
BLE Application to open a Garage door
Dependencies: BLE_API Crypto RNG mbed nRF51822
Fork of BLE_LED by
SecurityService.h
00001 #ifndef __BLE_SECURITY_SERVICE_H__ 00002 #define __BLE_SECURITY_SERVICE_H__ 00003 00004 #include "Crypto.h" 00005 #include "Random.h" 00006 00007 class SecurityService { 00008 public: 00009 const static uint16_t SECURITY_SERVICE_UUID = 0x3000; 00010 const static uint16_t SECURITY_IV_CHARACTERISTIC_UUID = 0x3001; 00011 const static uint16_t SECURITY_KEY_CHARACTERISTIC_UUID = 0x3002; 00012 00013 SecurityService(BLE &_ble) : 00014 ble(_ble), 00015 SecurityIV(SECURITY_IV_CHARACTERISTIC_UUID, (uint8_t *) "IV"), 00016 SecurityKey(SECURITY_KEY_CHARACTERISTIC_UUID, (uint8_t *) "KEY") 00017 { 00018 GattCharacteristic *charTable[] = {&SecurityIV, &SecurityKey}; 00019 00020 GattService SecurityService(SECURITY_SERVICE_UUID, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00021 ble.gattServer().addService(SecurityService); 00022 } 00023 void init(char *shared_key) { 00024 //Initialize AES 00025 uint8_t new_iv[16] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; 00026 memcpy(iv, new_iv,16); 00027 ble.gattServer().write(SecurityIV.getValueHandle(), (uint8_t *)iv, 16*sizeof(uint8_t)); 00028 setKey(shared_key); 00029 } 00030 00031 void decode(uint8_t *out, uint8_t *in, uint32_t len) { 00032 AES myAES(AES_128, myKey, iv); 00033 myAES.decrypt(out,in,len); 00034 genIV(); 00035 } 00036 00037 void setKey(char *shared_key) { 00038 DBG("Set shared_key = %s\r\n", shared_key); 00039 MD5::computeHash(myKey, (uint8_t*) shared_key, strlen(shared_key)); 00040 } 00041 00042 void genIV() { 00043 Random rnd; 00044 rnd.init(); 00045 rnd.getBytes(iv, 16); 00046 ble.gattServer().write(SecurityIV.getValueHandle(), (uint8_t *)iv, 16*sizeof(uint8_t)); 00047 } 00048 00049 GattAttribute::Handle_t getKeyHandle() const { 00050 return SecurityKey.getValueHandle(); 00051 } 00052 00053 private: 00054 uint8_t iv[16]; 00055 uint8_t myKey[16]; 00056 00057 BLE &ble; 00058 ReadOnlyArrayGattCharacteristic<uint8_t, 16> SecurityIV; 00059 WriteOnlyArrayGattCharacteristic<uint8_t, 16> SecurityKey; 00060 }; 00061 00062 #endif /* #ifndef __BLE_SECURITY_SERVICE_H__ */
Generated on Thu Jul 14 2022 08:19:32 by
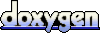