alignment=4 00110 #else 00111 static uint8_t user_frame_buffer[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); /* 32 bytes aligned */ 00112 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); /* 32 bytes aligned */ 00113 static uint8_t JpegBuffer[MAX_JPEG_SIZE]__attribute((section("NC_BSS"),aligned(8))); //8 bytes aligned!; 00114 #endif 00115 static frame_buffer_t frame_buffer_info; 00116 static volatile int32_t vsync_count = 0; 00117 static ScanFolder scan_foler; 00118 static int mouse_pos_x = 0; 00119 static int mouse_pos_y = 0; 00120 static bool mouse_pos = false; 00121 static bool mouse_view = false; 00122 static bool mouse_disp_time = false; 00123 static int event_req = EVENT_NONE; 00124 static int disp_time = WAIT_TIME; 00125 00126 static void IntCallbackFunc_Vsync(DisplayBase::int_type_t int_type) { 00127 /* Interrupt callback function for Vsync interruption */ 00128 if (vsync_count > 0) { 00129 vsync_count--; 00130 } 00131 } 00132 00133 static void Wait_Vsync(const int32_t wait_count) { 00134 /* Wait for the specified number of times Vsync occurs */ 00135 vsync_count = wait_count; 00136 while (vsync_count > 0) { 00137 /* Do nothing */ 00138 } 00139 } 00140 00141 static void Init_LCD_Display(uint8_t* disp_buf) { 00142 /* Create DisplayBase object */ 00143 DisplayBase::graphics_error_t error; 00144 DisplayBase::rect_t rect; 00145 DisplayBase::lcd_config_t lcd_config; 00146 PinName lcd_pin[28] = { 00147 /* data pin */ 00148 P11_15, P11_14, P11_13, P11_12, P5_7, P5_6, P5_5, P5_4, P5_3, P5_2, P5_1, P5_0, 00149 P4_7, P4_6, P4_5, P4_4, P10_12, P10_13, P10_14, P10_15, P3_15, P3_14, P3_13, 00150 P3_12, P3_11, P3_10, P3_9, P3_8 00151 }; 00152 00153 Thread::wait(100); 00154 00155 lcd_config.lcd_type = DisplayBase::LCD_TYPE_PARALLEL_RGB; 00156 lcd_config.intputClock = LCD_INPUT_CLOCK; 00157 lcd_config.outputClock = LCD_OUTPUT_CLOCK; 00158 lcd_config.lcd_outformat = DisplayBase::LCD_OUTFORMAT_RGB888; 00159 lcd_config.lcd_edge = DisplayBase::EDGE_RISING; 00160 lcd_config.h_toatal_period = (LCD_PIXEL_WIDTH + LCD_H_FRONT_PORCH + LCD_H_BACK_PORCH + LCD_H_SYNC_WIDTH); 00161 lcd_config.v_toatal_period = (LCD_PIXEL_HEIGHT + LCD_V_FRONT_PORCH + LCD_V_BACK_PORCH + LCD_V_SYNC_WIDTH); 00162 00163 lcd_config.h_disp_widht = LCD_PIXEL_WIDTH; 00164 lcd_config.v_disp_widht = LCD_PIXEL_HEIGHT; 00165 lcd_config.h_back_porch = LCD_H_BACK_PORCH; 00166 lcd_config.v_back_porch = LCD_V_BACK_PORCH; 00167 00168 lcd_config.h_sync_port = DisplayBase::LCD_TCON_PIN_1; 00169 lcd_config.h_sync_port_polarity = DisplayBase::SIG_POL_NOT_INVERTED; 00170 lcd_config.h_sync_width = LCD_H_SYNC_WIDTH; 00171 00172 lcd_config.v_sync_port = DisplayBase::LCD_TCON_PIN_2; 00173 lcd_config.v_sync_port_polarity = DisplayBase::SIG_POL_NOT_INVERTED; 00174 lcd_config.v_sync_width = LCD_V_SYNC_WIDTH; 00175 00176 lcd_config.de_port = DisplayBase::LCD_TCON_PIN_0; 00177 lcd_config.de_port_polarity = DisplayBase::SIG_POL_NOT_INVERTED; 00178 00179 /* Graphics initialization process */ 00180 error = Display.Graphics_init(&lcd_config); 00181 if (error != DisplayBase::GRAPHICS_OK) { 00182 printf("Line %d, error %d\n", __LINE__, error); 00183 while (1); 00184 } 00185 00186 /* Interrupt callback function setting (Vsync signal output from scaler 0) */ 00187 error = Display.Graphics_Irq_Handler_Set(DisplayBase::INT_TYPE_S0_LO_VSYNC, 0, IntCallbackFunc_Vsync); 00188 if (error != DisplayBase::GRAPHICS_OK) { 00189 printf("Line %d, error %d\n", __LINE__, error); 00190 while (1); 00191 } 00192 00193 Display.Graphics_Lcd_Port_Init(lcd_pin, 28); 00194 rect.vs = 0; 00195 rect.vw = LCD_PIXEL_HEIGHT; 00196 rect.hs = 0; 00197 rect.hw = LCD_PIXEL_WIDTH; 00198 00199 Display.Graphics_Read_Setting( 00200 DisplayBase::GRAPHICS_LAYER_0, 00201 (void *)disp_buf, 00202 FRAME_BUFFER_STRIDE, 00203 DisplayBase::GRAPHICS_FORMAT_RGB565, 00204 DisplayBase::WR_RD_WRSWA_32_16BIT, 00205 &rect 00206 ); 00207 } 00208 00209 static void Start_LCD_Display(void) { 00210 Display.Graphics_Start(DisplayBase::GRAPHICS_LAYER_0); 00211 } 00212 00213 static void Update_LCD_Display(frame_buffer_t * frmbuf_info) { 00214 Display.Graphics_Read_Change(DisplayBase::GRAPHICS_LAYER_0, 00215 (void *)frmbuf_info->buffer_address[frmbuf_info->draw_buffer_index]); 00216 Wait_Vsync(1); 00217 } 00218 00219 static void Swap_FrameBuffer(frame_buffer_t * frmbuf_info) { 00220 if (frmbuf_info->draw_buffer_index == 1) { 00221 frmbuf_info->draw_buffer_index = 0; 00222 } else { 00223 frmbuf_info->draw_buffer_index = 1; 00224 } 00225 } 00226 00227 static void draw_mouse_pos(void) { 00228 /* mouse pos */ 00229 if (mouse_pos != false) { 00230 mouse_view = true; 00231 /* Complete drawing */ 00232 R_GRAPHICS_Finish(canvas2d.c_LanguageContext); 00233 /* Draw a image */ 00234 canvas2d.drawImage(mousu_pos_File, mouse_pos_x, mouse_pos_y); 00235 R_OSPL_CLEAR_ERROR(); 00236 00237 if (mouse_disp_time != false) { 00238 const graphics_image_t * p_num; 00239 00240 /* Draw a image */ 00241 canvas2d.drawImage(disp_xsec_File, mouse_pos_x + 30, mouse_pos_y); 00242 R_OSPL_CLEAR_ERROR(); 00243 switch (disp_time) { 00244 case 0: p_num = number0_File; break; 00245 case 1000: p_num = number1_File; break; 00246 case 2000: p_num = number2_File; break; 00247 case 3000: p_num = number3_File; break; 00248 case 4000: p_num = number4_File; break; 00249 case 5000: p_num = number5_File; break; 00250 case 6000: p_num = number6_File; break; 00251 case 7000: p_num = number7_File; break; 00252 case 8000: p_num = number8_File; break; 00253 case 9000: p_num = number9_File; break; 00254 case 10000: p_num = number10_File; break; 00255 case 11000: p_num = number11_File; break; 00256 case 12000: p_num = number12_File; break; 00257 case 13000: p_num = number13_File; break; 00258 case 14000: p_num = number14_File; break; 00259 case 15000: p_num = number15_File; break; 00260 default: p_num = NULL; break; 00261 } 00262 if (p_num != NULL) { 00263 /* Draw a image */ 00264 canvas2d.drawImage(p_num, mouse_pos_x + 136, mouse_pos_y); 00265 R_OSPL_CLEAR_ERROR(); 00266 } 00267 } 00268 } 00269 } 00270 00271 static void draw_image(frame_buffer_t* frmbuf_info, const graphics_image_t* image_new) { 00272 Swap_FrameBuffer(frmbuf_info); 00273 /* Clear */ 00274 canvas2d.clearRect(0, 0, frmbuf_info->width, frmbuf_info->height); 00275 /* Draw a image */ 00276 canvas2d.globalAlpha = 1.0f; 00277 canvas2d.drawImage((const graphics_image_t*)image_new, 0, 0, frmbuf_info->width, frmbuf_info->height); 00278 R_OSPL_CLEAR_ERROR(); 00279 /* mouse pos */ 00280 draw_mouse_pos(); 00281 /* Complete drawing */ 00282 R_GRAPHICS_Finish(canvas2d.c_LanguageContext); 00283 Update_LCD_Display(frmbuf_info); 00284 } 00285 00286 static void draw_image_scroll(frame_buffer_t* frmbuf_info, const graphics_image_t* image_last, 00287 const graphics_image_t* image_new, float32_t scroll, int ditection) { 00288 Swap_FrameBuffer(frmbuf_info); 00289 /* Clear */ 00290 canvas2d.clearRect(0, 0, frmbuf_info->width, frmbuf_info->height); 00291 /* Draw a image */ 00292 canvas2d.globalAlpha = 1.0f; 00293 canvas2d.drawImage((const graphics_image_t*)image_last, 00294 (int_t)(frmbuf_info->width * scroll) * ditection, 0, 00295 frmbuf_info->width, frmbuf_info->height); 00296 R_OSPL_CLEAR_ERROR(); 00297 canvas2d.globalAlpha = 1.0f; 00298 canvas2d.drawImage((const graphics_image_t*)image_new, 00299 ((int_t)(frmbuf_info->width * scroll) - frmbuf_info->width) * ditection, 0, 00300 frmbuf_info->width, frmbuf_info->height); 00301 R_OSPL_CLEAR_ERROR(); 00302 /* mouse pos */ 00303 draw_mouse_pos(); 00304 /* Complete drawing */ 00305 R_GRAPHICS_Finish(canvas2d.c_LanguageContext); 00306 Update_LCD_Display(frmbuf_info); 00307 } 00308 00309 static void draw_image_dissolve(frame_buffer_t* frmbuf_info, const graphics_image_t* image_last, 00310 const graphics_image_t* image_new, float32_t alpha) { 00311 Swap_FrameBuffer(frmbuf_info); 00312 /* Clear */ 00313 canvas2d.clearRect(0, 0, frmbuf_info->width, frmbuf_info->height); 00314 /* Draw a image */ 00315 canvas2d.globalAlpha = 1.0f - alpha; 00316 canvas2d.drawImage((const graphics_image_t*)image_last, 0, 0, frmbuf_info->width, frmbuf_info->height); 00317 R_OSPL_CLEAR_ERROR(); 00318 canvas2d.globalAlpha = alpha; 00319 canvas2d.drawImage((const graphics_image_t*)image_new, 0, 0, frmbuf_info->width, frmbuf_info->height); 00320 R_OSPL_CLEAR_ERROR(); 00321 /* mouse pos */ 00322 draw_mouse_pos(); 00323 /* Complete drawing */ 00324 R_GRAPHICS_Finish(canvas2d.c_LanguageContext); 00325 Update_LCD_Display(frmbuf_info); 00326 } 00327 00328 static void next_file(uint32_t * file_id, uint32_t total_track) { 00329 if (file_id == NULL) { 00330 // do nothing 00331 } else if (*file_id < (total_track - 1)) { 00332 *file_id += 1; 00333 } else { 00334 *file_id = 0; 00335 } 00336 } 00337 00338 static void prev_file(uint32_t * file_id, uint32_t total_track) { 00339 if (file_id == NULL) { 00340 // do nothing 00341 } else if (*file_id != 0) { 00342 *file_id -= 1; 00343 } else { 00344 *file_id = total_track - 1; 00345 } 00346 } 00347 00348 void onMouseEvent(uint8_t buttons, int8_t x, int8_t y, int8_t z) { 00349 int wk_pos; 00350 int wk_disp_time; 00351 static uint8_t last_buttons = 0; 00352 00353 // printf("buttons: %d, x: %d, y: %d, z: %d\r\n", buttons, x, y, z); 00354 00355 wk_pos = mouse_pos_x; 00356 wk_pos += x; 00357 if (wk_pos < 0) { 00358 wk_pos = 0; 00359 } 00360 if (wk_pos > (LCD_PIXEL_WIDTH - 10)) { 00361 wk_pos = LCD_PIXEL_WIDTH - 10; 00362 } 00363 mouse_pos_x = wk_pos; 00364 00365 wk_pos = mouse_pos_y; 00366 wk_pos += y; 00367 if (wk_pos < 0) { 00368 wk_pos = 0; 00369 } 00370 if (wk_pos > (LCD_PIXEL_HEIGHT - 10)) { 00371 wk_pos = (LCD_PIXEL_HEIGHT - 10); 00372 } 00373 mouse_pos_y = wk_pos; 00374 00375 // left 00376 if (((buttons & 0x01) == 0) && ((last_buttons & 0x01) != 0)) { 00377 if (mouse_disp_time != false) { 00378 mouse_disp_time = false; 00379 } else { 00380 event_req = EVENT_NEXT; 00381 } 00382 } 00383 00384 // rigth 00385 if (((buttons & 0x02) == 0) && ((last_buttons & 0x02) != 0)) { 00386 if (mouse_disp_time != false) { 00387 mouse_disp_time = false; 00388 } else { 00389 event_req = EVENT_PREV; 00390 } 00391 } 00392 00393 if (((buttons & 0x04) != 0) && ((last_buttons & 0x04) == 0)) { 00394 mouse_pos = !mouse_pos; 00395 if (mouse_pos == false) { 00396 mouse_disp_time = false; 00397 } 00398 } 00399 00400 if (z != 0) { 00401 if (mouse_pos != false) { 00402 wk_disp_time = disp_time; 00403 if (z > 0) { 00404 wk_disp_time += 1000; 00405 if (wk_disp_time > 15000) { 00406 wk_disp_time = 15000; 00407 } 00408 } else { 00409 wk_disp_time -= 1000; 00410 if (wk_disp_time < 0) { 00411 wk_disp_time = 0; 00412 } 00413 } 00414 disp_time = wk_disp_time; 00415 mouse_disp_time = true; 00416 } 00417 } 00418 00419 last_buttons = buttons; 00420 00421 // printf("pos_x: %d, pos_y: %d\n", mouse_pos_x, mouse_pos_y); 00422 } 00423 00424 void mouse_task(void const *) { 00425 USBHostMouse mouse; 00426 00427 while(1) { 00428 // try to connect a USB mouse 00429 while(!mouse.connect()) 00430 Thread::wait(500); 00431 00432 // when connected, attach handler called on mouse event 00433 mouse.attachEvent(onMouseEvent); 00434 00435 // wait until the mouse is disconnected 00436 while(mouse.connected()) 00437 Thread::wait(500); 00438 } 00439 } 00440 00441 int main(void) { 00442 errnum_t err; 00443 Canvas2D_ContextConfigClass config; 00444 00445 memset(user_frame_buffer, 0, sizeof(user_frame_buffer)); 00446 memset(user_frame_buffer2, 0, sizeof(user_frame_buffer2)); 00447 frame_buffer_info.buffer_address[0] = user_frame_buffer; 00448 frame_buffer_info.buffer_address[1] = user_frame_buffer2; 00449 frame_buffer_info.buffer_count = 2; 00450 frame_buffer_info.show_buffer_index = 0; 00451 frame_buffer_info.draw_buffer_index = 0; 00452 frame_buffer_info.width = LCD_PIXEL_WIDTH; 00453 frame_buffer_info.byte_per_pixel = FRAME_BUFFER_BYTE_PER_PIXEL; 00454 frame_buffer_info.stride = LCD_PIXEL_WIDTH * FRAME_BUFFER_BYTE_PER_PIXEL; 00455 frame_buffer_info.height = LCD_PIXEL_HEIGHT; 00456 frame_buffer_info.pixel_format = PIXEL_FORMAT_RGB565; 00457 Init_LCD_Display(frame_buffer_info.buffer_address[0]); 00458 00459 config.frame_buffer = &frame_buffer_info; 00460 canvas2d = R_RGA_New_Canvas2D_ContextClass(config); 00461 err = R_OSPL_GetErrNum(); 00462 if (err != 0) { 00463 printf("Line %d, error %d\n", __LINE__, err); 00464 while (1); 00465 } 00466 Start_LCD_Display(); 00467 00468 Thread::wait(100); // wait sd mount 00469 Thread mouseTask(mouse_task, NULL, osPriorityNormal, 1024); 00470 00471 USBHostMSD msd(MOUNT_NAME); 00472 uint32_t now_file_id = 0xffffffff; 00473 uint32_t req_file_id = 0; 00474 int jpeg_toggle = 0; 00475 size_t ret; 00476 uint8_t * p_JpegBuffer[2]; 00477 int wait_time = 0; 00478 uint32_t total_file = 0; 00479 00480 p_JpegBuffer[0] = JpegBuffer; 00481 p_JpegBuffer[1] = new uint8_t[MAX_JPEG_SIZE]; 00482 00483 while (1) { 00484 bool key_press = false; 00485 00486 /* USB connect check */ 00487 while (!msd.connected()) { 00488 if (!msd.connect()) { 00489 Thread::wait(500); 00490 } else { 00491 scan_foler.scan("/"MOUNT_NAME, extension_tbl); 00492 total_file = scan_foler.getTotalFile(); 00493 } 00494 } 00495 00496 switch (event_req) { 00497 case EVENT_NEXT: 00498 next_file(&req_file_id, total_file); 00499 key_press = true; 00500 break; 00501 case EVENT_PREV: 00502 prev_file(&req_file_id, total_file); 00503 key_press = true; 00504 break; 00505 case EVENT_NONE: 00506 default: 00507 if (button == 0) { 00508 next_file(&req_file_id, total_file); 00509 key_press = true; 00510 } else if ((wait_time >= disp_time) && (mouse_pos == false)) { 00511 next_file(&req_file_id, total_file); 00512 wait_time = 0; 00513 } else { 00514 // Do Nothing 00515 } 00516 break; 00517 } 00518 event_req = EVENT_NONE; 00519 00520 if (now_file_id != req_file_id) { 00521 FILE * fp = scan_foler.open(req_file_id); 00522 ret = fread(p_JpegBuffer[jpeg_toggle], sizeof(char), MAX_JPEG_SIZE, fp); 00523 scan_foler.close(fp); 00524 if (ret < MAX_JPEG_SIZE) { 00525 if (now_file_id == 0xffffffff) { 00526 // Effect NONE 00527 draw_image(&frame_buffer_info, (const graphics_image_t*)p_JpegBuffer[jpeg_toggle]); 00528 } else if (key_press == false) { 00529 // Effect DISSOLVE 00530 for (int i = 1; i <= DISSOLVE_STEP_NUM; i++) { 00531 draw_image_dissolve(&frame_buffer_info, 00532 (const graphics_image_t*)p_JpegBuffer[!jpeg_toggle], 00533 (const graphics_image_t*)p_JpegBuffer[jpeg_toggle], 00534 (float32_t)i / (float32_t)DISSOLVE_STEP_NUM); 00535 } 00536 } else { 00537 // Effect SCROLL 00538 int direction; 00539 if ((req_file_id == 0) && (now_file_id == (total_file - 1))) { 00540 direction = SCROLL_DIRECTION_NEXT; 00541 } else if ((now_file_id == 0) && (req_file_id == (total_file - 1))) { 00542 direction = SCROLL_DIRECTION_PREV; 00543 } else if (req_file_id > now_file_id) { 00544 direction = SCROLL_DIRECTION_NEXT; 00545 } else { 00546 direction = SCROLL_DIRECTION_PREV; 00547 } 00548 for (int i = 1; i <= SCROLL_STEP_NUM; i++) { 00549 draw_image_scroll(&frame_buffer_info, 00550 (const graphics_image_t*)p_JpegBuffer[!jpeg_toggle], 00551 (const graphics_image_t*)p_JpegBuffer[jpeg_toggle], 00552 (float32_t)i / (float32_t)SCROLL_STEP_NUM, direction); 00553 } 00554 } 00555 jpeg_toggle = !jpeg_toggle; 00556 } 00557 now_file_id = req_file_id; 00558 wait_time = 0; 00559 } else { 00560 if ((mouse_pos != false) || (mouse_view != false)) { 00561 mouse_view = false; 00562 draw_image(&frame_buffer_info, (const graphics_image_t*)p_JpegBuffer[!jpeg_toggle]); 00563 wait_time = 0; 00564 } else { 00565 Thread::wait(100); 00566 wait_time += 100; 00567 } 00568 } 00569 } 00570 }
Generated on Thu Jul 28 2022 16:26:36 by
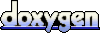