
Port of TI's CC3100 Websock camera demo. Using FreeRTOS, mbedTLS, also parts of Arducam for cams ov5642 and 0v2640. Can also use MT9D111. Work in progress. Be warned some parts maybe a bit flacky. This is for Seeed Arch max only, for an M3, see the demo for CM3 using the 0v5642 aducam mini.
ripemd160.h
00001 /** 00002 * \file ripemd160.h 00003 * 00004 * \brief RIPE MD-160 message digest 00005 * 00006 * Copyright (C) 2014-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_RIPEMD160_H 00025 #define POLARSSL_RIPEMD160_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00036 #include <basetsd.h> 00037 typedef UINT32 uint32_t; 00038 #else 00039 #include <inttypes.h> 00040 #endif 00041 00042 #define POLARSSL_ERR_RIPEMD160_FILE_IO_ERROR -0x007E /**< Read/write error in file. */ 00043 00044 #if !defined(POLARSSL_RIPEMD160_ALT) 00045 // Regular implementation 00046 // 00047 00048 #ifdef __cplusplus 00049 extern "C" { 00050 #endif 00051 00052 /** 00053 * \brief RIPEMD-160 context structure 00054 */ 00055 typedef struct 00056 { 00057 uint32_t total[2]; /*!< number of bytes processed */ 00058 uint32_t state[5]; /*!< intermediate digest state */ 00059 unsigned char buffer[64]; /*!< data block being processed */ 00060 00061 unsigned char ipad[64]; /*!< HMAC: inner padding */ 00062 unsigned char opad[64]; /*!< HMAC: outer padding */ 00063 } 00064 ripemd160_context; 00065 00066 /** 00067 * \brief Initialize RIPEMD-160 context 00068 * 00069 * \param ctx RIPEMD-160 context to be initialized 00070 */ 00071 void ripemd160_init( ripemd160_context *ctx ); 00072 00073 /** 00074 * \brief Clear RIPEMD-160 context 00075 * 00076 * \param ctx RIPEMD-160 context to be cleared 00077 */ 00078 void ripemd160_free( ripemd160_context *ctx ); 00079 00080 /** 00081 * \brief RIPEMD-160 context setup 00082 * 00083 * \param ctx context to be initialized 00084 */ 00085 void ripemd160_starts( ripemd160_context *ctx ); 00086 00087 /** 00088 * \brief RIPEMD-160 process buffer 00089 * 00090 * \param ctx RIPEMD-160 context 00091 * \param input buffer holding the data 00092 * \param ilen length of the input data 00093 */ 00094 void ripemd160_update( ripemd160_context *ctx, 00095 const unsigned char *input, size_t ilen ); 00096 00097 /** 00098 * \brief RIPEMD-160 final digest 00099 * 00100 * \param ctx RIPEMD-160 context 00101 * \param output RIPEMD-160 checksum result 00102 */ 00103 void ripemd160_finish( ripemd160_context *ctx, unsigned char output[20] ); 00104 00105 /* Internal use */ 00106 void ripemd160_process( ripemd160_context *ctx, const unsigned char data[64] ); 00107 00108 #ifdef __cplusplus 00109 } 00110 #endif 00111 00112 #else /* POLARSSL_RIPEMD160_ALT */ 00113 #include "ripemd160.h" 00114 #endif /* POLARSSL_RIPEMD160_ALT */ 00115 00116 #ifdef __cplusplus 00117 extern "C" { 00118 #endif 00119 00120 /** 00121 * \brief Output = RIPEMD-160( input buffer ) 00122 * 00123 * \param input buffer holding the data 00124 * \param ilen length of the input data 00125 * \param output RIPEMD-160 checksum result 00126 */ 00127 void ripemd160( const unsigned char *input, size_t ilen, 00128 unsigned char output[20] ); 00129 00130 #if defined(POLARSSL_FS_IO) 00131 /** 00132 * \brief Output = RIPEMD-160( file contents ) 00133 * 00134 * \param path input file name 00135 * \param output RIPEMD-160 checksum result 00136 * 00137 * \return 0 if successful, or POLARSSL_ERR_RIPEMD160_FILE_IO_ERROR 00138 */ 00139 int ripemd160_file( const char *path, unsigned char output[20] ); 00140 #endif /* POLARSSL_FS_IO */ 00141 00142 /** 00143 * \brief RIPEMD-160 HMAC context setup 00144 * 00145 * \param ctx HMAC context to be initialized 00146 * \param key HMAC secret key 00147 * \param keylen length of the HMAC key 00148 */ 00149 void ripemd160_hmac_starts( ripemd160_context *ctx, 00150 const unsigned char *key, size_t keylen ); 00151 00152 /** 00153 * \brief RIPEMD-160 HMAC process buffer 00154 * 00155 * \param ctx HMAC context 00156 * \param input buffer holding the data 00157 * \param ilen length of the input data 00158 */ 00159 void ripemd160_hmac_update( ripemd160_context *ctx, 00160 const unsigned char *input, size_t ilen ); 00161 00162 /** 00163 * \brief RIPEMD-160 HMAC final digest 00164 * 00165 * \param ctx HMAC context 00166 * \param output RIPEMD-160 HMAC checksum result 00167 */ 00168 void ripemd160_hmac_finish( ripemd160_context *ctx, unsigned char output[20] ); 00169 00170 /** 00171 * \brief RIPEMD-160 HMAC context reset 00172 * 00173 * \param ctx HMAC context to be reset 00174 */ 00175 void ripemd160_hmac_reset( ripemd160_context *ctx ); 00176 00177 /** 00178 * \brief Output = HMAC-RIPEMD-160( hmac key, input buffer ) 00179 * 00180 * \param key HMAC secret key 00181 * \param keylen length of the HMAC key 00182 * \param input buffer holding the data 00183 * \param ilen length of the input data 00184 * \param output HMAC-RIPEMD-160 result 00185 */ 00186 void ripemd160_hmac( const unsigned char *key, size_t keylen, 00187 const unsigned char *input, size_t ilen, 00188 unsigned char output[20] ); 00189 00190 /** 00191 * \brief Checkup routine 00192 * 00193 * \return 0 if successful, or 1 if the test failed 00194 */ 00195 int ripemd160_self_test( int verbose ); 00196 00197 #ifdef __cplusplus 00198 } 00199 #endif 00200 00201 #endif /* ripemd160.h */ 00202
Generated on Tue Jul 12 2022 22:22:38 by
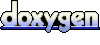